用java写一个飞机大战游戏
时间: 2023-12-19 07:07:51 浏览: 28
好的,关于飞机大战游戏的开发,我可以为您提供一些建议和思路。首先,您可以使用 Java 语言,配合使用游戏引擎来开发这个游戏。您可以先搭建好游戏引擎的框架,然后再进行游戏场景的绘制和游戏角色的设计。在游戏角色的设计中,您可以为玩家设计不同的飞机,每个飞机都有特有的属性和技能。同时,您也需要为敌人设计不同的角色,包括飞机和BOSS,他们具有不同的攻击和防御方式,需要玩家使用不同的策略进行击败。最后,为了提高游戏的趣味性,您可以增加各种特效和音效,使游戏更加动感和有趣。希望这些建议可以为您带来一些灵感,祝您开发成功!
相关问题
请用java写一个飞机大战小游戏
好的,我会尽力用Java来写一个飞机大战小游戏。
首先,我们需要创建一个飞机类,来表示飞机的状态和行为。我们可以让飞机具有属性如位置、速度、生命值等,并且可以通过方法如移动、射击、受伤来控制飞机的行为。
接着,我们可以创建一个游戏类,来管理游戏的整体逻辑。游戏类应该包含一个飞机的实例,并且可以通过方法如开始游戏、暂停游戏、结束游戏来控制游戏的流程。此外,游戏类还应该包含方法来处理玩家的输入、更新游戏画面、检测碰撞等。
最后,我们需要创建一个主函数,来启动游戏。主函数中应该实例化一个游戏类,并调用它的开始游戏方法。
以下是一个简单的飞机大战小游戏的代码示例:
```
// 飞机类
class Plane {
// 属性:位置、速度、生命值
private int x;
private int y;
private int speed;
private int health;
// 构造方法:初始化飞机的状态
public Plane(int x, int y, int speed, int health) {
this.x = x;
this.y = y;
this.speed = speed;
this.health = health;
}
// 方法:移动飞机
public void move(int dx, int dy
用java写一个飞机大战的游戏
这里是一个基于Java Swing的简单飞机大战游戏的示例代码,仅供参考:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.ArrayList;
public class PlaneGame extends JFrame {
// 窗口大小
private static final int FRAME_WIDTH = 480;
private static final int FRAME_HEIGHT = 700;
// 飞机移动速度
private static final int PLANE_SPEED = 8;
// 子弹速度
private static final int BULLET_SPEED = 20;
// 敌机生成速度
private static final int ENEMY_PLANE_INTERVAL = 100;
// 敌机移动速度
private static final int ENEMY_PLANE_SPEED = 5;
// 分数
private int score = 0;
// 飞机图片
private Image planeImage;
// 子弹图片
private Image bulletImage;
// 敌机图片
private Image enemyPlaneImage;
// 爆炸图片
private Image boomImage;
// 飞机坐标
private int planeX;
private int planeY;
// 飞机状态
private boolean planeAlive = true;
// 子弹列表
private ArrayList<Bullet> bulletList = new ArrayList<>();
// 敌机列表
private ArrayList<EnemyPlane> enemyPlaneList = new ArrayList<>();
public PlaneGame() {
// 设置标题
setTitle("Plane Game");
// 设置窗口大小
setSize(FRAME_WIDTH, FRAME_HEIGHT);
// 设置窗口不可调整大小
setResizable(false);
// 居中显示窗口
setLocationRelativeTo(null);
// 关闭窗口时退出程序
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 添加键盘监听器
addKeyListener(new KeyAdapter() {
@Override
public void keyPressed(KeyEvent e) {
// 获取键盘按下的键
int keyCode = e.getKeyCode();
// 根据按键移动飞机
if (keyCode == KeyEvent.VK_LEFT) {
planeX -= PLANE_SPEED;
} else if (keyCode == KeyEvent.VK_RIGHT) {
planeX += PLANE_SPEED;
} else if (keyCode == KeyEvent.VK_UP) {
planeY -= PLANE_SPEED;
} else if (keyCode == KeyEvent.VK_DOWN) {
planeY += PLANE_SPEED;
} else if (keyCode == KeyEvent.VK_SPACE) {
// 发射子弹
Bullet bullet = new Bullet(planeX + planeImage.getWidth(null) / 2 - bulletImage.getWidth(null) / 2, planeY - bulletImage.getHeight(null));
bulletList.add(bullet);
}
}
});
// 加载图片
planeImage = new ImageIcon("plane.png").getImage();
bulletImage = new ImageIcon("bullet.png").getImage();
enemyPlaneImage = new ImageIcon("enemy_plane.png").getImage();
boomImage = new ImageIcon("boom.png").getImage();
// 初始化飞机坐标
planeX = FRAME_WIDTH / 2 - planeImage.getWidth(null) / 2;
planeY = FRAME_HEIGHT - planeImage.getHeight(null) - 50;
// 开始游戏循环
startGameLoop();
// 显示窗口
setVisible(true);
}
// 开始游戏循环
private void startGameLoop() {
// 创建游戏循环线程
Thread gameLoopThread = new Thread(() -> {
while (true) {
try {
// 生成敌机
if (Math.random() * ENEMY_PLANE_INTERVAL < 1) {
EnemyPlane enemyPlane = new EnemyPlane((int) (Math.random() * (FRAME_WIDTH - enemyPlaneImage.getWidth(null))), -enemyPlaneImage.getHeight(null));
enemyPlaneList.add(enemyPlane);
}
// 移动子弹
for (int i = bulletList.size() - 1; i >= 0; i--) {
Bullet bullet = bulletList.get(i);
bullet.y -= BULLET_SPEED;
// 判断子弹是否击中敌机
for (int j = enemyPlaneList.size() - 1; j >= 0; j--) {
EnemyPlane enemyPlane = enemyPlaneList.get(j);
if (bullet.x >= enemyPlane.x && bullet.x <= enemyPlane.x + enemyPlaneImage.getWidth(null) && bullet.y >= enemyPlane.y && bullet.y <= enemyPlane.y + enemyPlaneImage.getHeight(null)) {
// 击中敌机,移除子弹和敌机,并增加分数
bulletList.remove(i);
enemyPlaneList.remove(j);
score += 10;
break;
}
}
// 移除越界的子弹
if (bullet.y < -bulletImage.getHeight(null)) {
bulletList.remove(i);
}
}
// 移动敌机
for (int i = enemyPlaneList.size() - 1; i >= 0; i--) {
EnemyPlane enemyPlane = enemyPlaneList.get(i);
enemyPlane.y += ENEMY_PLANE_SPEED;
// 判断敌机是否撞击到飞机
if (enemyPlane.x + enemyPlaneImage.getWidth(null) >= planeX && enemyPlane.x <= planeX + planeImage.getWidth(null) && enemyPlane.y + enemyPlaneImage.getHeight(null) >= planeY && enemyPlane.y <= planeY + planeImage.getHeight(null)) {
// 撞击到飞机,游戏结束
planeAlive = false;
break;
}
// 移除越界的敌机
if (enemyPlane.y > FRAME_HEIGHT) {
enemyPlaneList.remove(i);
}
}
// 重绘窗口
repaint();
// 等待一段时间
Thread.sleep(30);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
});
// 启动游戏循环线程
gameLoopThread.start();
}
@Override
public void paint(Graphics g) {
// 创建双缓冲图像
Image doubleBufferImage = createImage(getWidth(), getHeight());
Graphics doubleBufferGraphics = doubleBufferImage.getGraphics();
// 绘制背景
doubleBufferGraphics.setColor(Color.WHITE);
doubleBufferGraphics.fillRect(0, 0, getWidth(), getHeight());
// 绘制分数
doubleBufferGraphics.setColor(Color.BLACK);
doubleBufferGraphics.setFont(new Font("微软雅黑", Font.BOLD, 20));
doubleBufferGraphics.drawString("分数:" + score, 20, 40);
// 绘制飞机
if (planeAlive) {
doubleBufferGraphics.drawImage(planeImage, planeX, planeY, null);
} else {
doubleBufferGraphics.drawImage(boomImage, planeX, planeY, null);
doubleBufferGraphics.setColor(Color.RED);
doubleBufferGraphics.setFont(new Font("微软雅黑", Font.BOLD, 40));
doubleBufferGraphics.drawString("游戏结束", FRAME_WIDTH / 2 - 100, FRAME_HEIGHT / 2);
}
// 绘制子弹
for (Bullet bullet : bulletList) {
doubleBufferGraphics.drawImage(bulletImage, bullet.x, bullet.y, null);
}
// 绘制敌机
for (EnemyPlane enemyPlane : enemyPlaneList) {
doubleBufferGraphics.drawImage(enemyPlaneImage, enemyPlane.x, enemyPlane.y, null);
}
// 绘制双缓冲图像到窗口
g.drawImage(doubleBufferImage, 0, 0, null);
}
// 子弹类
private static class Bullet {
int x;
int y;
public Bullet(int x, int y) {
this.x = x;
this.y = y;
}
}
// 敌机类
private static class EnemyPlane {
int x;
int y;
public EnemyPlane(int x, int y) {
this.x = x;
this.y = y;
}
}
public static void main(String[] args) {
new PlaneGame();
}
}
```
注意:这个示例代码只是一个简单的飞机大战游戏,还有很多需要完善和优化的地方,比如增加多种敌机、道具、关卡等。
相关推荐
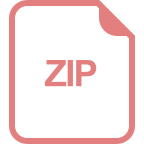

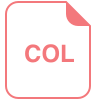
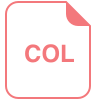
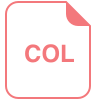
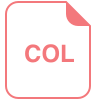







