1、阅读下面程序,叙述其功能 import java.io.FileReader; import java.io.IOException; public class FileViewer { /** Defines the entry point of the program. */ public static void main(String[] args) { System.out.println("Please enter the file path:"); try { String fileName = ""; while(true) { int readByte = System.in.read(); if(readByte == -1 || readByte == '\n') break; fileName += (char) readByte; } // Reads the file and prints it to the System.out stream. char[] buffer = new char[20]; FileReader reader = new FileReader(fileName); while(true) { int length = reader.read(buffer); if(length < 0) // Reads a long as there is more data. break; String text = new String(buffer, 0, length); System.out.print(text); } } catch (IOException e) { e.printStackTrace(); } } } [基本要求] 写出本题程序的功能。
时间: 2024-04-03 11:32:06 浏览: 37
这个程序的功能是读取用户输入的文件路径,然后读取该路径下的文件内容,并将文件内容输出到控制台。程序通过循环读取用户输入的字符,当读取的字符为换行符或者文件末尾时,停止读取。接着,程序使用FileReader类读取指定路径下的文件内容,每次读取20个字符,并将读取的字符存储在一个字符数组中,然后将数组中的内容转换为字符串输出到控制台。如果读取到文件末尾,程序停止读取。如果读取文件过程中发生了IO异常,程序将打印出异常信息。
相关问题
1、阅读下面程序,叙述其功能 import java.io.FileReader; import java.io.IOException; public class FileViewer { /** Defines the entry point of the program. */ public static void main(String[] args) { System.out.println("Please enter the file path:"); try { String fileName = ""; while(true) { int readByte = System.in.read(); if(readByte == -1 || readByte == '\n') break; fileName += (char) readByte; } // Reads the file and prints it to the System.out stream. char[] buffer = new char[20]; FileReader reader = new FileReader(fileName); while(true) { int length = reader.read(buffer); if(length < 0) // Reads a long as there is more data. break; String text = new String(buffer, 0, length); System.out.print(text); } } catch (IOException e) { e.printStackTrace(); } } }
这段程序的功能是接收用户输入的文件路径,然后读取该文件并将其内容输出到标准输出流(System.out)中。程序使用 FileReader 类来读取文件。程序首先提示用户输入文件路径,然后通过 System.in.read() 方法一次读取一个字节,直到读取到回车符为止。然后程序使用 FileReader 类读取文件,并将每次读取到的内容存储到一个字符数组中,最后将字符数组转换为字符串并输出。如果读取到文件末尾,程序会结束。如果读取文件时发生了 IOException 异常,程序会打印出异常信息。
请编写一个Java程序实现如下图所示的“文本文件阅读器”窗体(TextFileViewer): 窗体的主要区域是一个JTextArea对象,放置在一个JScrollPane中。窗体底部由三个组件构成,分别是标签、文本框(设置为只读,即不可编辑)和一个按钮。 请注意各个组件的布局关系并合理使用布局管理器。
好的,以下是您需要的Java程序(TextFileViewer):
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
public class TextFileViewer extends JFrame implements ActionListener {
private JTextArea textArea;
private JTextField filePathField;
public TextFileViewer() {
super("文本文件阅读器");
// 创建JTextArea对象并放置在JScrollPane中
textArea = new JTextArea();
JScrollPane scrollPane = new JScrollPane(textArea);
// 创建标签、文本框和按钮
JLabel filePathLabel = new JLabel("文件路径:");
filePathField = new JTextField(20);
filePathField.setEditable(false);
JButton openButton = new JButton("打开文件");
openButton.addActionListener(this);
// 创建底部Panel并加入组件
JPanel bottomPanel = new JPanel(new FlowLayout(FlowLayout.LEFT));
bottomPanel.add(filePathLabel);
bottomPanel.add(filePathField);
bottomPanel.add(openButton);
// 将JScrollPane和底部Panel加入到窗体中
getContentPane().add(scrollPane, BorderLayout.CENTER);
getContentPane().add(bottomPanel, BorderLayout.SOUTH);
// 设置窗体大小、位置和可见性
setSize(600, 400);
setLocationRelativeTo(null);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equals("打开文件")) {
JFileChooser fileChooser = new JFileChooser();
int result = fileChooser.showOpenDialog(this);
if (result == JFileChooser.APPROVE_OPTION) {
File selectedFile = fileChooser.getSelectedFile();
filePathField.setText(selectedFile.getAbsolutePath());
try {
BufferedReader reader = new BufferedReader(new FileReader(selectedFile));
String line;
textArea.setText("");
while ((line = reader.readLine()) != null) {
textArea.append(line + "\n");
}
reader.close();
} catch (IOException ex) {
JOptionPane.showMessageDialog(this, "文件读取错误!");
}
}
}
}
public static void main(String[] args) {
TextFileViewer viewer = new TextFileViewer();
viewer.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
```
程序的实现过程如下:
1. 首先,我们创建了一个 `JTextArea` 对象,然后将其放置在一个 `JScrollPane` 中,以便于用户可以滚动查看文本内容。
2. 接着,我们创建了一个标签、一个文本框和一个按钮,并将它们放置在一个底部的 `JPanel` 中,使用 `FlowLayout` 布局管理器以便于它们可以排列在一行中。
3. 然后,我们将 `JScrollPane` 和底部的 `JPanel` 加入到窗体中,使用 `BorderLayout` 布局管理器,以便于它们可以占据窗体的中间和底部区域。
4. 在按钮的 `actionPerformed` 方法中,我们创建了一个 `JFileChooser` 对话框,让用户选择要打开的文件。如果用户选择了一个文件,则读取这个文件的内容,并将其显示在 `JTextArea` 中。
5. 最后,我们创建了一个 `main` 方法来启动程序,并设置了窗体的关闭操作为 `EXIT_ON_CLOSE`。
希望这个程序能够帮助您解决问题!
阅读全文
相关推荐
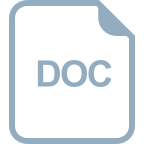
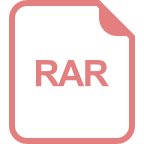
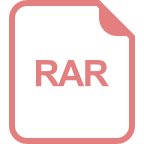
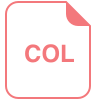
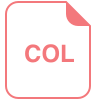
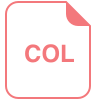
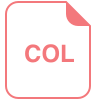



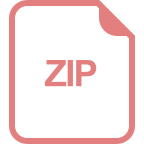