利用java小程序,利用drawline语句通过Bresenham算法绘制以(200,100)为圆心,100为半径的圆弧,左上部分圆弧为黑色,左下圆弧为青色,右下圆弧为红色,右上圆弧为灰色
时间: 2024-05-10 16:21:22 浏览: 120
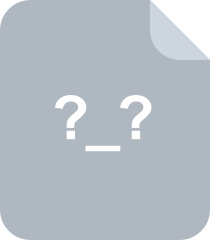
java实现Bresenham算法画圆

```java
import java.awt.Color;
import java.awt.Graphics;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class DrawArc extends JPanel {
public void paint(Graphics g) {
// 设置圆心
int centerX = 200;
int centerY = 100;
// 设置半径
int radius = 100;
// 左上部分圆弧(黑色)
g.setColor(Color.BLACK);
drawArc(g, centerX, centerY, radius, 135, 45);
// 左下部分圆弧(青色)
g.setColor(Color.CYAN);
drawArc(g, centerX, centerY, radius, 225, 45);
// 右下部分圆弧(红色)
g.setColor(Color.RED);
drawArc(g, centerX, centerY, radius, 315, 45);
// 右上部分圆弧(灰色)
g.setColor(Color.GRAY);
drawArc(g, centerX, centerY, radius, 45, 45);
}
// 以Bresenham算法绘制圆弧
private void drawArc(Graphics g, int centerX, int centerY, int radius, int startAngle, int arcAngle) {
int x = 0;
int y = radius;
int d = 3 - 2 * radius;
while (x <= y) {
drawPixel(g, centerX, centerY, x, y, startAngle, arcAngle);
if (d < 0) {
d = d + 4 * x + 6;
} else {
d = d + 4 * (x - y) + 10;
y--;
}
x++;
}
}
// 根据Bresenham算法绘制的像素点,绘制圆弧
private void drawPixel(Graphics g, int centerX, int centerY, int x, int y, int startAngle, int arcAngle) {
// 计算像素点的坐标
int[] xValues = {centerX + x, centerX - x, centerX + y, centerX - y};
int[] yValues = {centerY - y, centerY - y, centerY - x, centerY - x};
// 判断像素点是否在圆弧上,并绘制
for (int i = 0; i < 4; i++) {
if (isInArc(centerX, centerY, radius, xValues[i], yValues[i], startAngle, arcAngle)) {
g.drawLine(xValues[i], yValues[i], xValues[i], yValues[i]);
}
}
}
// 判断像素点是否在圆弧上
private boolean isInArc(int centerX, int centerY, int radius, int x, int y, int startAngle, int arcAngle) {
double angle = Math.atan2(y - centerY, x - centerX);
angle = Math.toDegrees(angle);
angle = angle < 0 ? angle + 360 : angle;
angle = angle - startAngle;
angle = angle < 0 ? angle + 360 : angle;
return angle <= arcAngle;
}
public static void main(String[] args) {
JFrame frame = new JFrame("DrawArc");
frame.setSize(400, 300);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.add(new DrawArc());
frame.setVisible(true);
}
}
```
阅读全文
相关推荐
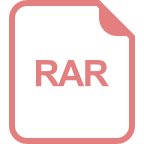
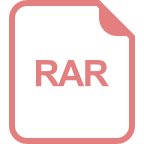
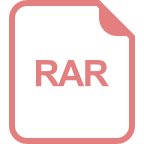
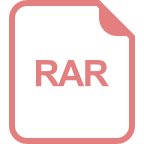
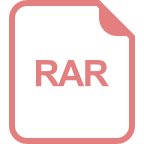
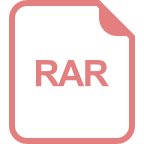
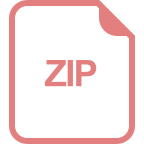
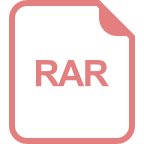
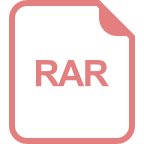
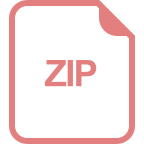
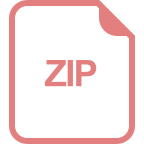
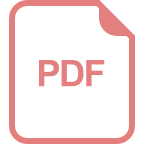
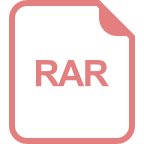
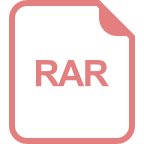
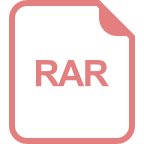
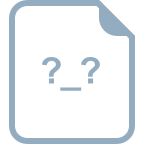