#include<cstring> using namespace std; int main() { char str1[7]="country",str2[7]="morning", temp; if(str1>str2) { temp=strl; str1=str2; str2=temp; } cout << str1 << " " << str2; return 0; }改错
时间: 2023-06-24 09:09:29 浏览: 40
这段代码中有几个错误:
1. `strl` 应该是 `str1`。
2. `str1` 和 `str2` 都是字符数组,不能直接使用大于号进行比较。
3. `str1` 和 `str2` 是数组名,不能被赋值。
4. `temp` 定义的类型应该是 `char` 而不是 `char[]`。
修改后的代码如下:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str1[7] = "country", str2[7] = "morning", temp;
if(strcmp(str1, str2) > 0) {
temp = str1[0];
strcpy(str1, str2);
str2[0] = temp;
}
cout << str1 << " " << str2;
return 0;
}
```
修改后的代码中,使用 `strcmp()` 函数比较两个字符串的大小,使用 `strcpy()` 函数将 `str2` 的内容复制到 `str1` 中,使用 `temp` 存储 `str1` 的首字符,将 `temp` 赋值给 `str2` 的首字符。
相关问题
#include<iostream> #include<cstring> using namespace std; int main() { char str1[7] = "country",str2[7]="morning", temp; if(strcmp(str1>str2)>0 { temp = str1[0] strcpy(str1,str2); str2[0] = temp; } cout << str1 << " " << str2; return 0; }
这段代码中存在语法错误和逻辑错误,下面是修改后的代码:
```c++
#include<iostream>
#include<cstring>
using namespace std;
int main() {
char str1[8] = "country", str2[8] = "morning", temp; // 字符串数组需要多分配一个字符用于存放'\0'
if(strcmp(str1, str2) > 0) { // strcmp 函数的使用有误
temp = str1[0];
strcpy(str1, str2);
str2[0] = temp;
}
cout << str1 << " " << str2;
return 0;
}
```
修改后的代码中,我们将字符串数组 `str1` 和 `str2` 分别初始化为 "country" 和 "morning"。在比较字符串大小时,我们使用了 `strcmp` 函数,该函数的返回值为整数,当第一个参数大于第二个参数时返回正整数,小于时返回负整数,等于时返回 0,因此我们需要将其与 0 进行比较。在交换字符串首字符时,我们需要使用一个中间变量 `temp` 存储 `str1[0]` 的值,然后将 `str1` 赋值为 `str2`,再将 `str2[0]` 赋值为 `temp`。最后输出结果即可。
请帮我逐句解释下列代码: #include<stdio.h> //f(n)=g(n)+h(n) #include<iostream> #include<cstring> #include<queue> #include<set> using namespace std;
这段代码是一个C++程序,包含了一些头文件的引用和命名空间的声明。下面是对代码的逐句解释:
1. `#include<stdio.h>`:引用了C标准库中的stdio.h头文件,该头文件包含了一些输入输出函数的声明,比如printf和scanf等。
2. `#include<iostream>`:引用了C++标准库中的iostream头文件,该头文件包含了一些输入输出流对象的声明,比如cin和cout等。
3. `#include<cstring>`:引用了C++标准库中的cstring头文件,该头文件包含了一些字符串处理函数的声明,比如strlen和strcpy等。
4. `#include<queue>`:引用了C++标准库中的queue头文件,该头文件包含了队列容器的声明和相关操作函数的定义。
5. `#include<set>`:引用了C++标准库中的set头文件,该头文件包含了集合容器的声明和相关操作函数的定义。
6. `using namespace std;`:使用了std命名空间,这样就可以直接使用std命名空间下的函数和对象,而不需要加上命名空间前缀。
总结起来,这段代码主要是引用了一些标准库的头文件,并使用了std命名空间。
相关推荐
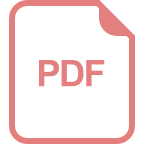
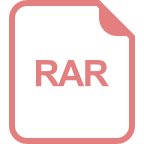













