用python写一个推箱子游戏
时间: 2023-06-04 22:07:30 浏览: 150
好的,我会用中文来帮助您编写一个推箱子游戏。
首先,我们需要定义游戏的地图。地图可以用一个二维数组来表示,其中 0 表示可以走的路,1 表示墙壁,2 表示箱子,3 表示目标位置。例如,我们可以定义一个 3x3 的地图,其中有一个箱子和一个目标位置:
```
map = [
[0, 0, 1],
[0, 2, 3],
[0, 0, 0]
]
```
接下来,我们需要定义玩家的位置。我们可以使用一个元组来表示玩家的位置,例如 (1, 1) 表示玩家在地图的第二行第二列。
接下来,我们可以使用循环来接受玩家的输入,并根据输入来移动玩家的位置。我们可以使用 Python 的内置函数 input() 来获取玩家的输入。
例如,我们可以使用以下代码来获取玩家的输入并移动玩家的位置:
```
while True:
# 获取玩家的输入
move = input("请输入你要移动的方向(w, a, s, d): ")
# 根据输入移动玩家的位置
if move == 'w':
player_row -= 1
elif move == 'a':
player_col -= 1
elif move == 's':
player_row += 1
elif move == 'd':
player_col += 1
相关问题
使用python 写一个推箱子游戏代码
以下是一个简单的推箱子游戏的 Python 代码示例,你可以根据你的需求进行修改和扩展:
```python
# 定义游戏地图
game_map = [
['#', '#', '#', '#', '#', '#', '#', '#', '#', '#', '#', '#'],
['#', '.', '.', '.', '#', '.', '.', '.', '.', '.', '.', '#'],
['#', '.', '.', '.', '#', '.', '.', '.', '.', '.', '.', '#'],
['#', '.', '.', '.', '.', '.', '#', '#', '#', '.', '.', '#'],
['#', '.', '.', '.', '.', '.', '#', 'X', '.', '.', '.', '#'],
['#', '.', '.', '.', '.', '.', '.', 'X', '.', '.', '.', '#'],
['#', '.', '.', '.', '.', '.', '.', '.', '.', '.', '.', '#'],
['#', '.', '.', '.', '.', '.', '.', '.', '.', '.', '.', '#'],
['#', '.', '.', '.', '.', '.', '.', '.', '.', '.', '.', '#'],
['#', '.', '.', '.', '.', '.', '.', '.', '.', '.', '.', '#'],
['#', '.', '.', '.', '.', '.', '.', '.', '.', '.', '.', '#'],
['#', '#', '#', '#', '#', '#', '#', '#', '#', '#', '#', '#'],
]
# 定义玩家初始位置
player_pos = [5, 7]
# 定义推箱子游戏主循环
while True:
# 打印游戏地图
for row in game_map:
print(' '.join(row))
# 检查是否达到胜利条件
win = True
for row in game_map:
if 'X' in row:
win = False
break
if win:
print('Congratulations, you win!')
break
# 获取玩家输入
move = input('Enter move (up/down/left/right): ')
# 根据玩家输入更新玩家位置和地图中的箱子位置
if move == 'up':
if game_map[player_pos[0] - 1][player_pos[1]] == '#':
continue
elif game_map[player_pos[0] - 1][player_pos[1]] == 'X':
if game_map[player_pos[0] - 2][player_pos[1]] == '#' or game_map[player_pos[0] - 2][player_pos[1]] == 'X':
continue
else:
game_map[player_pos[0] - 2][player_pos[1]] = 'X'
game_map[player_pos[0] - 1][player_pos[1]] = '.'
player_pos[0] -= 1
else:
player_pos[0] -= 1
elif move == 'down':
if game_map[player_pos[0] + 1][player_pos[1]] == '#':
continue
elif game_map[player_pos[0] + 1][player_pos[1]] == 'X':
if game_map[player_pos[0] + 2][player_pos[1]] == '#' or game_map[player_pos[0] + 2][player_pos[1]] == 'X':
continue
else:
game_map[player_pos[0] + 2][player_pos[1]] = 'X'
game_map[player_pos[0] + 1][player_pos[1]] = '.'
player_pos[0] += 1
else:
player_pos[0] += 1
elif move == 'left':
if game_map[player_pos[0]][player_pos[1] - 1] == '#':
continue
elif game_map[player_pos[0]][player_pos[1] - 1] == 'X':
if game_map[player_pos[0]][player_pos[1] - 2] == '#' or game_map[player_pos[0]][player_pos[1] - 2] == 'X':
continue
else:
game_map[player_pos[0]][player_pos[1] - 2] = 'X'
game_map[player_pos[0]][player_pos[1] - 1] = '.'
player_pos[1] -= 1
else:
player_pos[1] -= 1
elif move == 'right':
if game_map[player_pos[0]][player_pos[1] + 1] == '#':
continue
elif game_map[player_pos[0]][player_pos[1] + 1] == 'X':
if game_map[player_pos[0]][player_pos[1] + 2] == '#' or game_map[player_pos[0]][player_pos[1] + 2] == 'X':
continue
else:
game_map[player_pos[0]][player_pos[1] + 2] = 'X'
game_map[player_pos[0]][player_pos[1] + 1] = '.'
player_pos[1] += 1
else:
player_pos[1] += 1
```
在这个示例代码中,用 # 表示墙,. 表示空地,X 表示箱子,玩家用箭头表示。玩家可以通过输入方向键来移动,如果玩家的移动能够推动箱子,那么箱子也会移动。如果所有箱子都被推到正确的位置,玩家就胜利了。
使用python写一个推箱子游戏的函数
好的,下面是一个简单的推箱子游戏的 Python 函数实现:
```python
def push_box_game(map):
ROWS = len(map)
COLS = len(map[0])
player_pos = None
boxes = []
goals = []
for r in range(ROWS):
for c in range(COLS):
if map[r][c] == "P":
player_pos = (r, c)
map[r][c] = " "
elif map[r][c] == "B":
boxes.append((r, c))
map[r][c] = " "
elif map[r][c] == "G":
goals.append((r, c))
map[r][c] = " "
def check_win():
for box in boxes:
if box not in goals:
return False
return True
def move_player(dx, dy):
nonlocal player_pos
x, y = player_pos
new_x, new_y = x + dx, y + dy
if not (0 <= new_x < ROWS and 0 <= new_y < COLS):
return False
if map[new_x][new_y] == " ":
player_pos = (new_x, new_y)
return True
for box in boxes:
bx, by = box
if bx == new_x and by == new_y:
new_bx, new_by = bx + dx, by + dy
if not (0 <= new_bx < ROWS and 0 <= new_by < COLS):
return False
if map[new_bx][new_by] == " " and (new_bx, new_by) not in boxes:
box = (new_bx, new_by)
boxes.remove((bx, by))
boxes.append(box)
player_pos = (new_x, new_y)
return True
return False
while not check_win():
print_map(map, player_pos, boxes, goals)
move = input("Enter move (u/d/l/r): ")
dx, dy = 0, 0
if move == "u":
dx, dy = -1, 0
elif move == "d":
dx, dy = 1, 0
elif move == "l":
dx, dy = 0, -1
elif move == "r":
dx, dy = 0, 1
if dx != 0 or dy != 0:
if move_player(dx, dy):
clear_output()
else:
print("Invalid move!")
time.sleep(1)
clear_output()
print_map(map, player_pos, boxes, goals)
print("You won!")
def print_map(map, player_pos, boxes, goals):
ROWS = len(map)
COLS = len(map[0])
for r in range(ROWS):
for c in range(COLS):
if (r, c) == player_pos:
print("P", end="")
elif (r, c) in boxes:
print("B", end="")
elif (r, c) in goals:
print("G", end="")
else:
print(map[r][c], end="")
print()
map = [
["#", "#", "#", "#", "#", "#", "#", "#", "#"],
["#", " ", " ", " ", "B", " ", " ", " ", "#"],
["#", " ", " ", " ", " ", " ", " ", " ", "#"],
["#", " ", " ", " ", " ", " ", " ", " ", "#"],
["#", " ", " ", " ", " ", " ", " ", " ", "#"],
["#", " ", " ", " ", " ", " ", " ", " ", "#"],
["#", " ", " ", " ", " ", " ", "G", " ", "#"],
["#", " ", " ", " ", " ", " ", " ", " ", "#"],
["#", "#", "#", "#", "#", "#", "#", "#", "#"]
]
push_box_game(map)
```
这个函数接受一个地图作为参数,地图是一个二维数组,其中 # 表示墙,空格表示空格子,P 表示玩家,B 表示箱子,G 表示目标点。
函数使用了 Python 的闭包来实现内部函数的访问和修改外部变量。函数中的 `check_win` 函数用于判断游戏是否胜利,`move_player` 函数用于移动玩家和箱子,`print_map` 函数用于输出当前地图状态。函数执行时会进入一个循环,不断等待玩家输入移动指令,然后根据输入来移动玩家和箱子。当所有的箱子都被推到目标点上时,函数会输出胜利信息并结束游戏。
注意:这个函数使用了 `clear_output` 和 `time.sleep` 函数来清除屏幕和暂停一段时间,这些函数只在 Jupyter Notebook 等交互式环境中可用,如果在命令行中运行需要另外实现清屏和暂停功能。
阅读全文
相关推荐








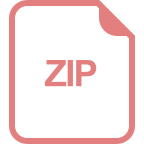