python如何获取图像上黑色正方形四个角点坐标
时间: 2023-06-20 14:03:35 浏览: 143
可以使用Python中的OpenCV库来实现。以下是一个简单的例子:
1. 首先,读取图像并将其转换为灰度图像:
``` python
import cv2
img = cv2.imread('image.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 然后,使用Canny算子进行边缘检测:
``` python
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
```
3. 接下来,使用霍夫变换检测图像中的直线:
``` python
lines = cv2.HoughLines(edges, 1, np.pi/180, 200)
```
4. 对于每个检测到的直线,计算其与图像边界的交点:
``` python
for line in lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
```
5. 最后,使用这些交点计算出正方形的四个角点坐标:
``` python
corners = cv2.goodFeaturesToTrack(gray, 4, 0.01, 10)
corners = np.int0(corners)
for corner in corners:
x, y = corner.ravel()
cv2.circle(img, (x, y), 3, (0, 255, 0), -1)
```
完整代码如下:
``` python
import cv2
import numpy as np
img = cv2.imread('image.png')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray, 50, 150, apertureSize=3)
lines = cv2.HoughLines(edges, 1, np.pi/180, 200)
for line in lines:
rho, theta = line[0]
a = np.cos(theta)
b = np.sin(theta)
x0 = a * rho
y0 = b * rho
x1 = int(x0 + 1000 * (-b))
y1 = int(y0 + 1000 * (a))
x2 = int(x0 - 1000 * (-b))
y2 = int(y0 - 1000 * (a))
cv2.line(img, (x1, y1), (x2, y2), (0, 0, 255), 2)
corners = cv2.goodFeaturesToTrack(gray, 4, 0.01, 10)
corners = np.int0(corners)
for corner in corners:
x, y = corner.ravel()
cv2.circle(img, (x, y), 3, (0, 255, 0), -1)
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐



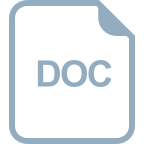










