单链表的增删查改的c语言代码实现
时间: 2023-06-04 07:04:45 浏览: 80
可以的,以下是单链表的增删查改的c语言代码实现:
单链表的定义:
```
typedef struct Node{
int data; //存放链表的数据
struct Node *next; //指向下一个链表节点的指针
}*LinkedList, Node;
```
链表节点的插入:
```
LinkedList LinkedListInsert(LinkedList head, int data, int index)
{
Node* node = (Node*)malloc(sizeof(Node));
node->data = data;
node->next = NULL;
Node* p = head;
int count = 0;
while (p->next != NULL && count < index)
{
p = p->next;
count++;
}
if (count == index)
{
node->next = p->next;
p->next = node;
}
return head;
}
```
链表节点的删除:
```
LinkedList LinkedListDelete(LinkedList head, int index)
{
Node* p = head;
Node* temp;
int count = 0;
while (p->next != NULL && count < index)
{
p = p->next;
count++;
}
if (count == index)
{
temp = p->next;
p->next = temp->next;
free(temp);
}
return head;
}
```
链表节点的查找:
```
int LinkedListSearch(LinkedList head, int data)
{
Node* p = head;
int index = 0;
while (p->next != NULL)
{
if (p->data == data)
{
return index;
}
p = p->next;
index++;
}
return -1;
}
```
链表节点的修改:
```
LinkedList LinkedListModify(LinkedList head, int data, int index)
{
Node* p = head;
int count = 0;
while (p->next != NULL && count < index)
{
p = p->next;
count++;
}
if (count == index)
{
p->data = data;
}
return head;
}
```
相关推荐
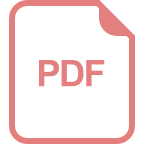














