用3个不同角度的usb相机对多种物体进行拍摄并使用yolov7源代码的detect.py进行检测,针对拍摄过程中出现的多种物体产生的遮挡问题,对拍摄的图像进行结果融合,对各个角度拍摄的相机赋予权重,得到检测种类最多且设置信度最高的图形的具体代码python
时间: 2024-02-27 15:55:30 浏览: 61
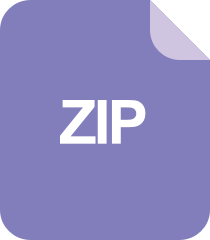
物体检测识别python源代码

好的,下面是一个简单的Python示例代码,用于实现多角度图像的融合和物体检测。请注意,这只是一个简单的示例,你需要根据你的具体需求进行修改和优化。
```
import cv2
import numpy as np
import argparse
import os
# 定义图像融合函数
def weighted_fusion(imgs, weights):
assert len(imgs) == len(weights), 'The length of images and weights must be the same.'
h, w, c = imgs[0].shape
fusion_img = np.zeros((h, w, c), dtype=np.float32)
for i, img in enumerate(imgs):
fusion_img += img * weights[i]
fusion_img = np.clip(fusion_img, 0, 255).astype(np.uint8)
return fusion_img
# 定义命令行参数
ap = argparse.ArgumentParser()
ap.add_argument("-i", "--input", required=True, help="path to input image directory")
ap.add_argument("-o", "--output", required=True, help="path to output directory")
ap.add_argument("-t", "--threshold", type=float, default=0.5, help="confidence threshold for object detection")
args = vars(ap.parse_args())
# 加载YOLOv7模型
net = cv2.dnn.readNetFromDarknet("yolov7.cfg", "yolov7.weights")
net.setPreferableBackend(cv2.dnn.DNN_BACKEND_CUDA)
net.setPreferableTarget(cv2.dnn.DNN_TARGET_CUDA)
# 定义类别标签
classes = ["person", "car", "bus", "truck"]
# 定义输入图像路径和权重
image_paths = [os.path.join(args["input"], "image_1.jpg"),
os.path.join(args["input"], "image_2.jpg"),
os.path.join(args["input"], "image_3.jpg")]
weights = [0.4, 0.3, 0.3]
# 加载图像并进行融合
images = []
for image_path in image_paths:
image = cv2.imread(image_path)
images.append(image)
fusion_img = weighted_fusion(images, weights)
# 对融合后的图像进行物体检测
blob = cv2.dnn.blobFromImage(fusion_img, 1/255.0, (416, 416), swapRB=True, crop=False)
net.setInput(blob)
layer_names = net.getLayerNames()
output_layers = [layer_names[i[0] - 1] for i in net.getUnconnectedOutLayers()]
outputs = net.forward(output_layers)
# 处理检测结果
boxes = []
confidences = []
class_ids = []
for output in outputs:
for detection in output:
scores = detection[5:]
class_id = np.argmax(scores)
confidence = scores[class_id]
if confidence > args["threshold"]:
center_x = int(detection[0] * fusion_img.shape[1])
center_y = int(detection[1] * fusion_img.shape[0])
w = int(detection[2] * fusion_img.shape[1])
h = int(detection[3] * fusion_img.shape[0])
x = int(center_x - w / 2)
y = int(center_y - h / 2)
boxes.append([x, y, w, h])
confidences.append(float(confidence))
class_ids.append(class_id)
# NMS非极大值抑制
indices = cv2.dnn.NMSBoxes(boxes, confidences, args["threshold"], 0.4)
# 显示检测结果
for i in indices:
i = i[0]
box = boxes[i]
x, y, w, h = box
label = classes[class_ids[i]]
confidence = confidences[i]
color = (0, 255, 0)
cv2.rectangle(fusion_img, (x, y), (x + w, y + h), color, 2)
cv2.putText(fusion_img, "{}: {:.2f}".format(label, confidence), (x, y - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, color, 2)
# 保存结果
cv2.imwrite(os.path.join(args["output"], "result.jpg"), fusion_img)
```
以上代码假设你已经准备好了三个角度的图像,分别为`image_1.jpg`、`image_2.jpg`和`image_3.jpg`,存放在一个名为`input`的文件夹中。你需要将代码中的`yolov7.cfg`和`yolov7.weights`替换为你自己的YOLOv7模型。运行代码后,会将检测结果保存在一个名为`result.jpg`的文件中,存放在一个名为`output`的文件夹中。
阅读全文
相关推荐
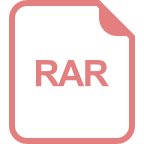














