js 实现excel表格拖拽排序
时间: 2023-07-20 11:08:39 浏览: 154
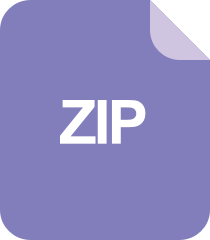
js实现的表格自由拖拽排序特效源码.zip
实现表格拖拽排序可以通过以下步骤:
1. 为表格中每个可排序的列头添加一个拖拽事件监听器,当用户按下鼠标左键时,开启拖拽状态。
2. 当用户移动鼠标时,获取当前鼠标位置和上一个位置之间的水平位移量,根据位移量计算出需要移动的列头和列的数量。
3. 将需要移动的列头和列的位置进行更新,同时更新表格中的数据。
4. 当用户释放鼠标左键时,结束拖拽状态。
以下是一个基于原生 JavaScript 实现的表格拖拽排序的示例代码:
```html
<table id="table">
<thead>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
</thead>
<tbody>
<tr>
<td>小明</td>
<td>18</td>
<td>男</td>
</tr>
<tr>
<td>小红</td>
<td>20</td>
<td>女</td>
</tr>
<tr>
<td>小华</td>
<td>19</td>
<td>男</td>
</tr>
</tbody>
</table>
```
```javascript
const table = document.getElementById('table');
let draggingColumn = null;
// 添加拖拽事件监听器
table.addEventListener('mousedown', e => {
const th = e.target.closest('th');
if (!th) return;
draggingColumn = th;
draggingColumn.classList.add('dragging');
});
// 监听拖拽过程事件
table.addEventListener('mousemove', e => {
if (!draggingColumn) return;
const x = e.pageX;
const ths = table.querySelectorAll('thead th');
const index = Array.from(ths).indexOf(draggingColumn);
const offsetX = x - draggingColumn.getBoundingClientRect().left;
const colspan = draggingColumn.colSpan || 1;
const next = ths[index + colspan];
const width = draggingColumn.offsetWidth + (next ? next.offsetWidth : 0);
const cols = table.querySelectorAll(`tr td:nth-child(${index + 1})`);
draggingColumn.style.left = `${offsetX}px`;
cols.forEach(col => {
col.style.left = `${offsetX}px`;
});
if (x > draggingColumn.getBoundingClientRect().right) {
draggingColumn.style.width = `${width}px`;
}
});
// 监听拖拽结束事件
table.addEventListener('mouseup', e => {
if (!draggingColumn) return;
draggingColumn.classList.remove('dragging');
draggingColumn.style.left = null;
draggingColumn.style.width = null;
const ths = table.querySelectorAll('thead th');
const index = Array.from(ths).indexOf(draggingColumn);
const next = ths[index + draggingColumn.colSpan];
const cols = table.querySelectorAll(`tr td:nth-child(${index + 1})`);
if (next) {
table.querySelectorAll(`tr td:nth-child(${index + 2})`).forEach(col => {
col.parentNode.insertBefore(col, next);
});
cols.forEach(col => {
col.parentNode.insertBefore(col, next);
});
} else {
const trs = table.querySelectorAll('tbody tr');
const arr = Array.from(trs).map(tr => Array.from(tr.children));
arr.forEach(row => {
const td = row.splice(index, draggingColumn.colSpan)[0];
row.push(td);
});
trs.forEach((tr, i) => {
tr.innerHTML = arr[i].map(td => td.outerHTML).join('');
});
}
draggingColumn = null;
});
```
在上面的代码中,我们为表格添加了三个事件监听器,分别是 mousedown、mousemove 和 mouseup。其中 mousedown 事件监听器用于启动拖拽状态,mousemove 事件监听器用于更新列头和列的位置,mouseup 事件监听器用于结束拖拽状态。
在拖拽过程中,我们使用了 translateX 属性来改变列头和列的位置,同时也使用了 left、width 属性和 insertBefore 方法来更新表格中的数据。
另外,我们还使用了一个 dragging 类来标记当前拖拽的列头,以便在样式上进行区分。
阅读全文
相关推荐
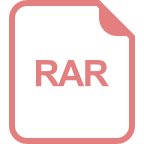
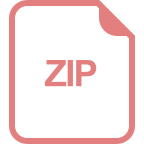
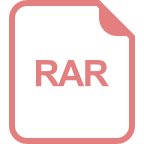
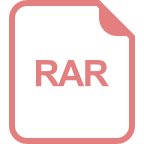
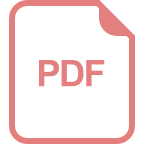
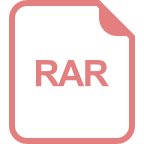
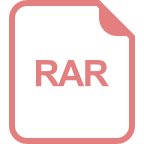
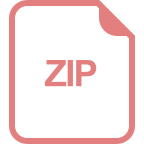
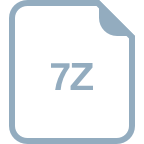
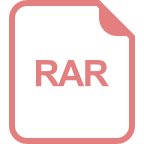

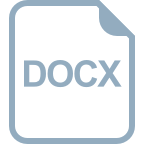
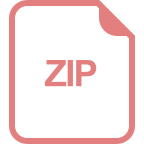
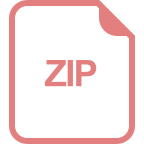
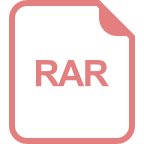
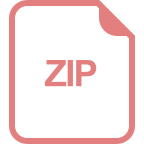
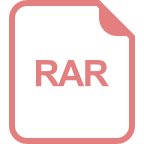