用py3写一个变更文件归档的功能
时间: 2024-04-29 08:24:48 浏览: 95
好的,这个问题很简单。您可以使用Python的os和shutil模块来实现将文件归档到指定目录的功能。示例代码如下:
```
import os
import shutil
def archive_files(source_dir, target_dir):
for root, dirs, files in os.walk(source_dir):
for file in files:
source_path = os.path.join(root, file)
target_path = os.path.join(target_dir, file)
shutil.move(source_path, target_path)
source_dir = '/path/to/source/directory'
target_dir = '/path/to/target/directory'
archive_files(source_dir, target_dir)
```
您需要将`source_dir`和`target_dir`替换为您实际的源目录和目标目录。当运行Python脚本时,它将遍历源目录中的所有文件,并将它们移动到目标目录中。如果目标目录中已经存在同名文件,则旧文件将被覆盖。
相关问题
python脚本完成 变更文件归档、旧脚本备份、作业参数文件备份
以下是一个Python脚本,它可以根据指定的目录和规则,自动将变更文件归档、备份旧脚本和作业参数文件。
```python
import os
import shutil
import datetime
change_dir = "/path/to/change/files"
backup_dir = "/path/to/backup/files"
param_dir = "/path/to/parameter/files"
# 归档变更文件
today = datetime.date.today()
change_archive_dir = os.path.join(backup_dir, "change_archive_{}".format(today))
os.makedirs(change_archive_dir, exist_ok=True)
for root, dirs, files in os.walk(change_dir):
for file in files:
if file.endswith(".txt"):
file_path = os.path.join(root, file)
shutil.move(file_path, change_archive_dir)
# 备份旧脚本
script_dir = "/path/to/scripts"
script_backup_dir = os.path.join(backup_dir, "script_backup_{}".format(today))
os.makedirs(script_backup_dir, exist_ok=True)
for root, dirs, files in os.walk(script_dir):
for file in files:
if file.endswith(".py"):
file_path = os.path.join(root, file)
backup_path = os.path.join(script_backup_dir, file)
shutil.copy(file_path, backup_path)
# 备份作业参数文件
param_backup_dir = os.path.join(param_dir, "param_backup_{}".format(today))
os.makedirs(param_backup_dir, exist_ok=True)
for root, dirs, files in os.walk(param_dir):
for file in files:
if file.endswith(".txt"):
file_path = os.path.join(root, file)
backup_path = os.path.join(param_backup_dir, file)
shutil.copy(file_path, backup_path)
```
这个脚本会将指定目录下所有扩展名为`.txt`的文件移动到以当前日期命名的归档目录中。它还会备份指定目录下所有扩展名为`.py`的文件到以当前日期命名的备份目录中,并将指定目录下所有扩展名为`.txt`的文件备份到另一个以当前日期命名的备份目录中。
阅读全文
相关推荐
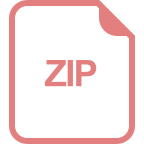
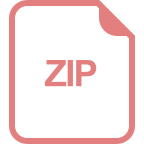
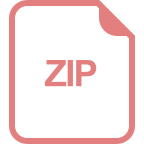
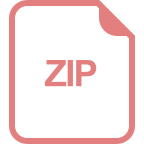
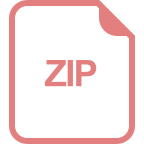
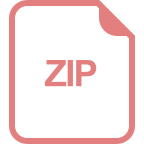
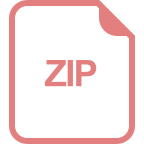
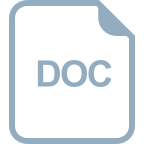
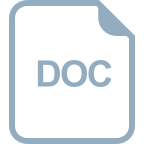
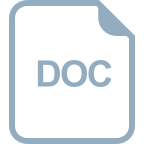
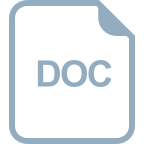
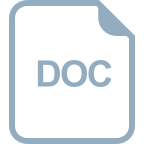
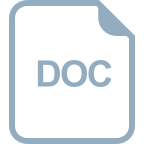
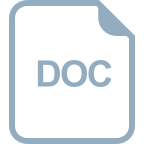
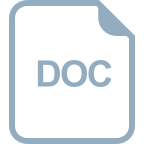
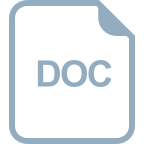
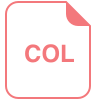
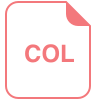