python实现遗传算法代码
时间: 2024-05-14 07:11:04 浏览: 117
遗传算法是一种优化算法,可以用来求解函数最大值或最小值问题。Python是一种非常适合编写遗传算法的编程语言。以下是一个简单的Python实现遗传算法的例子:
1. 初始化种群:生成一组随机的个体作为初始种群,每个个体都是由一组基因组成的。
2. 评估适应度:对每个个体计算其适应度,适应度越高表示该个体越优秀。
3. 选择:从当前种群中选择一部分个体作为下一代的父代,通常采用轮盘赌选择或者锦标赛选择方法。
4. 交叉:对父代个体进行交叉操作,产生新的后代个体。
5. 变异:对后代个体进行变异操作,引入一些随机因素,增加种群多样性。
6. 更新种群:用新的后代个体替换掉原来的父代个体,形成新的种群。
7. 重复上述过程,直到达到停止条件。
具体的Python代码如下:
```python
import random
# 初始化种群
def init_population(pop_size, chrom_size):
population = []
for i in range(pop_size):
chromosome = [random.randint(0, 1) for j in range(chrom_size)]
population.append(chromosome)
return population
# 计算适应度
def fitness(chromosome):
x = int(''.join([str(i) for i in chromosome]), 2)
return x * x
# 选择
def selection(population, fitness):
fitness_sum = sum(fitness)
probability = [f / fitness_sum for f in fitness]
probability_sum = [sum(probability[:i+1]) for i in range(len(probability))]
new_population = []
for i in range(len(population)):
r = random.random()
for j in range(len(probability_sum)):
if r <= probability_sum[j]:
new_population.append(population[j])
break
return new_population
# 交叉
def crossover(parent1, parent2, pc):
if random.random() > pc:
return parent1, parent2
pos = random.randint(0, len(parent1)-1)
child1 = parent1[:pos] + parent2[pos:]
child2 = parent2[:pos] + parent1[pos:]
return child1, child2
# 变异
def mutation(child, pm):
for i in range(len(child)):
if random.random() < pm:
child[i] = 1 - child[i]
return child
# 遗传算法主程序
def genetic_algorithm(pop_size, chrom_size, pc, pm, max_iter):
population = init_population(pop_size, chrom_size)
best_fitness = -float('inf')
best_chromosome = None
for i in range(max_iter):
fitness_list = [fitness(chromosome) for chromosome in population]
if max(fitness_list) > best_fitness:
best_fitness = max(fitness_list)
index = fitness_list.index(best_fitness)
best_chromosome = population[index]
population = selection(population, fitness_list)
new_population = []
for j in range(0, len(population), 2):
if j+1 < len(population):
child1, child2 = crossover(population[j], population[j+1], pc)
child1 = mutation(child1, pm)
child2 = mutation(child2, pm)
new_population.append(child1)
new_population.append(child2)
population = new_population
return best_chromosome, best_fitness
# 测试代码
if __name__ == '__main__':
pop_size = 100
chrom_size = 8
pc = 0.8
pm = 0.01
max_iter = 100
best_chromosome, best_fitness = genetic_algorithm(pop_size, chrom_size, pc, pm, max_iter)
print('Best Chromosome:', best_chromosome)
print('Best Fitness:', best_fitness)
```
阅读全文
相关推荐
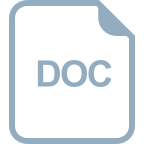
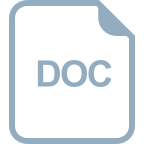
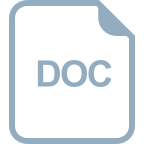
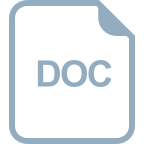


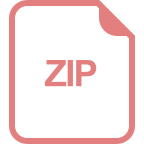
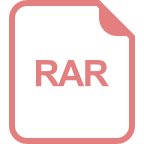
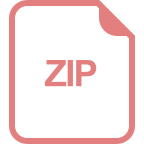
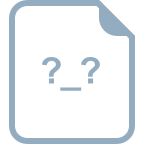
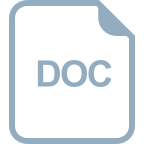
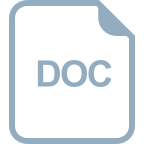
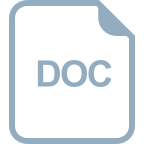
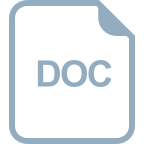
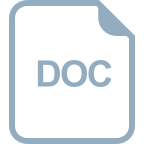
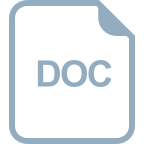
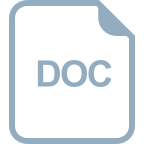

