list std::string constructor type
时间: 2023-11-16 15:05:57 浏览: 32
std::string有4种构造函数类型,分别为:
1. 基于char*的构造函数:可以通过传入char*类型的字符串来创建一个std::string对象。
2. 基于std::string的构造函数:可以通过传入std::string对象来创建另一个std::string对象。
3. 基于迭代器的构造函数:可以利用迭代器来构造std::string对象。
4. 基于重复字符的构造函数:可以通过传入两个参数,一个是单个字符,另一个是重复的次数,来创建一个std::string对象。
相关问题
MqttServer.cpp:125:43: No viable conversion from 'std::string' (aka 'basic_string<char>') to 'const QByteArray' qbytearray.h:181:5: candidate constructor not viable: no known conversion from 'std::string' (aka 'basic_string<char>') to 'const char *' for 1st argument qbytearray.h:189:12: candidate constructor not viable: no known conversion from 'std::string' (aka 'basic_string<char>') to 'QByteArray &&' for 1st argument qbytearray.h:463:12: candidate constructor not viable: no known conversion from 'std::string' (aka 'basic_string<char>') to 'QByteArrayDataPtr' for 1st argument qbytearray.h:524:20: candidate constructor not viable: no known conversion from 'std::string' (aka 'basic_string<char>') to 'const QByteArray &' for 1st argument qbytearray.h:405:67: passing argument to parameter 'hexEncoded' here
这个错误的意思是说,你正在尝试将一个`std::string`类型的变量转换为`QByteArray`类型,但是`QByteArray`没有一个可以接受`std::string`类型参数的构造函数。
要解决这个问题,你需要将`std::string`类型转换为`QByteArray`类型。你可以使用`QByteArray::fromStdString()`函数来实现这一点,例如:
```
std::string myString = "hello";
QByteArray myByteArray = QByteArray::fromStdString(myString);
```
这将把`myString`转换为一个`QByteArray`类型的变量`myByteArray`。你可以在你的代码中使用类似的方法来解决这个问题。
ambiguous overload for 'operator=' (operand types are 'std::string {aka std::basic_string<char>}' and 'long long int')
This error message occurs when you try to assign a long long integer value to a std::string variable using the assignment operator (=). Since there is no overloaded operator= function that takes both a std::string and a long long integer argument, the compiler cannot determine which one to use.
To fix this error, you need to convert the long long integer to a std::string before assigning it to the variable. You can use std::to_string() function to convert the long long integer to a string as shown below:
```
std::string str = std::to_string(100LL); // convert long long integer to string
```
Alternatively, you can use a constructor of std::string that takes a const char* argument to create a string from a C-style string representation of the long long integer:
```
std::string str = std::string("100"); // create string from C-style string
```
In either case, the resulting string can be assigned to the std::string variable without any ambiguity.
相关推荐
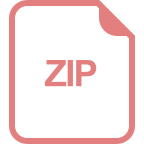












