连接28BYJ步进电机和ARM板的驱动程序怎么写
时间: 2024-06-12 21:03:37 浏览: 75
下面是一个示例程序,用于连接28BYJ步进电机和ARM板的驱动程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <wiringPi.h>
#define IN1 0 //定义引脚
#define IN2 1
#define IN3 2
#define IN4 3
const int stepsPerRevolution = 4096; //每圈步数
int stepCount = 0; //计数器,记录已经转过的步数
int stepDelay = 2; //每步之间的延迟时间,控制步进电机的速度
int sequence[][4] = {{1, 0, 0, 1}, //步进电机的步序,依次为IN1, IN2, IN3, IN4
{0, 1, 0, 1},
{0, 1, 1, 0},
{1, 0, 1, 0}};
void setStep(int a, int b, int c, int d) //控制引脚输出的函数
{
digitalWrite(IN1, a);
digitalWrite(IN2, b);
digitalWrite(IN3, c);
digitalWrite(IN4, d);
}
void step(int count, int dir) //步进函数,count为需要转的步数,dir为方向,1为正转,-1为反转
{
int i;
for (i = 0; i < count; i++) {
stepCount += dir;
if (stepCount == stepsPerRevolution) { //如果已经转了一圈,重置计数器
stepCount = 0;
}
else if (stepCount == -1) { //如果已经转过了一圈再反转,也要重置计数器
stepCount = stepsPerRevolution - 1;
}
setStep(sequence[stepCount % 4][0], sequence[stepCount % 4][1], sequence[stepCount % 4][2], sequence[stepCount % 4][3]);
delay(stepDelay);
}
}
int main(void)
{
wiringPiSetup(); //初始化wiringPi库
pinMode(IN1, OUTPUT); //设置引脚模式
pinMode(IN2, OUTPUT);
pinMode(IN3, OUTPUT);
pinMode(IN4, OUTPUT);
while (1) {
step(2048, 1); //正转2048步
delay(1000);
step(2048, -1); //反转2048步
delay(1000);
}
return 0;
}
```
在这个示例程序中,我们使用了wiringPi库来控制GPIO引脚的输入输出。程序中定义了步进电机的步序,以及每步之间的延迟时间,通过控制步进电机的引脚输出,使其按照指定的步序运动。在主函数中,我们通过循环控制步进电机的运动,先是正转2048步,然后反转2048步,每次转完之后都会延迟1秒钟。
阅读全文
相关推荐
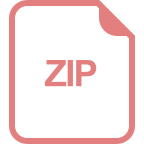

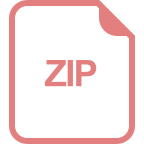
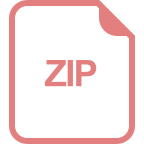
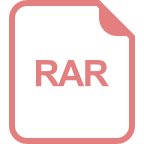
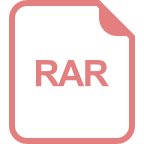
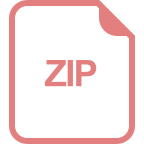
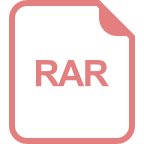
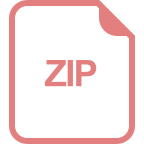
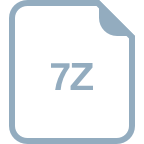
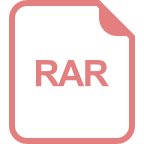
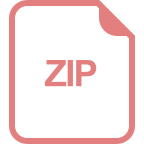
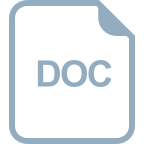
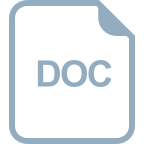
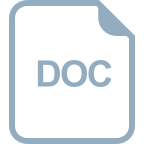

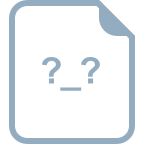