用python写一个推箱子的小游戏
时间: 2023-08-04 19:32:33 浏览: 117
好的,下面是一个简单的推箱子小游戏的Python代码实现。在游戏中,玩家需要移动箱子抵达终点。玩家可以使用键盘上的箭头键来控制人物移动,并且只有在箱子的前面没有障碍物时才能推动箱子。
```python
# 导入必要的模块
import pygame
from pygame.locals import *
# 初始化Pygame
pygame.init()
# 设置游戏窗口大小
screen_width = 640
screen_height = 480
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("推箱子小游戏")
# 加载游戏素材
wall_image = pygame.image.load("wall.png").convert()
box_image = pygame.image.load("box.png").convert()
destination_image = pygame.image.load("destination.png").convert()
player_image = pygame.image.load("player.png").convert()
# 定义游戏地图
game_map = [
"WWWWWWWWWWWWWWWWWWWW",
"W W",
"W W",
"W D W",
"W W",
"W W",
"W B W",
"W W",
"W W",
"W P W",
"W W",
"W W",
"WWWWWWWWWWWWWWWWWWWW",
]
# 定义游戏中的角色
class Player:
def __init__(self, x, y):
self.x = x
self.y = y
def move(self, dx, dy):
if game_map[self.y+dy][self.x+dx] != "W":
self.x += dx
self.y += dy
def draw(self):
screen.blit(player_image, (self.x*32, self.y*32))
class Box:
def __init__(self, x, y):
self.x = x
self.y = y
def draw(self):
screen.blit(box_image, (self.x*32, self.y*32))
class Destination:
def __init__(self, x, y):
self.x = x
self.y = y
def draw(self):
screen.blit(destination_image, (self.x*32, self.y*32))
# 初始化游戏角色
player = None
boxes = []
destinations = []
for y in range(len(game_map)):
for x in range(len(game_map[y])):
if game_map[y][x] == "P":
player = Player(x, y)
elif game_map[y][x] == "B":
boxes.append(Box(x, y))
elif game_map[y][x] == "D":
destinations.append(Destination(x, y))
# 游戏循环
while True:
# 处理游戏事件
for event in pygame.event.get():
if event.type == QUIT:
pygame.quit()
sys.exit()
elif event.type == KEYDOWN:
if event.key == K_LEFT:
player.move(-1, 0)
elif event.key == K_RIGHT:
player.move(1, 0)
elif event.key == K_UP:
player.move(0, -1)
elif event.key == K_DOWN:
player.move(0, 1)
# 绘制游戏场景
for y in range(len(game_map)):
for x in range(len(game_map[y])):
if game_map[y][x] == "W":
screen.blit(wall_image, (x*32, y*32))
elif game_map[y][x] == "D":
Destination(x, y).draw()
for box in boxes:
box.draw()
player.draw()
# 判断游戏是否胜利
win = True
for box in boxes:
if game_map[box.y][box.x] != "D":
win = False
break
if win:
pygame.quit()
sys.exit()
# 刷新游戏画面
pygame.display.update()
```
在运行代码之前,请先准备游戏素材(如墙壁、箱子、终点和人物的图片)并将其放置在与代码相同的目录下。此外,您还需要安装Pygame模块才能运行代码。
阅读全文
相关推荐




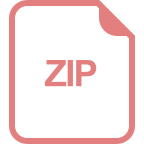








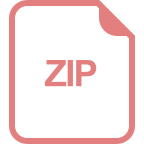