with open('室外温度数据', 'r', encoding='utf-8', errors='replace') as file: FileNotFoundError: [Errno 2] No such file or directory: '室外温度数据'
时间: 2023-12-26 15:03:57 浏览: 39
这个错误通常表示在你尝试打开一个不存在的文件时出现了错误。请确保你的文件名拼写正确,并且该文件位于正确的文件路径下。
另外,需要注意的是,文件名应该包括文件的扩展名。例如,如果你的文件是一个CSV文件,文件名应该是"室外温度数据.csv"。
如果你不确定文件路径和文件名是否正确,可以尝试使用绝对路径来打开文件。例如:
```
with open('/path/to/室外温度数据.csv', 'r', encoding='utf-8', errors='replace') as file:
data = file.read()
```
请注意,这里的"/path/to/"应该替换为实际文件所在的绝对路径。
相关问题
str.encode(encoding="utf-8", errors="strict")
`str.encode(encoding="utf-8", errors="strict")`是一个Python字符串方法,它返回给定字符串的编码版本。此方法使用给定编码格式将字符串编码为字节序列。如果给定编码格式不支持,则会引发`LookupError`异常。下面是一个例子,演示如何使用这个方法:
```python
s = '你好,世界!'
b = s.encode('utf-8')
print(b)
```
输出:
```
b'\xe4\xbd\xa0\xe5\xa5\xbd\xef\xbc\x8c\xe4\xb8\x96\xe7\x95\x8c\xef\xbc\x81'
```
在这个例子中,我们将字符串`s`使用utf-8进行编码,并赋值给变量`b`,最终在控制台上输出了字节序列`b'\xe4\xbd\xa0\xe5\xa5\xbd\xef\xbc\x8c\xe4\xb8\x96\xe7\x95\x8c\xef\xbc\x81'`。
`encoding`参数是可选的,默认值为"utf-8",它指定了要使用的字符编码。如果你想使用其他编码格式,你可以在这里指定。
`errors`参数也是可选的,默认值为"strict",它指定了如何处理编码错误。如果你不想处理错误,你可以将其设置为"ignore"或"replace"。
with open(os.path.join(root, filename), "r", encoding='utf-8', errors='gbk') as long_file_line: content = long_file_line.readlines()
这段代码的作用是打开一个文件,并读取其中的所有行内容。具体来说,它使用了Python内置的open函数打开了文件,其中os.path.join(root, filename)是文件的完整路径,"r"表示以只读模式打开文件,encoding='utf-8'表示以UTF-8编码读取文件,errors='gbk'表示在遇到无法解码的字符时采用GBK编码进行替换。接着,使用readlines()方法将文件内容按行读取并存储到列表content中。
相关推荐
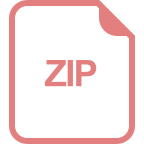
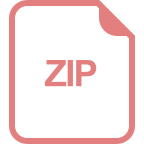
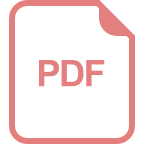
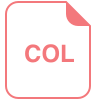











