用python写一个推箱子的代码
时间: 2023-12-17 14:54:58 浏览: 71
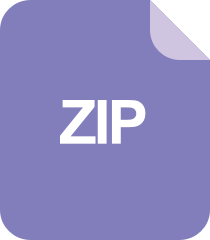
推箱子python代码

好的,推箱子游戏可以通过编写代码来实现。以下是一个简单的 Python 推箱子游戏代码示例:
```python
import copy
# 定义地图和方块
wall = '#'
empty = '-'
destination = '.'
man_on_empty = 'm'
man_on_destination = 'M'
box_on_empty = 'b'
box_on_destination = 'B'
# 初始化地图和方块位置
map_data = [
['#', '#', '#', '#', '#', '#', '#', '#', '#', '#', '#', '#'],
['#', '-', '-', '-', '#', '-', '-', '-', '-', '-', '.', '#'],
['#', '-', '#', '-', '#', '-', '#', '#', '#', '#', '-', '#'],
['#', '-', '#', '-', '-', '-', '-', '-', '-', '#', 'B', '#'],
['#', '-', '#', '-', '#', '#', '#', '#', '-', '#', '-', '#'],
['#', '-', '-', '-', '#', '.', '-', '-', '-', '#', '-', '#'],
['#', '-', '#', '-', '-', '-', '#', '#', '-', '#', '-', '#'],
['#', '-', '#', '#', '#', '-', '#', '-', '-', '#', '-', '#'],
['#', '-', '-', '-', '#', '-', '-', '-', '-', '-', '-', '#'],
['#', '#', '#', '#', '#', '#', '#', '#', '#', '#', '#', '#']]
# 初始化人和箱子位置
man_row = 1
man_col = 1
box_row = 3
box_col = 10
# 判断是否胜利
def is_win():
for row in range(1, len(map_data)-1):
for col in range(1, len(map_data[row])-1):
if map_data[row][col] == destination or map_data[row][col] == man_on_destination:
return False
return True
# 移动控制
def move(delta_row, delta_col):
global man_row, man_col, box_row, box_col
# 判断下一步位置是否合法
if map_data[man_row+delta_row][man_col+delta_col] == wall:
return False
if map_data[man_row+delta_row][man_col+delta_col] == box_on_empty or map_data[man_row+delta_row][man_col+delta_col] == box_on_destination:
if map_data[box_row+delta_row][box_col+delta_col] != empty and map_data[box_row+delta_row][box_col+delta_col] != destination:
return False
# 移动人和箱子
map_data[man_row][man_col] = destination if map_data[man_row][man_col] == man_on_destination else empty
map_data[man_row+delta_row][man_col+delta_col] = man_on_destination if map_data[man_row+delta_row][man_col+delta_col] == destination else man_on_empty
if map_data[man_row+delta_row][man_col+delta_col] == box_on_empty or map_data[man_row+delta_row][man_col+delta_col] == box_on_destination:
map_data[box_row+delta_row][box_col+delta_col] = box_on_destination if map_data[box_row+delta_row][box_col+delta_col] == destination else box_on_empty
# 更新箱子位置
box_row += delta_row
box_col += delta_col
# 更新人的位置
man_row += delta_row
man_col += delta_col
return True
# 游戏循环
while not is_win():
# 绘制地图
for row in range(len(map_data)):
for col in range(len(map_data[row])):
print(map_data[row][col], end='')
print()
print()
# 获取用户输入
direction = input('请输入方向(w=上,s=下,a=左,d=右):')
if direction == 'w':
if not move(-1, 0):
print('不合法的移动!')
elif direction == 's':
if not move(1, 0):
print('不合法的移动!')
elif direction == 'a':
if not move(0, -1):
print('不合法的移动!')
elif direction == 'd':
if not move(0, 1):
print('不合法的移动!')
else:
print('无效的输入!')
print('恭喜您,通关了!')
```
这个程序会输出推箱子游戏的地图,并等待用户输入方向进行移动。在用户输入方向后,程序判断下一步位置是否合法,如果位置不合法则输出错误提示信息。如果位置合法,则移动人和箱子,并更新地图。最后输出恭喜通关的消息。
阅读全文
相关推荐












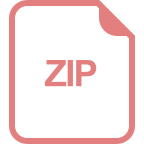
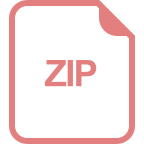