用python编写一个推箱子游戏
时间: 2023-07-18 13:25:40 浏览: 50
好的,推箱子游戏是一款非常有趣的益智游戏,下面是一个简单的Python实现:
```python
# 导入必要的库
import pygame
# 定义常量
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 480
CELL_SIZE = 32
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
BLUE = (0, 0, 255)
RED = (255, 0, 0)
# 定义游戏地图
MAP = [
"##########",
"# #",
"# $ #",
"# * #",
"# @ #",
"# #",
"##########"
]
# 定义游戏对象
class GameObject:
def __init__(self, x, y):
self.x = x
self.y = y
def draw(self, screen):
pass
# 定义墙壁对象
class Wall(GameObject):
def draw(self, screen):
pygame.draw.rect(screen, BLUE, [self.x, self.y, CELL_SIZE, CELL_SIZE])
# 定义箱子对象
class Box(GameObject):
def draw(self, screen):
pygame.draw.rect(screen, RED, [self.x, self.y, CELL_SIZE, CELL_SIZE])
# 定义目标对象
class Goal(GameObject):
def draw(self, screen):
pygame.draw.rect(screen, WHITE, [self.x, self.y, CELL_SIZE, CELL_SIZE])
# 定义玩家对象
class Player(GameObject):
def __init__(self, x, y):
super().__init__(x, y)
self.dx = 0
self.dy = 0
def draw(self, screen):
pygame.draw.rect(screen, WHITE, [self.x, self.y, CELL_SIZE, CELL_SIZE])
def move(self, dx, dy, boxes):
self.dx = dx
self.dy = dy
new_x = self.x + dx
new_y = self.y + dy
if self.check_collision(new_x, new_y, boxes):
self.x = new_x
self.y = new_y
def check_collision(self, x, y, boxes):
for box in boxes:
if box.x == x and box.y == y:
new_x = box.x + self.dx
new_y = box.y + self.dy
if self.check_collision(new_x, new_y, boxes):
box.x = new_x
box.y = new_y
return True
else:
return False
else:
return True
# 初始化游戏
def init_game():
# 初始化地图和对象列表
walls = []
boxes = []
goals = []
player = None
for row in range(len(MAP)):
for col in range(len(MAP[row])):
x = col * CELL_SIZE
y = row * CELL_SIZE
if MAP[row][col] == "#":
walls.append(Wall(x, y))
elif MAP[row][col] == "$":
boxes.append(Box(x, y))
elif MAP[row][col] == "*":
boxes.append(Box(x, y))
goals.append(Goal(x, y))
elif MAP[row][col] == "@":
player = Player(x, y)
return walls, boxes, goals, player
# 游戏循环
def run_game():
# 初始化pygame
pygame.init()
# 设置窗口大小和标题
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption("Push Box Game")
# 初始化游戏
walls, boxes, goals, player = init_game()
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
player.move(0, -CELL_SIZE, boxes)
elif event.key == pygame.K_DOWN:
player.move(0, CELL_SIZE, boxes)
elif event.key == pygame.K_LEFT:
player.move(-CELL_SIZE, 0, boxes)
elif event.key == pygame.K_RIGHT:
player.move(CELL_SIZE, 0, boxes)
# 绘制游戏
screen.fill(BLACK)
for wall in walls:
wall.draw(screen)
for box in boxes:
box.draw(screen)
for goal in goals:
goal.draw(screen)
player.draw(screen)
pygame.display.update()
# 退出pygame
pygame.quit()
# 运行游戏
if __name__ == "__main__":
run_game()
```
这个推箱子游戏使用了Pygame库来实现图形界面,游戏地图通过一个二维字符串来定义。你可以尝试修改MAP数组来创建自己的关卡。
相关推荐
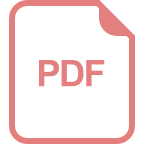
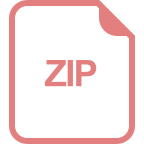












