各种导航坐标系之间转换之类的代码用C++语言实现
时间: 2024-02-17 15:03:15 浏览: 26
下面是一个简单的C++代码示例,用于将经纬度坐标系(WGS84)转换为UTM坐标系:
```cpp
#include <iostream>
#include <cmath>
const double PI = 3.14159265358979323846;
const double a = 6378137.0;
const double b = 6356752.314245;
double deg2rad(double deg) {
return deg * PI / 180.0;
}
double rad2deg(double rad) {
return rad * 180.0 / PI;
}
void wgs84_to_utm(double lat, double lon, int &zone, double &easting, double &northing) {
// Convert latitude and longitude to radians
double phi = deg2rad(lat);
double lambda = deg2rad(lon);
// Compute the UTM zone number
zone = static_cast<int>(std::floor((lon + 180.0) / 6.0) + 1);
// Compute the central meridian for the zone
double lambda0 = deg2rad((zone - 1) * 6 - 180 + 3);
// Compute the necessary parameters for the UTM projection
double e = std::sqrt(1.0 - b*b / (a*a));
double ep2 = (a*a - b*b) / (b*b);
double n = a / std::sqrt(1.0 - e*e * std::sin(phi)*std::sin(phi));
double rho = a * (1.0 - e*e) / std::pow(1.0 - e*e * std::sin(phi)*std::sin(phi), 1.5);
double nu = a / std::sqrt(1.0 - e*e * std::sin(phi)*std::sin(phi));
double t = std::tan(phi);
double t2 = t * t;
double l = lambda - lambda0;
double l3 = l * l * l;
// Compute the UTM easting and northing
easting = 500000.0 + (nu / 6.0) * std::pow(cos(phi), 3) * (l*l - t2*l3 + (5 - t2 + 9*ep2)*l3*l*l*l / 24.0);
northing = (rho / 6.0) * cos(phi) * (l3 - t2*l*l + l3*l*l*l*(7 - t2 - 58*ep2) / 24.0);
if (lat < 0.0) {
northing += 10000000.0;
}
}
int main() {
// Convert WGS84 coordinates to UTM coordinates
double lat = 37.7749295;
double lon = -122.4194155;
int zone;
double easting, northing;
wgs84_to_utm(lat, lon, zone, easting, northing);
// Print the results
std::cout << "Zone: " << zone << std::endl;
std::cout << "Easting: " << easting << " meters" << std::endl;
std::cout << "Northing: " << northing << " meters" << std::endl;
return 0;
}
```
这个示例代码将经度为-122.4194155度,纬度为37.7749295度的WGS84坐标系转换为UTM坐标系。
相关推荐
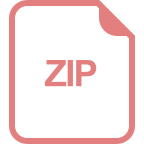
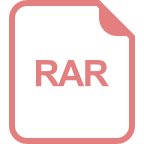
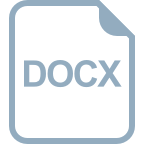














