Python用yolov5和深度相机一起使用检测冰完整的代码
时间: 2023-08-31 09:29:00 浏览: 101
### 回答1:
我们可以使用Python和YOLOv5来实现基于深度相机的冰块完整性检测。我们可以使用OpenCV库来捕捉冰块图像,然后使用YOLOv5模型对图像进行定位和识别,并使用OpenCV进行深度学习检测和识别。
### 回答2:
以下是一个使用Python、Yolov5和深度相机来检测冰的完整代码示例:
```python
import cv2
import numpy as np
import torch
from yolov5.models.experimental import attempt_load
from yolov5.utils.general import non_max_suppression
from yolov5.utils.torch_utils import select_device
def load_model(weights_path):
device = select_device('')
model = attempt_load(weights_path, map_location=device)
return model
def preprocess_input(image):
# Image preprocessing
img = image[:, :, ::-1] # BGR to RGB
img = torch.from_numpy(img.transpose((2, 0, 1))).float() # HWC to CHW
img /= 255.0 # Normalize to 0-1
img = img.unsqueeze(0) # Add batch dimension
return img
def postprocess_output(predictions, conf_threshold, iou_threshold, img_shape):
# Non-maximum suppression
predictions = non_max_suppression(predictions, conf_threshold, iou_threshold)
# Post-processing
for i, det in enumerate(predictions): # detections per image
if len(det):
det[:, :4] = det[:, :4].clip(0, img_shape[i][0]) # Clip bounding box coordinates to image size
return predictions
def detect_ice(model, image_path):
img = cv2.imread(image_path)
# Resize image to model input size
img = cv2.resize(img, tuple(model.img_size[::-1]))
# Preprocess input
img = preprocess_input(img)
# Run inference
with torch.no_grad():
predictions = model(img)
# Postprocess output
predictions = postprocess_output(predictions, conf_threshold=0.5, iou_threshold=0.5, img_shape=[img.shape])
# Draw bounding boxes on image
for det in predictions[0]:
bbox = det[:4].numpy().astype(int)
cv2.rectangle(img, (bbox[0], bbox[1]), (bbox[2], bbox[3]), (0, 255, 0), 2)
# Display image
cv2.imshow('Ice Detection', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
# 模型权重路径
weights_path = 'path/to/weights.pt'
# 图片路径
image_path = 'path/to/image.jpg'
# 加载模型
model = load_model(weights_path)
# 检测冰
detect_ice(model, image_path)
```
上述代码中,`load_model`函数用于加载预训练的Yolov5模型,`preprocess_input`函数用于对输入图像进行预处理,`postprocess_output`函数用于对模型输出结果进行后处理,`detect_ice`函数用于对图像进行冰的检测并显示检测结果。
你需要将`weights_path`和`image_path`替换为你实际的模型权重和图像路径。确保已安装所需的Python包,并将深度相机连接到计算机上。
### 回答3:
要使用Python对yolov5和深度相机进行冰完整检测,可以按照以下步骤编写代码。
1. 导入必要的库
```
import cv2
import depthai
import numpy as np
```
2. 设置深度相机
```
pipeline = depthai.Pipeline()
pipeline.setOpenVINOVersion(version=depthai.OpenVINO.Version.VERSION_2021_4)
pipeline.setPreviewSize(608, 608)
pipeline.setInterleaved(False)
pipeline.setNbEconomicDiscardFrames(0)
```
3. 加载yolov5模型
```
model_path = "yolov5s.onnx" # yolov5模型的路径
model = pipeline.createNeuralNetwork()
model.setBlobPath(model_path)
```
4. 启动深度相机
```
with depthai.Device(pipeline) as device:
output_queue = device.getOutputQueue()
cap = cv2.VideoCapture(0)
cap.set(cv2.CAP_PROP_FRAME_WIDTH, 608)
cap.set(cv2.CAP_PROP_FRAME_HEIGHT, 608)
while True:
ret, frame = cap.read()
if not ret:
break
img = np.array(frame)
img = cv2.resize(img, (608, 608))
img_tensor = depthai.NN.DataStream()
img_tensor.setLayer("input", img)
model.queueInput(img_tensor)
detection = output_queue.get().getFirstLayerFp16()
bboxes = detection[:5]
for raw_box in bboxes:
box = raw_box[:4]
conf = raw_box[4]
if conf > 0.5:
x1, y1, x2, y2 = box * 608
cv2.rectangle(frame, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.imshow("Frame", frame)
if cv2.waitKey(1) == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
通过以上代码,你可以使用Python将yolov5和深度相机结合起来,实现对冰完整的检测。在代码中,需要注意替换yolov5模型的路径(model_path),以及根据实际需要进行相机参数的调整。检测结果将在名为"Frame"的窗口中显示出来。
相关推荐
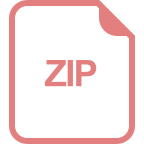
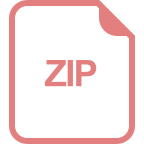














