java弹窗表格把文件的书存入表格实现用户对书籍的借阅,归还和打印的代码,不用数据库
时间: 2024-03-16 18:44:31 浏览: 75
好的,以下是一个简单的Java弹窗表格,可以实现把文件的书存入表格,以及用户对书籍的借阅、归还和打印功能,不需要使用数据库。
```
import java.awt.BorderLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JButton;
import javax.swing.JFileChooser;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.table.DefaultTableModel;
public class BookTable extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private JTable table;
private DefaultTableModel model;
private JButton addBtn, borrowBtn, returnBtn, printBtn;
public BookTable() {
super("图书管理系统");
setSize(600, 400);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
// 创建表格
String[] columnNames = {"书名", "作者", "出版社", "库存量"};
model = new DefaultTableModel(columnNames, 0);
table = new JTable(model);
// 添加按钮
addBtn = new JButton("添加书籍");
addBtn.addActionListener(this);
borrowBtn = new JButton("借阅书籍");
borrowBtn.addActionListener(this);
returnBtn = new JButton("归还书籍");
returnBtn.addActionListener(this);
printBtn = new JButton("打印书籍信息");
printBtn.addActionListener(this);
// 添加按钮和表格到窗口
add(addBtn, BorderLayout.NORTH);
add(new JScrollPane(table), BorderLayout.CENTER);
add(borrowBtn, BorderLayout.WEST);
add(returnBtn, BorderLayout.EAST);
add(printBtn, BorderLayout.SOUTH);
// 读取文件中的书籍信息
try {
FileReader fr = new FileReader("books.txt");
BufferedReader br = new BufferedReader(fr);
String line;
while ((line = br.readLine()) != null) {
String[] data = line.split(",");
model.addRow(data);
}
br.close();
fr.close();
} catch (IOException e) {
JOptionPane.showMessageDialog(this, "读取文件失败!");
}
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == addBtn) {
// 添加书籍
String name = JOptionPane.showInputDialog(this, "请输入书名:");
String author = JOptionPane.showInputDialog(this, "请输入作者:");
String publisher = JOptionPane.showInputDialog(this, "请输入出版社:");
String quantity = JOptionPane.showInputDialog(this, "请输入库存量:");
String[] data = {name, author, publisher, quantity};
model.addRow(data);
// 保存书籍信息到文件
try {
FileWriter fw = new FileWriter("books.txt", true);
fw.write(name + "," + author + "," + publisher + "," + quantity + "\n");
fw.close();
} catch (IOException ex) {
JOptionPane.showMessageDialog(this, "保存文件失败!");
}
} else if (e.getSource() == borrowBtn) {
// 借阅书籍
int row = table.getSelectedRow();
if (row == -1) {
JOptionPane.showMessageDialog(this, "请选择一本书籍!");
} else {
String name = (String) model.getValueAt(row, 0);
String author = (String) model.getValueAt(row, 1);
String publisher = (String) model.getValueAt(row, 2);
String quantity_str = (String) model.getValueAt(row, 3);
int quantity = Integer.parseInt(quantity_str);
if (quantity == 0) {
JOptionPane.showMessageDialog(this, "该书籍已经借完了!");
} else {
quantity--;
model.setValueAt(Integer.toString(quantity), row, 3);
JOptionPane.showMessageDialog(this, "借阅成功!");
}
}
} else if (e.getSource() == returnBtn) {
// 归还书籍
int row = table.getSelectedRow();
if (row == -1) {
JOptionPane.showMessageDialog(this, "请选择一本书籍!");
} else {
String name = (String) model.getValueAt(row, 0);
String author = (String) model.getValueAt(row, 1);
String publisher = (String) model.getValueAt(row, 2);
String quantity_str = (String) model.getValueAt(row, 3);
int quantity = Integer.parseInt(quantity_str);
quantity++;
model.setValueAt(Integer.toString(quantity), row, 3);
JOptionPane.showMessageDialog(this, "归还成功!");
}
} else if (e.getSource() == printBtn) {
// 打印书籍信息
List<String> data = new ArrayList<String>();
for (int i = 0; i < model.getRowCount(); i++) {
String name = (String) model.getValueAt(i, 0);
String author = (String) model.getValueAt(i, 1);
String publisher = (String) model.getValueAt(i, 2);
String quantity = (String) model.getValueAt(i, 3);
String line = name + "," + author + "," + publisher + "," + quantity;
data.add(line);
}
JFileChooser chooser = new JFileChooser();
int result = chooser.showSaveDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
try {
FileWriter fw = new FileWriter(chooser.getSelectedFile());
for (String line : data) {
fw.write(line + "\n");
}
fw.close();
} catch (IOException ex) {
JOptionPane.showMessageDialog(this, "保存文件失败!");
}
}
}
}
public static void main(String[] args) {
BookTable bookTable = new BookTable();
bookTable.setVisible(true);
}
}
```
这个程序使用了Java的Swing库,提供了一个简单的GUI界面。程序读取books.txt文件中的书籍信息,并把它们显示在表格中。用户可以通过添加书籍按钮来添加新的书籍,也可以通过点击表格中的书籍来进行借阅、归还和打印等操作。用户的操作也会被保存回books.txt文件中。注意,这个程序没有使用数据库,而是直接把书籍信息保存到了一个文本文件中。
阅读全文
相关推荐









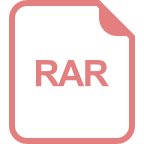







