用html+css+js写一个粒子光束生长的代码
时间: 2023-07-15 12:12:19 浏览: 66
以下是一个简单的粒子光束生长的示例代码,使用了 HTML、CSS 和 JavaScript。
HTML:
```html
<!DOCTYPE html>
<html>
<head>
<title>Particle Beam Growth</title>
<style>
body {
background-color: black;
margin: 0;
padding: 0;
}
canvas {
display: block;
}
</style>
</head>
<body>
<canvas></canvas>
<script src="particle-beam.js"></script>
</body>
</html>
```
CSS:
```css
canvas {
width: 100%;
height: 100%;
}
```
JavaScript:
```js
const canvas = document.querySelector("canvas");
const ctx = canvas.getContext("2d");
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
window.addEventListener("resize", () => {
canvas.width = window.innerWidth;
canvas.height = window.innerHeight;
});
class Particle {
constructor(x, y, dx, dy, size, color) {
this.x = x;
this.y = y;
this.dx = dx;
this.dy = dy;
this.size = size;
this.color = color;
}
draw() {
ctx.beginPath();
ctx.arc(this.x, this.y, this.size, 0, Math.PI * 2, false);
ctx.fillStyle = this.color;
ctx.fill();
}
update() {
if (this.x + this.size > canvas.width || this.x - this.size < 0) {
this.dx = -this.dx;
}
if (this.y + this.size > canvas.height || this.y - this.size < 0) {
this.dy = -this.dy;
}
this.x += this.dx;
this.y += this.dy;
this.draw();
}
}
class ParticleBeam {
constructor(x, y, color) {
this.particles = [];
this.color = color;
for (let i = 0; i < 30; i++) {
const dx = Math.random() - 0.5;
const dy = Math.random() - 0.5;
const size = Math.random() * 3;
const particle = new Particle(x, y, dx, dy, size, this.color);
this.particles.push(particle);
}
}
draw() {
this.particles.forEach((particle) => {
particle.update();
});
}
}
let particleBeams = [];
function animate() {
requestAnimationFrame(animate);
ctx.clearRect(0, 0, canvas.width, canvas.height);
particleBeams.forEach((particleBeam, index) => {
if (particleBeam.particles.length <= 0) {
particleBeams.splice(index, 1);
} else {
particleBeam.draw();
}
});
}
canvas.addEventListener("click", (event) => {
const x = event.clientX;
const y = event.clientY;
const color = `rgb(${Math.random() * 255}, ${Math.random() * 255}, ${
Math.random() * 255
})`;
const particleBeam = new ParticleBeam(x, y, color);
particleBeams.push(particleBeam);
});
animate();
```
这段代码使用了 Canvas 来绘制粒子,每次点击鼠标时会在点击位置生成一个随机颜色的粒子光束,并在画布上逐渐生长。在动画循环中,会遍历所有粒子光束并更新并绘制所有粒子。当一个粒子光束的粒子数为0时,将从粒子光束数组中移除。
相关推荐
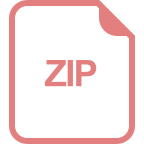
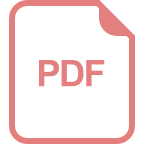














