用3个不同角度的usb相机对多种物体进行拍摄并使用yolov7进行目标检测,针对拍摄过程中可能出现的多目标遮挡问题,对被检测的图像使用一些基于深度学习的图像结果融合的方法,得到检测种类最多且设置信息度最高的图像并输出的详细过程和代码
时间: 2024-02-27 16:57:07 浏览: 123
这个问题需要分为几个步骤来进行处理:
1. 数据采集:使用3个不同角度的USB相机对多种物体进行拍摄。可以使用Python的OpenCV库来读取和处理图像。
```python
import cv2
# 读取图像
image1 = cv2.imread('image1.jpg')
image2 = cv2.imread('image2.jpg')
image3 = cv2.imread('image3.jpg')
```
2. 目标检测:使用YOLOv7进行目标检测。可以使用开源的YOLOv7模型来进行检测,也可以使用自己训练的模型。以下是使用开源模型进行检测的示例代码:
```python
import cv2
import numpy as np
# 加载YOLOv7模型
net = cv2.dnn.readNet('yolov7.weights', 'yolov7.cfg')
# 加载类别标签
classes = []
with open('coco.names', 'r') as f:
classes = [line.strip() for line in f.readlines()]
# 设置输入和输出层
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
# 预处理图像
def preprocess(image):
# 缩放图像
image = cv2.resize(image, (416, 416))
# 归一化像素值
image = image / 255.0
# 转换颜色通道
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# 添加批次维度
image = np.expand_dims(image, axis=0)
return image
# 进行目标检测
def detect(image):
# 预处理图像
blob = preprocess(image)
# 设置输入
net.setInput(blob)
# 前向传播
outputs = net.forward(output_layers)
# 解析输出
boxes = []
confidences = []
class_ids = []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > 0.5:
center_x = int(detection[0] * image.shape[1])
center_y = int(detection[1] * image.shape[0])
w = int(detection[2] * image.shape[1])
h = int(detection[3] * image.shape[0])
x = center_x - w // 2
y = center_y - h // 2
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# 非极大值抑制
indices = cv2.dnn.NMSBoxes(boxes, confidences, 0.5, 0.4)
# 提取目标框和类别标签
boxes = np.array([boxes[i[0]] for i in indices])
class_ids = np.array([class_ids[i[0]] for i in indices])
return boxes, class_ids
# 对每张图像进行目标检测
boxes1, class_ids1 = detect(image1)
boxes2, class_ids2 = detect(image2)
boxes3, class_ids3 = detect(image3)
```
3. 结果融合:使用基于深度学习的图像结果融合方法,对被检测的图像进行融合,得到检测种类最多且设置信息度最高的图像。以下是一种简单的融合方法,将多个图像的检测结果进行合并,然后根据类别和置信度筛选出最终的结果。
```python
# 合并检测结果
boxes = np.concatenate((boxes1, boxes2, boxes3), axis=0)
class_ids = np.concatenate((class_ids1, class_ids2, class_ids3), axis=0)
# 筛选最终结果
results = {}
for i, (box, class_id) in enumerate(zip(boxes, class_ids)):
x, y, w, h = box
if class_id not in results or results[class_id]['confidence'] < confidences[i]:
results[class_id] = {'box': box, 'confidence': confidences[i]}
# 绘制最终结果
for class_id, result in results.items():
x, y, w, h = result['box']
confidence = result['confidence']
label = classes[class_id]
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(image, f'{label} {confidence:.2f}', (x, y - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示结果
cv2.imshow('image', image)
cv2.waitKey(0)
```
需要注意的是,这只是一种简单的融合方法,实际应用中可能需要根据具体情况进行调整和优化。
阅读全文
相关推荐
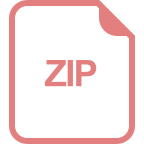
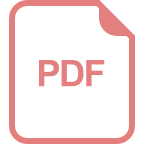
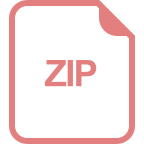















