基于qt.4开发打砖块
时间: 2024-05-16 13:18:04 浏览: 9
打砖块是一款经典的游戏,通过qt.4可以很容易地实现这个游戏。下面是一个简单的打砖块游戏示例:
首先,我们需要一个主窗口,可以使用QMainWindow类来实现。在主窗口中,我们需要绘制游戏区域和球、板、砖块等游戏元素。可以使用QPainter类来进行绘制。
接着,我们需要定义游戏逻辑。游戏逻辑包括球的运动轨迹、板的移动、砖块的消除等。当球碰到砖块时,砖块会消失,并且玩家得分增加。当球碰到板时,球的运动方向会改变。如果球碰到边界,游戏失败。
最后,我们需要添加交互逻辑。玩家可以通过键盘控制板的移动。可以使用QKeyEvent类来实现键盘事件的处理。
下面是一个简单的示例代码,仅供参考:
```cpp
#include <QtGui>
class MainWindow : public QMainWindow
{
Q_OBJECT
public:
MainWindow(QWidget *parent = 0);
protected:
void paintEvent(QPaintEvent *event);
void keyPressEvent(QKeyEvent *event);
private:
int ball_x, ball_y;
int ball_dx, ball_dy;
int board_x, board_y;
int board_dx;
int brick[5][10];
int score;
void initGame();
void updateGame();
};
MainWindow::MainWindow(QWidget *parent)
: QMainWindow(parent)
{
setFixedSize(400, 400);
setWindowTitle("打砖块");
initGame();
QTimer *timer = new QTimer(this);
connect(timer, SIGNAL(timeout()), this, SLOT(updateGame()));
timer->start(20);
}
void MainWindow::paintEvent(QPaintEvent *event)
{
QPainter painter(this);
// 绘制游戏区域
painter.setPen(Qt::black);
painter.drawRect(0, 0, 400, 400);
// 绘制球
painter.setBrush(Qt::red);
painter.drawEllipse(ball_x, ball_y, 10, 10);
// 绘制板
painter.setBrush(Qt::blue);
painter.drawRect(board_x, board_y, 60, 10);
// 绘制砖块
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 10; j++) {
if (brick[i][j] > 0) {
QRect rect(j*40, i*20, 40, 20);
painter.setBrush(Qt::green);
painter.drawRect(rect);
}
}
}
// 绘制得分
painter.setPen(Qt::red);
painter.drawText(10, 390, QString("Score: %1").arg(score));
}
void MainWindow::keyPressEvent(QKeyEvent *event)
{
if (event->key() == Qt::Key_Left) {
board_dx = -5;
} else if (event->key() == Qt::Key_Right) {
board_dx = 5;
}
}
void MainWindow::initGame()
{
ball_x = 190;
ball_y = 290;
ball_dx = 5;
ball_dy = -5;
board_x = 170;
board_y = 380;
board_dx = 0;
for (int i = 0; i < 5; i++) {
for (int j = 0; j < 10; j++) {
brick[i][j] = 1;
}
}
score = 0;
}
void MainWindow::updateGame()
{
// 移动球
ball_x += ball_dx;
ball_y += ball_dy;
// 碰到边界
if (ball_x < 0 || ball_x > 390) {
ball_dx = -ball_dx;
}
if (ball_y < 0) {
ball_dy = -ball_dy;
}
if (ball_y > 390) {
initGame();
return;
}
// 碰到板
QRect rect(board_x, board_y, 60, 10);
if (rect.intersects(QRect(ball_x, ball_y, 10, 10))) {
ball_dy = -ball_dy;
}
// 碰到砖块
int row = ball_y / 20;
int col = ball_x / 40;
if (row >= 0 && row < 5 && col >= 0 && col < 10 && brick[row][col] > 0) {
brick[row][col] = 0;
ball_dy = -ball_dy;
score += 10;
}
// 移动板
board_x += board_dx;
if (board_x < 0 || board_x > 340) {
board_dx = 0;
}
update();
}
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
MainWindow window;
window.show();
return app.exec();
}
```
在上面的代码中,我们使用了QTimer类来定时更新游戏状态,使用QKeyEvent类来处理键盘事件,使用QPainter类来进行游戏元素的绘制。通过以上的代码,我们就可以打造出一个简单的打砖块游戏了。
相关推荐
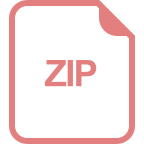














