下面这段代码中的子进程是否并行执行 int main(){ int i; pid_t pid; for(i = 0; i < 200; i++){ pid = fork(); if(-1==pid){ printf("fork:%m\n"); exit(-1); } else if(pid == 0){ break; } } if(i <= 200){
时间: 2024-04-21 07:29:09 浏览: 22
是的,子进程是并行执行的。这段代码创建了一个循环,在循环中调用 fork() 函数创建子进程。如果 fork() 返回值为 0,则说明当前进程是子进程,此时会跳出循环体并执行 break 语句。因此,在这个循环中创建的子进程会同时运行,而且它们之间的执行顺序是不确定的。如果 i 的值小于等于 200,则说明当前进程是一个子进程,因此会执行 if 语句中的代码。否则,说明当前进程是父进程,会执行 if 语句之后的代码。
相关问题
#include <sys/types.h> #include <unistd.h> #include <stdio.h> #include <stdlib.h> int main(){ pid_t pid; char *message; int n; pid=fork(); if (pid<0){ perror("fork failed"); exit(1); } if(pid==0){ message="This is the children\n"; n=6; } else { message="This is the parent\n"; n=3; } for(;n>0;n--){ printf(message); // sleep(1); } return 0; } 请分析以上代码在linux中运行结果
在Linux中,以上代码会输出以下结果:
```
This is the parent
This is the parent
This is the parent
This is the children
This is the children
This is the children
```
当程序运行到 `fork()` 函数时,会创建一个新的子进程,该子进程完全复制了父进程的所有内容。然后,父进程和子进程都会从 `fork()` 函数返回,但是返回值不同。在父进程中,`pid` 的值是子进程的进程ID,而在子进程中,`pid` 的值为0。
因此,在程序中,父进程和子进程都会执行 `for` 循环中的代码,但是由于输出的 `message` 不同,因此输出内容也不同。由于父进程和子进程是并行执行的,所以输出的顺序可能不同,但是肯定会包含父进程和子进程各自的输出。
linux多进程并行循环怎么写
在Linux中,可以使用fork函数创建子进程,使用wait函数等待子进程结束,使用exec函数执行程序。下面是一种多进程并行循环的写法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
int i, j;
pid_t pid;
for (i = 0; i < 10; i++) {
pid = fork();
if (pid == 0) { // 子进程
for (j = 0; j < 10; j++) {
printf("Child %d: %d\n", getpid(), j);
}
exit(0); // 子进程结束
} else if (pid > 0) { // 父进程
// do nothing
} else { // fork失败
printf("Fork error.\n");
exit(1);
}
}
// 父进程等待子进程结束
for (i = 0; i < 10; i++) {
wait(NULL);
}
return 0;
}
```
上面的代码中,先使用for循环创建了10个子进程,每个子进程循环输出10个数字。父进程等待10个子进程结束后才结束。这样就实现了多进程并行循环。
相关推荐
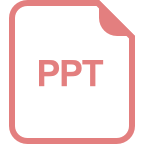
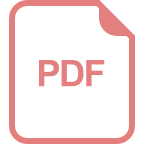
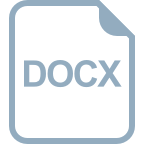









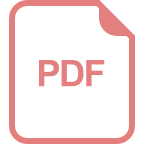
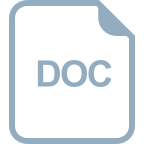
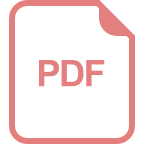
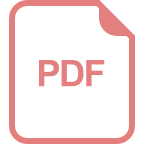