(1) 构建学生(Student)类,包含学号、姓名、出生年月基本信息,离散数学、大学物理、高等数学、面向对象程序设计(Java)成绩及平均分。 (2) 应用集合 架实现某班所有学生的成绩管理,计算所有学生的平均分;应用Comparable接口或 Compartor实现平均分比较,能够对所有学生按分均分排序并输出。 (3) 将每位学生的信息按照平均分的排序输出到屏幕上。
时间: 2024-03-20 07:44:52 浏览: 18
好的,这是一个典型的Java编程问题。我可以给您提供一个简单的实现思路,希望能够帮助到您。
1. 首先创建一个学生类,包含学号、姓名、出生年月和成绩等属性。可以添加以下方法:
```java
public class Student {
private int id; // 学号
private String name; // 姓名
private Date birthday; // 出生日期
private double mathScore; // 离散数学成绩
private double physicsScore; // 大学物理成绩
private double calculusScore; // 高等数学成绩
private double javaScore; // 面向对象程序设计(Java)成绩
public Student(int id, String name, Date birthday, double mathScore, double physicsScore, double calculusScore, double javaScore) {
this.id = id;
this.name = name;
this.birthday = birthday;
this.mathScore = mathScore;
this.physicsScore = physicsScore;
this.calculusScore = calculusScore;
this.javaScore = javaScore;
}
// 计算平均分
public double calculateAverageScore() {
return (mathScore + physicsScore + calculusScore + javaScore) / 4;
}
// 获取学号
public int getId() {
return id;
}
// 获取姓名
public String getName() {
return name;
}
// 获取出生日期
public Date getBirthday() {
return birthday;
}
// 获取离散数学成绩
public double getMathScore() {
return mathScore;
}
// 获取大学物理成绩
public double getPhysicsScore() {
return physicsScore;
}
// 获取高等数学成绩
public double getCalculusScore() {
return calculusScore;
}
// 获取面向对象程序设计(Java)成绩
public double getJavaScore() {
return javaScore;
}
}
```
2. 创建一个管理学生成绩的类,使用List集合存储所有学生信息。可以添加以下方法:
```java
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
public class StudentScoreManager {
private List<Student> students;
public StudentScoreManager(List<Student> students) {
this.students = students;
}
// 计算所有学生的平均分
public double calculateAverageScore() {
double totalScore = 0;
for (Student student : students) {
totalScore += student.calculateAverageScore();
}
return totalScore / students.size();
}
// 对所有学生按平均分排序并输出
public void sortAndPrint() {
Collections.sort(students, new Comparator<Student>() {
@Override
public int compare(Student s1, Student s2) {
double averageScore1 = s1.calculateAverageScore();
double averageScore2 = s2.calculateAverageScore();
return Double.compare(averageScore2, averageScore1);
}
});
for (Student student : students) {
System.out.println("学号:" + student.getId()
+ ",姓名:" + student.getName()
+ ",平均分:" + student.calculateAverageScore());
}
}
}
```
3. 可以编写一个测试类,创建多个学生对象,添加到List集合中,然后创建一个学生成绩管理器对象,调用其中的方法进行测试。
```java
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class Test {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
students.add(new Student(1001, "张三", new Date(1995, 5, 15), 80, 85, 90, 95));
students.add(new Student(1002, "李四", new Date(1996, 8, 20), 75, 90, 85, 80));
students.add(new Student(1003, "王五", new Date(1997, 3, 8), 90, 80, 95, 85));
StudentScoreManager manager = new StudentScoreManager(students);
double averageScore = manager.calculateAverageScore();
System.out.println("所有学生的平均分:" + averageScore);
manager.sortAndPrint();
}
}
```
这样就实现了一个简单的学生成绩管理系统。希望能够对您有所帮助。
相关推荐
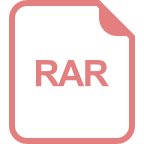
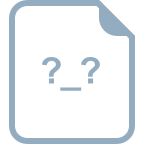














