如何使用 RenderScript 使 AlertDialog 实现高斯模糊
时间: 2023-07-20 18:11:24 浏览: 238
实现 AlertDialog 高斯模糊的方法与一般的 View 高斯模糊基本相同,只需要在 AlertDialog 的背景中添加高斯模糊效果即可。下面是使用 RenderScript 实现 AlertDialog 高斯模糊的示例代码:
1. 在 res/values/styles.xml 文件中定义一个 MyAlertDialogTheme 主题,用于设置 AlertDialog 的样式:
```
<style name="MyAlertDialogTheme" parent="Theme.AppCompat.Light.Dialog.Alert">
<item name="android:windowBackground">@drawable/dialog_bg_blur</item>
</style>
```
2. 在 res/drawable 文件夹中创建一个 dialog_bg_blur.xml 文件,用于设置 AlertDialog 背景:
```
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<!-- 设置底层背景 -->
<item android:id="@android:id/background">
<shape android:shape="rectangle">
<solid android:color="@android:color/transparent" />
</shape>
</item>
<!-- 设置高斯模糊层 -->
<item android:id="@+id/dialog_blur_layer">
<bitmap android:src="@drawable/dialog_bg" />
</item>
</layer-list>
```
其中,dialog_bg.xml 是一个不带高斯模糊效果的背景图片。
3. 在 res/drawable-v21 文件夹中创建一个 dialog_bg_blur.xml 文件,用于设置 AlertDialog 背景(仅适用于 Android 5.0 及以上版本):
```
<layer-list xmlns:android="http://schemas.android.com/apk/res/android">
<!-- 设置底层背景 -->
<item android:id="@android:id/background">
<shape android:shape="rectangle">
<solid android:color="@android:color/transparent" />
</shape>
</item>
<!-- 设置高斯模糊层 -->
<item android:id="@+id/dialog_blur_layer">
<bitmap android:src="@drawable/dialog_bg" />
<blur android:radius="25dp" android:scale="1" />
</item>
</layer-list>
```
其中,blur 标签用于设置高斯模糊效果,radius 属性表示模糊半径,scale 属性表示模糊程度。
4. 在 Java 代码中创建一个 Bitmap 对象,用于存储高斯模糊后的图片:
```
private Bitmap getBlurBitmap(Context context, Bitmap bitmap) {
// 创建一个空的 Bitmap 对象
Bitmap blurBitmap = Bitmap.createBitmap(bitmap.getWidth(), bitmap.getHeight(), Bitmap.Config.ARGB_8888);
// 创建一个 RenderScript 对象
RenderScript rs = RenderScript.create(context);
// 创建一个 Allocation 对象,用于存储原始图片数据
Allocation input = Allocation.createFromBitmap(rs, bitmap);
// 创建一个 Allocation 对象,用于存储高斯模糊后的图片数据
Allocation output = Allocation.createFromBitmap(rs, blurBitmap);
// 创建一个 ScriptIntrinsicBlur 对象,用于实现高斯模糊效果
ScriptIntrinsicBlur script = ScriptIntrinsicBlur.create(rs, Element.U8_4(rs));
// 设置高斯模糊半径
script.setRadius(25);
// 执行高斯模糊
script.setInput(input);
script.forEach(output);
// 将高斯模糊后的图片数据保存到 Bitmap 对象中
output.copyTo(blurBitmap);
// 销毁 RenderScript 对象
rs.destroy();
return blurBitmap;
}
```
5. 在 Java 代码中创建一个 AlertDialog 对象,并为其设置 MyAlertDialogTheme 主题:
```
AlertDialog.Builder builder = new AlertDialog.Builder(this, R.style.MyAlertDialogTheme);
builder.setTitle("AlertDialog");
builder.setMessage("This is an AlertDialog with blur background.");
builder.setPositiveButton("OK", null);
builder.setNegativeButton("Cancel", null);
AlertDialog dialog = builder.create();
```
6. 在 Java 代码中获取 dialog_blur_layer 图层,并为其设置高斯模糊后的 Bitmap 对象:
```
// 获取 dialog_blur_layer 图层
ViewGroup decorView = (ViewGroup) dialog.getWindow().getDecorView();
ViewGroup contentContainer = (ViewGroup) decorView.findViewById(android.R.id.content);
FrameLayout container = (FrameLayout) contentContainer.getChildAt(0);
ImageView blurLayer = (ImageView) container.findViewById(R.id.dialog_blur_layer);
// 获取原始图片
Drawable drawable = blurLayer.getDrawable();
Bitmap bitmap = Bitmap.createBitmap(drawable.getIntrinsicWidth(), drawable.getIntrinsicHeight(), Bitmap.Config.ARGB_8888);
Canvas canvas = new Canvas(bitmap);
drawable.setBounds(0, 0, canvas.getWidth(), canvas.getHeight());
drawable.draw(canvas);
// 获取高斯模糊后的图片
Bitmap blurBitmap = getBlurBitmap(this, bitmap);
// 将高斯模糊后的图片设置给 dialog_blur_layer 图层
blurLayer.setImageBitmap(blurBitmap);
```
需要注意的是,Android 中的 RenderScript 只能在 Android 4.2(API 17)及以上版本中使用。在使用 RenderScript 时,需要注意内存的使用和回收,以避免内存泄漏和程序崩溃。
阅读全文
相关推荐
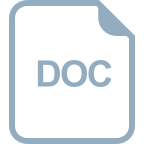
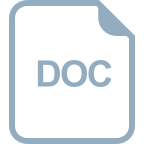
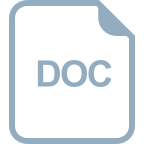
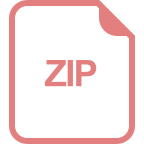
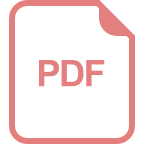
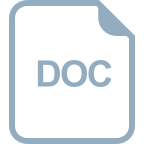
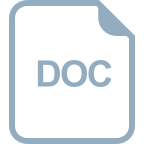
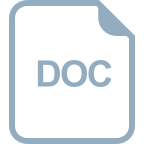
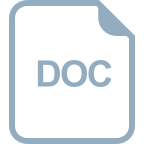
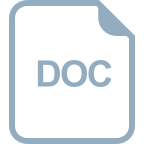
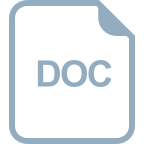
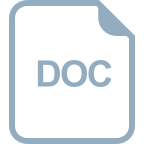
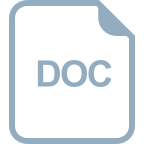
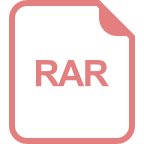
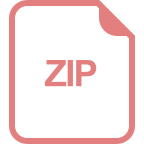
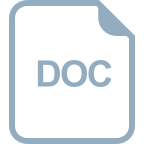
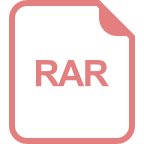
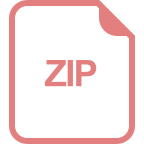