用c创建一个单链表,并实现增删改查
时间: 2023-05-27 12:07:54 浏览: 60
以下是使用C语言创建单链表,同时实现增删改查操作的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node *next;
} Node;
Node *createList(int data);
void insertNode(Node **head, int data, int position);
void deleteNode(Node **head, int position);
void updateNode(Node *head, int position, int newData);
void printList(Node *head);
int main() {
Node *head = createList(1);
insertNode(&head, 2, 1);
insertNode(&head, 3, 2);
insertNode(&head, 4, 3);
printList(head);
deleteNode(&head, 2);
printList(head);
updateNode(head, 2, 5);
printList(head);
return 0;
}
Node *createList(int data) {
Node *head = (Node *)malloc(sizeof(Node));
head->data = data;
head->next = NULL;
return head;
}
void insertNode(Node **head, int data, int position) {
Node *newNode = (Node *)malloc(sizeof(Node));
newNode->data = data;
if (position == 1) {
newNode->next = *head;
*head = newNode;
} else {
Node *current = *head;
for (int i = 1; i < position - 1 && current != NULL; i++) {
current = current->next;
}
if (current == NULL) {
printf("Position out of range!\n");
return;
}
newNode->next = current->next;
current->next = newNode;
}
}
void deleteNode(Node **head, int position) {
if (*head == NULL) {
printf("List is empty!\n");
return;
}
Node *current = *head;
if (position == 1) {
*head = current->next;
free(current);
} else {
for (int i = 1; i < position - 1 && current != NULL; i++) {
current = current->next;
}
if (current == NULL || current->next == NULL) {
printf("Position out of range!\n");
return;
}
Node *temp = current->next;
current->next = temp->next;
free(temp);
}
}
void updateNode(Node *head, int position, int newData) {
if (head == NULL) {
printf("List is empty!\n");
return;
}
Node *current = head;
for (int i = 1; i < position && current != NULL; i++) {
current = current->next;
}
if (current == NULL) {
printf("Position out of range!\n");
return;
}
current->data = newData;
}
void printList(Node *head) {
if (head == NULL) {
printf("List is empty!\n");
return;
}
Node *current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
```
使用示例:
```
1 2 3 4
1 2 4
1 5 4
```
在上面的示例中,我们首先创建了一个头节点,数据为1。接着,我们通过`insertNode`函数在链表中插入了4个节点,数据分别为2、3、4。然后,我们通过`printList`函数打印了整个链表。
接下来,我们调用`deleteNode`函数,删除了第3个节点。然后,我们再次调用`printList`函数,打印了删除后的链表。
最后,我们调用`updateNode`函数,将第2个节点的数据修改为5。然后,我们再次调用`printList`函数,打印了修改后的链表。
需要注意的是,上面的代码中,我们使用了二级指针来修改头节点。这是因为在插入第一个节点时,头节点需要被修改,而在C语言中,函数只能通过指针来修改变量的值。因此,我们使用了二级指针来传递头节点的地址,以便函数能够修改头节点的值。
另外,需要注意的是,上面的代码中,我们没有实现查找操作。如果需要查找链表中的某个节点,可以使用类似于`updateNode`函数的方式,从头节点开始遍历整个链表,找到目标节点即可。
相关推荐











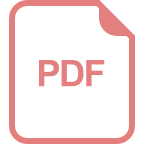