在Linux平台上编写一个C语言应用程序,采用多进程的方法,实现下列两个任务并行: 1)每隔1秒在屏幕上打印出自己的中文姓名;
时间: 2024-05-02 20:16:41 浏览: 114
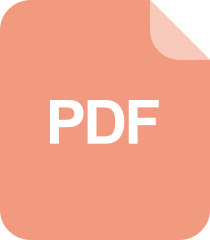
用C语言编写的Linux应用程序
2)每隔5秒在屏幕上打印出自己的英文名字。
下面是一个基本的实现,使用了fork()函数创建两个子进程,分别执行两个任务。其中,使用sleep()函数来控制每个任务的执行时间。请注意,由于中文字符在终端上的显示可能存在问题,因此建议将中文姓名替换为英文字符。
```c
#include <stdio.h>
#include <unistd.h>
#include <stdlib.h>
#include <sys/wait.h>
void print_chinese_name() {
char *name = "Your Chinese Name";
while (1) {
printf("%s\n", name);
sleep(1);
}
}
void print_english_name() {
char *name = "Your English Name";
while (1) {
printf("%s\n", name);
sleep(5);
}
}
int main() {
pid_t pid1, pid2;
pid1 = fork();
if (pid1 == 0) {
// child process 1
print_chinese_name();
exit(0);
} else if (pid1 > 0) {
pid2 = fork();
if (pid2 == 0) {
// child process 2
print_english_name();
exit(0);
} else if (pid2 > 0) {
// parent process
wait(NULL);
wait(NULL);
printf("All child processes have terminated.\n");
} else {
perror("Error: fork() failed.\n");
exit(1);
}
} else {
perror("Error: fork() failed.\n");
exit(1);
}
return 0;
}
```
在实际使用过程中,可以根据具体需求对代码进行修改和优化。
阅读全文
相关推荐
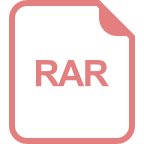
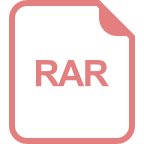
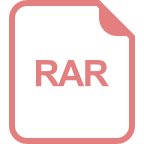
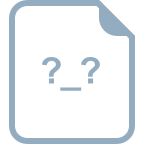
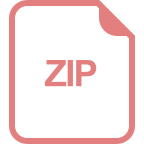
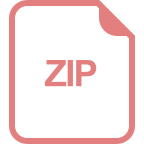
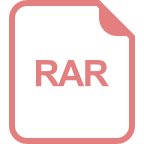
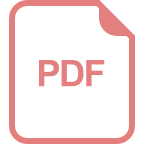
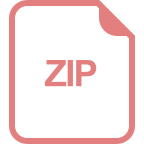
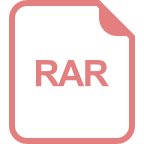
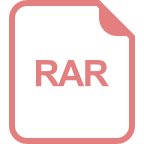
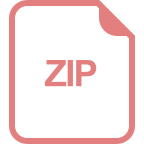