用python写一个贪吃蛇小游戏
时间: 2023-12-07 20:55:02 浏览: 54
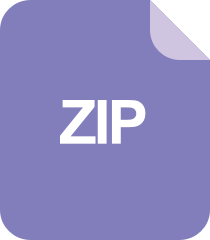
Python编写一个贪吃蛇游戏
好的,下面是用 Python 实现贪吃蛇小游戏的代码示例:
```python
import pygame
import random
pygame.init()
# 游戏窗口大小和标题
window_width = 500
window_height = 500
game_window = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption('贪吃蛇小游戏')
# 游戏颜色
white = (255, 255, 255)
black = (0, 0, 0)
red = (213, 50, 80)
green = (0, 255, 0)
blue = (50, 153, 213)
# 定义蛇的大小
snake_size = 10
# 定义游戏时钟
clock = pygame.time.Clock()
# 定义字体
font_style = pygame.font.SysFont(None, 30)
def message(msg, color):
"""
在屏幕上显示消息的函数
"""
mesg = font_style.render(msg, True, color)
game_window.blit(mesg, [window_width / 6, window_height / 3])
def draw_snake(snake_size, snake_list):
"""
在游戏窗口上画蛇的函数
"""
for x in snake_list:
pygame.draw.rect(game_window, black, [x[0], x[1], snake_size, snake_size])
def game_loop():
"""
游戏主循环
"""
game_over = False
game_close = False
# 初始化贪吃蛇的位置和长度
x1 = window_width / 2
y1 = window_height / 2
x1_change = 0
y1_change = 0
snake_List = []
Length_of_snake = 1
# 随机生成食物的位置
foodx = round(random.randrange(0, window_width - snake_size) / 10.0) * 10.0
foody = round(random.randrange(0, window_height - snake_size) / 10.0) * 10.0
while not game_over:
while game_close == True:
game_window.fill(white)
message("你输了!按 Q-退出,C-重新开始", red)
pygame.display.update()
for event in pygame.event.get():
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
game_over = True
game_close = False
if event.key == pygame.K_c:
game_loop()
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
x1_change = -snake_size
y1_change = 0
elif event.key == pygame.K_RIGHT:
x1_change = snake_size
y1_change = 0
elif event.key == pygame.K_UP:
y1_change = -snake_size
x1_change = 0
elif event.key == pygame.K_DOWN:
y1_change = snake_size
x1_change = 0
# 判断蛇是否越界
if x1 >= window_width or x1 < 0 or y1 >= window_height or y1 < 0:
game_close = True
# 移动蛇头的位置
x1 += x1_change
y1 += y1_change
# 在游戏窗口上画蛇和食物
game_window.fill(white)
pygame.draw.rect(game_window, green, [foodx, foody, snake_size, snake_size])
snake_Head = []
snake_Head.append(x1)
snake_Head.append(y1)
snake_List.append(snake_Head)
if len(snake_List) > Length_of_snake:
del snake_List[0]
for x in snake_List[:-1]:
if x == snake_Head:
game_close = True
draw_snake(snake_size, snake_List)
pygame.display.update()
# 当蛇头吃到食物时,贪吃蛇的长度加 1
if x1 == foodx and y1 == foody:
foodx = round(random.randrange(0, window_width - snake_size) / 10.0) * 10.0
foody = round(random.randrange(0, window_height - snake_size) / 10.0) * 10.0
Length_of_snake += 1
clock.tick(10)
pygame.quit()
quit()
game_loop()
```
这个代码实现了一个简单的贪吃蛇小游戏,玩家通过上下左右键控制蛇的移动,蛇头碰到边界或自己的身体时游戏结束,蛇头吃到食物时贪吃蛇的长度加 1。希望你玩得愉快!
阅读全文
相关推荐




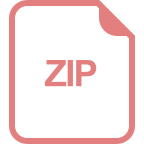
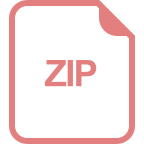
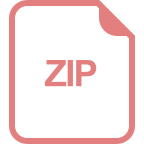
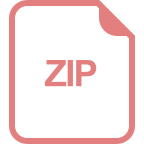