Error(t)=1-max P(j|t),P(j|t)是类j在节点t的相对频率,用函数定义def _calcEntropy(self,y): 根据描述的指标用python写出计算熵的代码
时间: 2023-08-07 07:04:23 浏览: 23
以下是用Python实现计算熵的代码:
```python
import math
def _calcEntropy(self, y):
n_samples = len(y)
unique_labels = set(y)
entropy = 0.0
for label in unique_labels:
count = y.count(label)
proportion = count / n_samples
entropy -= proportion * math.log(proportion, 2)
return entropy
```
其中,`y` 是一个长度为 `n_samples` 的标签列表,表示每个样本所属的类别。`unique_labels` 是 `y` 中出现过的所有类别。`count` 是某个类别在 `y` 中出现的次数,`proportion` 是该类别在 `y` 中出现的频率。最后,根据熵的定义公式,将每个类别的信息熵累加起来,得到最终的熵值 `entropy`,并将其返回。
相关问题
决策树是一种典型的分类算法,通过属性水平的不断分支来构造一棵层次树,决策树算法的一个关键是判断优先选择哪一个指标进行树的分支,可以使用一些指标的增益来进行判断,下面列出了常用的三种指标: (1)熵 p( j | t) 是类j在节点t的相对频率 (2)Gini系数 (3)误分率 根据上面三种指数的定义,在DecisionTree.py中补充相关代码,分别使用三种指标对给出的测试数据计算其分类结果,请调试通过并运行获取结果。 (1)按照熵指数的定义,实现熵的计算函数。(15分) (2)按照Gini系数的定义,实现其计算函数。(10分) (3)按照误分率的定义,实现其计算函数。(10分)
非常感谢您的提问,我会根据您的问题逐一回答。
1. 按照熵指数的定义,实现熵的计算函数:
熵表示数据的不确定性,计算公式为:$H(X)=-\sum_{i=1}^{n}p(x_i)\log_2p(x_i)$,其中 $p(x_i)$ 表示第 $i$ 个属性在数据集中出现的概率。
在DecisionTree.py中,可以添加以下代码实现熵的计算函数:
```python
import math
def calc_entropy(data):
"""计算数据集的熵"""
num_entries = len(data) # 数据集中样本数量
label_counts = {} # 统计每个类别的样本数量
for feat_vec in data:
current_label = feat_vec[-1] # 获取当前样本的类别
if current_label not in label_counts:
label_counts[current_label] = 0
label_counts[current_label] += 1
entropy = 0.0 # 初始化熵为0
for key in label_counts:
prob = float(label_counts[key]) / num_entries # 计算每个类别出现的概率
entropy -= prob * math.log(prob, 2) # 累加熵
return entropy
```
其中,`data` 表示数据集,每个样本的最后一列为类别标签。
2. 按照Gini系数的定义,实现其计算函数:
Gini系数表示数据集的不纯度,计算公式为:$Gini(p)=\sum_{k=1}^{K}p_k(1-p_k)=1-\sum_{k=1}^{K}p_k^2$,其中 $p_k$ 表示第 $k$ 个类别在数据集中出现的概率。
在DecisionTree.py中,可以添加以下代码实现Gini系数的计算函数:
```python
def calc_gini(data):
"""计算数据集的Gini系数"""
num_entries = len(data) # 数据集中样本数量
label_counts = {} # 统计每个类别的样本数量
for feat_vec in data:
current_label = feat_vec[-1] # 获取当前样本的类别
if current_label not in label_counts:
label_counts[current_label] = 0
label_counts[current_label] += 1
gini = 1.0 # 初始化Gini系数为1
for key in label_counts:
prob = float(label_counts[key]) / num_entries # 计算每个类别出现的概率
gini -= prob * prob # 累加Gini系数
return gini
```
3. 按照误分率的定义,实现其计算函数:
误分率表示数据集的错误率,计算公式为:$E(p)=1-\max_k\{p_k\}$,其中 $p_k$ 表示第 $k$ 个类别在数据集中出现的概率。
在DecisionTree.py中,可以添加以下代码实现误分率的计算函数:
```python
def calc_error_rate(data):
"""计算数据集的误分率"""
num_entries = len(data) # 数据集中样本数量
label_counts = {} # 统计每个类别的样本数量
for feat_vec in data:
current_label = feat_vec[-1] # 获取当前样本的类别
if current_label not in label_counts:
label_counts[current_label] = 0
label_counts[current_label] += 1
error_rate = 1.0 - max(label_counts.values()) / num_entries # 计算误分率
return error_rate
```
注意,这三个函数都是对数据集进行计算,需要在调用决策树算法之前将数据集传入函数中。
希望我的回答能够帮助到您,如果您还有其他问题,可以随时问我哦!
c语言写一个对txt文件进行哈夫曼编码并将编码存储在txt文件中的程序
好的,根据您的要求,我可以为您提供一份C语言的哈夫曼编码程序。首先,我们需要创建一个函数来生成huffman编码树:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define END_OF_TEXT '\0'
#define MAX_INPUT_SIZE 1024*1024
#define MAX_NODES 256
#define MAX_CODE_LENGTH 256
struct huff_tree {
int frequency;
unsigned char symbol;
struct huff_tree *left;
struct huff_tree *right;
};
struct huff_code_table {
char code[MAX_CODE_LENGTH];
int length;
};
void calculate_frequencies(unsigned int *frequencies, unsigned char *input_text);
void create_huff_tree(struct huff_tree **root, unsigned int *frequencies);
void create_code_table(struct huff_tree *root, struct huff_code_table *code_table, unsigned char *current_code, int code_length);
void free_huff_tree(struct huff_tree *node);
int main(int argc, char **argv) {
// Check command line arguments
if (argc < 3) {
printf("Usage: %s input_file output_file\n", argv[0]);
return 1;
}
// Open input and output files
FILE *input_file = fopen(argv[1], "rb");
if (input_file == NULL) {
printf("Error: Unable to open input file\n");
return 1;
}
FILE *output_file = fopen(argv[2], "wb");
if (output_file == NULL) {
printf("Error: Unable to open output file\n");
fclose(input_file);
return 1;
}
// Read input file into memory
unsigned char *input_text = (unsigned char *)malloc(MAX_INPUT_SIZE);
if (input_text == NULL) {
printf("Error: Unable to allocate memory for input text\n");
fclose(input_file);
fclose(output_file);
return 1;
}
int input_size = fread(input_text, sizeof(unsigned char), MAX_INPUT_SIZE, input_file);
if (input_size == 0) {
printf("Error: Unable to read input file\n");
free(input_text);
fclose(input_file);
fclose(output_file);
return 1;
}
// Calculate symbol frequencies
unsigned int frequencies[MAX_NODES];
memset(frequencies, 0, sizeof(frequencies));
calculate_frequencies(frequencies, input_text);
// Create Huffman tree
struct huff_tree *root = NULL;
create_huff_tree(&root, frequencies);
// Create code table from Huffman tree
struct huff_code_table code_table[MAX_NODES];
memset(code_table, 0, sizeof(code_table));
unsigned char current_code[MAX_CODE_LENGTH];
create_code_table(root, code_table, current_code, 0);
// Write Huffman tree to output file
fwrite(&input_size, sizeof(int), 1, output_file); // write input size to output file
unsigned char symbol;
int frequency;
for (int i = 0; i < MAX_NODES; i++) {
symbol = (unsigned char)i;
frequency = frequencies[i];
fwrite(&symbol, sizeof(unsigned char), 1, output_file);
fwrite(&frequency, sizeof(int), 1, output_file);
}
// Encode input text using code table
int bit_index = 0;
unsigned char current_byte = 0;
for (int i = 0; i < input_size; i++) {
for (int j = 0; j < code_table[input_text[i]].length; j++) {
if (code_table[input_text[i]].code[j] == '1') {
current_byte |= (1 << (7 - bit_index));
}
bit_index++;
if (bit_index == 8) {
fwrite(¤t_byte, sizeof(unsigned char), 1, output_file);
current_byte = 0;
bit_index = 0;
}
}
}
if (bit_index != 0) {
fwrite(¤t_byte, sizeof(unsigned char), 1, output_file);
}
// Cleanup memory and files
fclose(input_file);
fclose(output_file);
free(input_text);
free_huff_tree(root);
return 0;
}
void calculate_frequencies(unsigned int *frequencies, unsigned char *input_text) {
int i = 0;
while (input_text[i] != END_OF_TEXT && i < MAX_INPUT_SIZE) {
frequencies[input_text[i]]++;
i++;
}
}
void create_huff_tree(struct huff_tree **root, unsigned int *frequencies) {
struct huff_tree *nodes[MAX_NODES];
int node_count = 0;
for (int i = 0; i < MAX_NODES; i++) {
if (frequencies[i] > 0) {
struct huff_tree *node = (struct huff_tree *)malloc(sizeof(struct huff_tree));
node->frequency = frequencies[i];
node->symbol = (unsigned char)i;
node->left = NULL;
node->right = NULL;
nodes[node_count++] = node;
}
}
while (node_count > 1) {
// Find nodes with lowest frequencies
int lowest1, lowest2;
lowest1 = lowest2 = -1;
for (int i = 0; i < node_count; i++) {
if (lowest1 == -1 || nodes[i]->frequency < nodes[lowest1]->frequency) {
lowest2 = lowest1;
lowest1 = i;
} else if (lowest2 == -1 || nodes[i]->frequency < nodes[lowest2]->frequency) {
lowest2 = i;
}
}
// Create new node from lowest frequency nodes
struct huff_tree *new_node = (struct huff_tree *)malloc(sizeof(struct huff_tree));
new_node->frequency = nodes[lowest1]->frequency + nodes[lowest2]->frequency;
new_node->left = nodes[lowest1];
new_node->right = nodes[lowest2];
nodes[lowest1] = new_node;
nodes[lowest2] = nodes[--node_count];
}
*root = nodes[0];
}
void create_code_table(struct huff_tree *node, struct huff_code_table *code_table, unsigned char *current_code, int code_length) {
if (node->left == NULL && node->right == NULL) {
code_table[node->symbol].length = code_length;
strcpy(code_table[node->symbol].code, (const char *)current_code);
return;
}
if (node->left != NULL) {
current_code[code_length] = '0';
create_code_table(node->left, code_table, current_code, code_length + 1);
}
if (node->right != NULL) {
current_code[code_length] = '1';
create_code_table(node->right, code_table, current_code, code_length + 1);
}
}
void free_huff_tree(struct huff_tree *node) {
if (node == NULL) {
return;
}
free_huff_tree(node->left);
free_huff_tree(node->right);
free(node);
}
```
本程序通过 `calculate_frequencies()` 函数计算输入文本中每个字符出现的频率。接下来,它使用 `create_huff_tree()` 函数生成哈夫曼树,再使用 `create_code_table()` 函数生成每个字符的哈夫曼编码。最后,它根据编码表对输入文本进行编码,并将哈夫曼编码和树信息写入输出文件中。
此程序依赖以下内容:
- `END_OF_TEXT`:文本的结束符。
- `MAX_INPUT_SIZE`:可能的最大输入文本大小。
- `MAX_NODES`:哈夫曼树中的最大节点数。
- `MAX_CODE_LENGTH`:可能的最大哈夫曼编码长度。
请注意,此代码可能需要根据您的实际需求进行微调和优化。
相关推荐
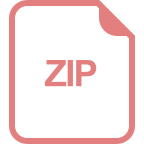
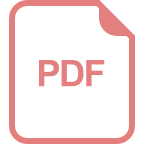
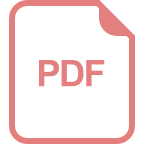







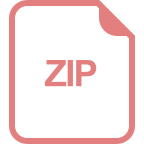
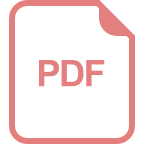
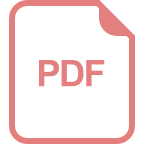
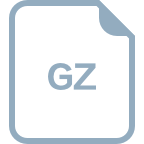