怎么制作一个基于yolox的pyqt5界面,界面包括这些功能:选择权重文件、检测图片、检测视频、保存检测结果
时间: 2023-06-17 12:06:33 浏览: 246
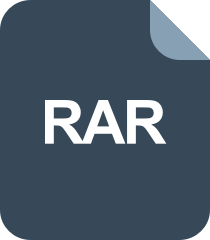
YOLOv5文本表格检测+检测权重+数据集+pyqt界面

制作一个基于yolox的pyqt5界面,需要按照以下步骤进行操作:
1. 安装yolox和pyqt5库
```
pip install yolox
pip install PyQt5
```
2. 创建一个PyQt5界面,并添加选择权重文件、检测图片、检测视频、保存检测结果的按钮和文本框。
```python
import sys
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QPushButton, QFileDialog, QLineEdit
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 选择权重文件
self.btn_select_weight = QPushButton('选择权重文件', self)
self.btn_select_weight.move(20, 20)
self.btn_select_weight.clicked.connect(self.select_weight_file)
# 检测图片
self.btn_detect_image = QPushButton('检测图片', self)
self.btn_detect_image.move(20, 60)
self.btn_detect_image.clicked.connect(self.detect_image)
# 检测视频
self.btn_detect_video = QPushButton('检测视频', self)
self.btn_detect_video.move(20, 100)
self.btn_detect_video.clicked.connect(self.detect_video)
# 保存检测结果
self.btn_save_result = QPushButton('保存检测结果', self)
self.btn_save_result.move(20, 140)
self.btn_save_result.clicked.connect(self.save_result)
# 显示权重文件路径
self.weight_file_path = QLineEdit(self)
self.weight_file_path.move(150, 25)
self.weight_file_path.resize(400, 20)
# 显示检测图片路径
self.image_file_path = QLineEdit(self)
self.image_file_path.move(150, 65)
self.image_file_path.resize(400, 20)
# 显示检测视频路径
self.video_file_path = QLineEdit(self)
self.video_file_path.move(150, 105)
self.video_file_path.resize(400, 20)
# 显示保存结果路径
self.result_file_path = QLineEdit(self)
self.result_file_path.move(150, 145)
self.result_file_path.resize(400, 20)
self.setGeometry(300, 300, 600, 200)
self.setWindowTitle('YOLOX GUI')
self.show()
# 选择权重文件
def select_weight_file(self):
file_name, _ = QFileDialog.getOpenFileName(self, '选择权重文件', '', '(*.pth)')
self.weight_file_path.setText(file_name)
# 检测图片
def detect_image(self):
pass
# 检测视频
def detect_video(self):
pass
# 保存检测结果
def save_result(self):
pass
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MainWindow()
sys.exit(app.exec_())
```
3. 在按钮的回调函数中添加yolox代码,实现选择权重文件、检测图片、检测视频和保存检测结果的功能。
```python
import sys
import torch
import cv2
import numpy as np
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QPushButton, QFileDialog, QLineEdit
from yolox.data.data_augment import preproc as preprocess
from yolox.exp import get_exp
from yolox.utils import postprocess, demo_postprocess
class MainWindow(QWidget):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
# 选择权重文件
self.btn_select_weight = QPushButton('选择权重文件', self)
self.btn_select_weight.move(20, 20)
self.btn_select_weight.clicked.connect(self.select_weight_file)
# 检测图片
self.btn_detect_image = QPushButton('检测图片', self)
self.btn_detect_image.move(20, 60)
self.btn_detect_image.clicked.connect(self.detect_image)
# 检测视频
self.btn_detect_video = QPushButton('检测视频', self)
self.btn_detect_video.move(20, 100)
self.btn_detect_video.clicked.connect(self.detect_video)
# 保存检测结果
self.btn_save_result = QPushButton('保存检测结果', self)
self.btn_save_result.move(20, 140)
self.btn_save_result.clicked.connect(self.save_result)
# 显示权重文件路径
self.weight_file_path = QLineEdit(self)
self.weight_file_path.move(150, 25)
self.weight_file_path.resize(400, 20)
# 显示检测图片路径
self.image_file_path = QLineEdit(self)
self.image_file_path.move(150, 65)
self.image_file_path.resize(400, 20)
# 显示检测视频路径
self.video_file_path = QLineEdit(self)
self.video_file_path.move(150, 105)
self.video_file_path.resize(400, 20)
# 显示保存结果路径
self.result_file_path = QLineEdit(self)
self.result_file_path.move(150, 145)
self.result_file_path.resize(400, 20)
self.setGeometry(300, 300, 600, 200)
self.setWindowTitle('YOLOX GUI')
self.show()
# 选择权重文件
def select_weight_file(self):
file_name, _ = QFileDialog.getOpenFileName(self, '选择权重文件', '', '(*.pth)')
self.weight_file_path.setText(file_name)
# 检测图片
def detect_image(self):
exp = get_exp('yolox-s')
exp.test_conf = 0.01
exp.nms_conf = 0.65
exp.device = 'cpu'
weight_file = self.weight_file_path.text()
image_file = self.image_file_path.text()
model = exp.get_model()
with torch.no_grad():
img = cv2.imread(image_file)
img_info = np.array([img.shape[0], img.shape[1], 1.0], dtype=np.float32)
img = preprocess(img, exp.test_size, exp.mean, exp.std)
img = img.unsqueeze(0)
outputs = model(img)
outputs = postprocess(outputs, exp.test_conf, exp.nms_conf)
outputs = demo_postprocess(outputs, img_info, exp.test_size)
for output in outputs:
print(output['class_id'], output['score'], output['bbox'])
# 检测视频
def detect_video(self):
exp = get_exp('yolox-s')
exp.test_conf = 0.01
exp.nms_conf = 0.65
exp.device = 'cpu'
weight_file = self.weight_file_path.text()
video_file = self.video_file_path.text()
model = exp.get_model()
cap = cv2.VideoCapture(video_file)
while True:
ret, frame = cap.read()
if not ret:
break
with torch.no_grad():
img_info = np.array([frame.shape[0], frame.shape[1], 1.0], dtype=np.float32)
img = preprocess(frame, exp.test_size, exp.mean, exp.std)
img = img.unsqueeze(0)
outputs = model(img)
outputs = postprocess(outputs, exp.test_conf, exp.nms_conf)
outputs = demo_postprocess(outputs, img_info, exp.test_size)
for output in outputs:
print(output['class_id'], output['score'], output['bbox'])
cv2.imshow('frame', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
# 保存检测结果
def save_result(self):
pass
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = MainWindow()
sys.exit(app.exec_())
```
4. 运行程序,选择权重文件、检测图片、检测视频和保存检测结果。每次检测都会在控制台输出检测结果。
阅读全文
相关推荐
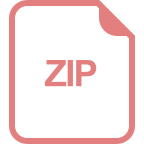
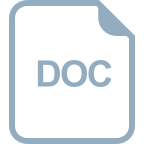
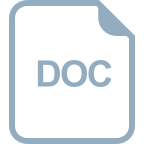
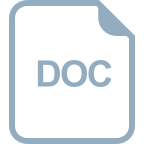
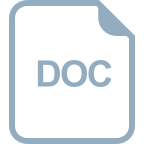

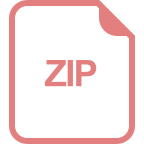
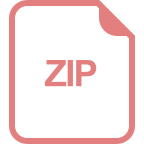
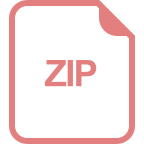
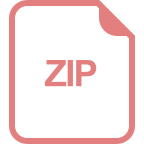
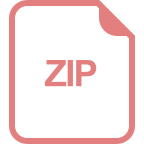
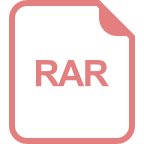
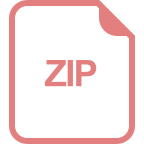
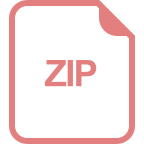
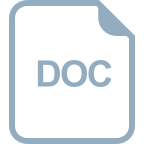
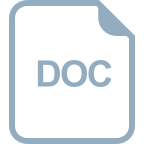
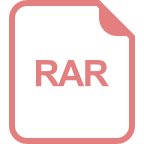
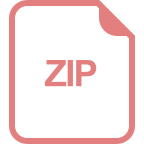