用html+css+js写一个粒子光束生长的完整代码
时间: 2023-07-15 14:12:14 浏览: 72
以下是一个简单的粒子光束生长动画的HTML + CSS + JS代码示例:
HTML
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Particle Beam Growth</title>
<style>
body {
margin: 0;
padding: 0;
}
canvas {
display: block;
}
</style>
</head>
<body>
<canvas id="canvas"></canvas>
<script src="particle_beam.js"></script>
</body>
</html>
```
CSS
```css
canvas {
background-color: #000;
}
```
JavaScript
```javascript
const canvas = document.getElementById('canvas');
const context = canvas.getContext('2d');
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
let particles = [];
const particleCount = 100;
let particleSpeed = 1;
// Create particles
for (let i = 0; i < particleCount; i++) {
particles.push(new particle());
}
function particle() {
// Randomize initial position
this.x = Math.random() * canvas.width;
this.y = Math.random() * canvas.height;
// Randomize direction
this.direction = Math.random() * 360;
// Randomize speed
this.speed = Math.random() * particleSpeed;
// Randomize color
this.color = `rgb(${Math.random() * 255}, ${Math.random() * 255}, ${Math.random() * 255})`;
// Update particle position
this.update = function() {
let radians = this.direction * Math.PI / 180;
this.x += Math.cos(radians) * this.speed;
this.y += Math.sin(radians) * this.speed;
}
// Draw particle
this.draw = function() {
context.beginPath();
context.arc(this.x, this.y, 3, 0, Math.PI * 2);
context.fillStyle = this.color;
context.fill();
}
}
function animate() {
context.clearRect(0, 0, canvas.width, canvas.height);
// Draw particles
for (let i = 0; i < particles.length; i++) {
particles[i].update();
particles[i].draw();
}
// Draw beam
context.beginPath();
context.moveTo(canvas.width / 2, canvas.height / 2);
context.lineTo(particles[0].x, particles[0].y);
context.strokeStyle = '#fff';
context.lineWidth = 2;
context.stroke();
requestAnimationFrame(animate);
}
animate();
```
这个示例创建了一个包含100个随机颜色、位置、方向和速度的粒子数组。粒子通过其方向和速度更新其位置,并在每个帧上绘制其颜色的圆形。在画布中央绘制了一条白色的光束,其中一端连接到画布中心,另一端连接到数组中的第一个粒子。通过调整`particleSpeed`变量的值,可以调整粒子移动的速度。
相关推荐
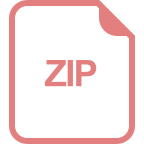
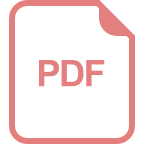














