springboot ServletOutputStream 结合S3TransferManager DownloadFileRequestj进行文件下载
时间: 2024-04-04 15:33:00 浏览: 12
在Spring Boot应用程序中,您可以使用`@RequestMapping`注解来创建一个处理文件下载请求的Controller。您可以在Controller中使用`S3TransferManager`下载文件并将其写入`ServletOutputStream`。下面是一个示例代码片段,演示如何将从Amazon S3下载的文件写入`ServletOutputStream`:
```java
import com.amazonaws.services.s3.transfer.Download;
import com.amazonaws.services.s3.transfer.TransferManager;
import com.amazonaws.services.s3.transfer.TransferManagerBuilder;
import com.amazonaws.services.s3.transfer.model.DownloadResult;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.core.io.InputStreamResource;
import org.springframework.core.io.Resource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.net.URLEncoder;
@Controller
public class FileDownloadController {
@Value("${aws.s3.bucketName}")
private String bucketName;
@Value("${aws.s3.region}")
private String region;
@Value("${aws.s3.accessKeyId}")
private String accessKeyId;
@Value("${aws.s3.secretAccessKey}")
private String secretAccessKey;
@GetMapping("/download/{key}")
public ResponseEntity<Resource> downloadFile(@PathVariable String key, HttpServletResponse response) throws IOException {
TransferManager transferManager = TransferManagerBuilder.standard()
.withS3Client(AmazonS3ClientBuilder.standard()
.withRegion(region)
.withCredentials(new AWSStaticCredentialsProvider(new BasicAWSCredentials(accessKeyId, secretAccessKey)))
.build())
.build();
File file = new File(key);
Download download = transferManager.download(bucketName, key, file);
InputStream inputStream = new FileInputStream(file);
InputStreamResource resource = new InputStreamResource(inputStream);
HttpHeaders headers = new HttpHeaders();
headers.add(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + URLEncoder.encode(key, "UTF-8") + "\"");
headers.add(HttpHeaders.CONTENT_TYPE, MediaType.APPLICATION_OCTET_STREAM_VALUE);
headers.add(HttpHeaders.CONTENT_LENGTH, file.length() + "");
headers.add(HttpHeaders.CACHE_CONTROL, "no-cache, no-store, must-revalidate");
headers.add(HttpHeaders.PRAGMA, "no-cache");
headers.add(HttpHeaders.EXPIRES, "0");
return ResponseEntity.ok()
.headers(headers)
.body(resource);
}
}
```
在上面的代码中,`@GetMapping("/download/{key}")`用于创建一个处理文件下载请求的Controller方法。`TransferManager`用于下载文件,其中`bucketName`是存储桶名称,`region`是存储桶所在的区域,`accessKeyId`和`secretAccessKey`是AWS的访问密钥。
在下载完成后,将文件内容写入`HttpServletResponse`中,通过设置`Content-Disposition`响应头,指定浏览器以附件形式下载文件。最后,将文件内容写入`InputStreamResource`并返回`ResponseEntity`对象。
相关推荐
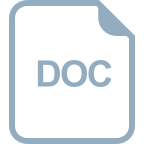
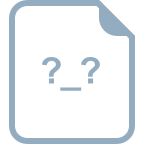














