python写遗传算法
时间: 2023-11-07 11:01:09 浏览: 40
以下是Python中的简单遗传算法实现示例:
```
import random
# 目标函数,本例为求解x^2的最小值,x的范围在-10到10之间
def fitness_function(x):
return x**2
# 生成初始种群
def generate_initial_population(population_size):
population = []
for i in range(population_size):
individual = {'chromosome': random.uniform(-10, 10)}
individual['fitness'] = fitness_function(individual['chromosome'])
population.append(individual)
return population
# 选择操作,采用轮盘赌选择
def selection(population):
total_fitness = sum([individual['fitness'] for individual in population])
selection_probs = [(individual['fitness'] / total_fitness) for individual in population]
selected_individuals = []
for i in range(len(population)):
selected_individual = random.choices(population, weights=selection_probs)[0]
selected_individuals.append(selected_individual)
return selected_individuals
# 交叉操作,采用单点交叉
def crossover(parent1, parent2):
offspring1 = parent1['chromosome']
offspring2 = parent2['chromosome']
if random.random() < crossover_rate:
crossover_point = random.randint(1, len(bin(int(parent1['chromosome']))[2:])-1)
mask = int('1' * crossover_point + '0' * (len(bin(int(parent1['chromosome']))[2:])-crossover_point), 2)
offspring1 = (parent1['chromosome'] & mask) + (parent2['chromosome'] & ~mask)
offspring2 = (parent2['chromosome'] & mask) + (parent1['chromosome'] & ~mask)
offspring1 = {'chromosome': offspring1}
offspring2 = {'chromosome': offspring2}
offspring1['fitness'] = fitness_function(offspring1['chromosome'])
offspring2['fitness'] = fitness_function(offspring2['chromosome'])
return offspring1, offspring2
# 变异操作,采用随机变异
def mutation(individual):
mutated_chromosome = individual['chromosome']
if random.random() < mutation_rate:
mutation_bit = random.randint(1, len(bin(int(individual['chromosome']))[2:]))
mask = 1 << (mutation_bit - 1)
mutated_chromosome = individual['chromosome'] ^ mask
mutated_individual = {'chromosome': mutated_chromosome}
mutated_individual['fitness'] = fitness_function(mutated_individual['chromosome'])
return mutated_individual
# 主程序
population_size = 50
crossover_rate = 0.8
mutation_rate = 0.1
max_generations = 100
# 生成初始种群
population = generate_initial_population(population_size)
# 迭代
for generation in range(max_generations):
# 选择
selected_individuals = selection(population)
# 交叉
offspring_population = []
for i in range(0, len(selected_individuals), 2):
parent1 = selected_individuals[i]
parent2 = selected_individuals[i+1]
offspring1, offspring2 = crossover(parent1, parent2)
offspring_population.append(offspring1)
offspring_population.append(offspring2)
# 变异
mutated_population = [mutation(individual) for individual in offspring_population]
# 合并种群
population = selected_individuals + mutated_population
# 选择最优个体
best_individual = min(population, key=lambda x: x['fitness'])
print('最优解为:', best_individual['chromosome'])
print('最优解对应的函数值为:', best_individual['fitness'])
```
请注意,此示例中的目标函数和操作是根据问题而定的,您需要根据您的问题进行相应的修改。此外,此示例还需要其他优化,例如添加停止准则和参数优化等。
相关推荐
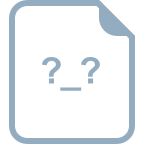
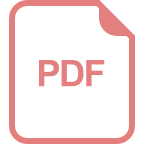














