逆时偏移c语言算法程序
时间: 2023-09-03 17:12:06 浏览: 61
逆时偏移算法是一种加密算法,其基本思路是将明文中的每个字符按照一定规律进行偏移,从而得到密文。逆时偏移算法的加密和解密过程是相反的,因此只需要将加密过程中的偏移量取反即可得到解密算法。
下面是一个简单的逆时偏移算法的 C 语言程序示例:
```c
#include <stdio.h>
#include <string.h>
void decrypt(char* str, int key) {
int len = strlen(str);
for (int i = 0; i < len; i++) {
if (str[i] >= 'a' && str[i] <= 'z') {
str[i] = 'a' + ((str[i] - 'a' - key + 26) % 26);
} else if (str[i] >= 'A' && str[i] <= 'Z') {
str[i] = 'A' + ((str[i] - 'A' - key + 26) % 26);
}
}
}
int main() {
char plaintext[100] = "hello world";
int key = 3;
printf("明文:%s\n", plaintext);
decrypt(plaintext, key);
printf("密文:%s\n", plaintext);
decrypt(plaintext, -key);
printf("解密后:%s\n", plaintext);
return 0;
}
```
该程序中的 `decrypt` 函数实现了逆时偏移算法的解密过程,其参数 `str` 表示待解密的字符串,`key` 表示偏移量。程序通过遍历字符串中的每个字符,如果字符是小写字母则将其转换为 `a` 到 `z` 中的一个字母,如果是大写字母则将其转换为 `A` 到 `Z` 中的一个字母。具体的转换规则是将字符的 ASCII 码值减去偏移量,再加上 26 取模,最后加上 `a` 或 `A` 的 ASCII 码值即可。
在 `main` 函数中,我们使用一个简单的字符串和偏移量进行测试,程序先输出明文,然后调用 `decrypt` 函数进行解密并输出密文,最后再次调用 `decrypt` 函数进行解密并输出解密后的明文。
相关推荐
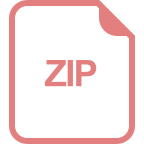

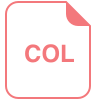












