用python实现遗传算法的源代码
时间: 2023-08-31 22:32:01 浏览: 102
### 回答1:
以下是一个简单的使用Python实现遗传算法的示例代码,用于解决一个基本的优化问题:
```python
import random
# 定义优化目标函数
def fitness(individual):
# 假设我们要最小化函数 f(x) = x^2
return individual[0]**2
# 初始化种群
def init_population(pop_size, gene_size):
population = []
for i in range(pop_size):
individual = [random.uniform(-5, 5) for _ in range(gene_size)]
population.append(individual)
return population
# 选择操作
def selection(population, fitness_func):
fitness_list = [fitness_func(individual) for individual in population]
total_fitness = sum(fitness_list)
selection_prob = [fitness / total_fitness for fitness in fitness_list]
return random.choices(population, weights=selection_prob)
# 交叉操作
def crossover(individual1, individual2):
crossover_point = random.randint(1, len(individual1)-1)
child1 = individual1[:crossover_point] + individual2[crossover_point:]
child2 = individual2[:crossover_point] + individual1[crossover_point:]
return child1, child2
# 变异操作
def mutation(individual, gene_range=(-5, 5), mutation_prob=0.1):
for i in range(len(individual)):
if random.random() < mutation_prob:
individual[i] += random.uniform(*gene_range)
return individual
# 遗传算法主程序
def genetic_algorithm(pop_size, gene_size, max_gen, fitness_func):
population = init_population(pop_size, gene_size)
for i in range(max_gen):
new_population = []
for j in range(pop_size//2):
parent1 = selection(population, fitness_func)
parent2 = selection(population, fitness_func)
child1, child2 = crossover(parent1[0], parent2[0])
child1 = mutation(child1)
child2 = mutation(child2)
new_population.append(child1)
new_population.append(child2)
population = new_population
return min(population, key=fitness_func)
# 使用遗传算法寻找函数 f(x) = x^2 的最小值
best_individual = genetic_algorithm(pop_size=100, gene_size=1, max_gen=100, fitness_func=fitness)
print('Best individual:', best_individual, 'Fitness:', fitness(best_individual))
```
在这个示例代码中,我们定义了一个简单的优化目标函数f(x) = x^2,然后使用遗传算法来寻找该函数的最小值。在遗传算法主程序中,我们首先初始化一个种群,然后进行一定数量的进化代数。在每个进化代数中,我们选择两个个体进行交叉和变异,然后将新的个体加入到下一代种群中。最后,我们返回最适应的个体,即具有最小适应度的个体。
请注意,这只是一个简单的示例代码,实际上,使用遗传算法解决复杂的优化问题需要更多的优化和调整,例如选择操作和交叉操作的变化,变异率和种群大小的优化等。
### 回答2:
遗传算法是一种模仿进化原理设计问题解决方案的优化算法。下面是使用Python实现遗传算法的源代码示例:
```python
import random
# 适应度函数:评估染色体的适应度
def fitness(chromosome):
# TODO: 根据问题需求,计算染色体的适应度
pass
# 交叉操作:对于两个父代染色体,生成子代染色体
def crossover(parent1, parent2):
# TODO: 根据问题需求,实现交叉操作
pass
# 变异操作:对染色体进行基因突变
def mutation(chromosome):
# TODO: 根据问题需求,实现变异操作
pass
# 创建初始种群
def create_population(population_size, chromosome_length):
population = []
for _ in range(population_size):
chromosome = [random.randint(0, 1) for _ in range(chromosome_length)]
population.append(chromosome)
return population
# 遗传算法主函数
def genetic_algorithm(population_size, chromosome_length, max_generations):
# 参数设置
crossover_rate = 0.8 # 交叉概率
mutation_rate = 0.1 # 变异概率
# 创建初始种群
population = create_population(population_size, chromosome_length)
# 逐代优化
for generation in range(max_generations):
# 计算适应度
fitness_scores = [fitness(chromosome) for chromosome in population]
# 选择
selected_parent_indexes = random.choices(
population=range(population_size),
weights=fitness_scores,
k=population_size
)
# 繁殖
new_population = []
for i in range(0, population_size, 2):
parent1 = population[selected_parent_indexes[i]]
parent2 = population[selected_parent_indexes[i+1]]
# 交叉
if random.random() < crossover_rate:
child1, child2 = crossover(parent1, parent2)
else:
child1, child2 = parent1, parent2
# 变异
if random.random() < mutation_rate:
child1 = mutation(child1)
if random.random() < mutation_rate:
child2 = mutation(child2)
new_population.extend([child1, child2])
population = new_population
# 返回最优解
best_chromosome = max(population, key=fitness)
return best_chromosome
# 调用遗传算法主函数
population_size = 100 # 种群大小
chromosome_length = 10 # 染色体长度
max_generations = 100 # 最大迭代次数
best_chromosome = genetic_algorithm(population_size, chromosome_length, max_generations)
print(best_chromosome)
```
以上代码为一个简单的遗传算法框架,其中的各个函数需要根据具体问题需求进行相应的实现。
### 回答3:
以下是使用Python编写的一个简单遗传算法的示例代码:
```
import random
# 设置问题的解空间范围
DNA_SIZE = 10 # 基因长度
POP_SIZE = 100 # 种群数量
TARGET = "Hello" # 目标字符串
# 初始化种群
def init_population(pop_size, dna_size):
pop = []
for _ in range(pop_size):
individual = ''.join(random.choice('abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ') for _ in range(dna_size))
pop.append(individual)
return pop
# 计算个体的适应度
def fitness(individual):
score = 0
for i in range(len(individual)):
if individual[i] == TARGET[i]:
score += 1
return score / len(TARGET)
# 选择种群中的父代个体
def selection(pop, k=0.5):
fitness_scores = [fitness(individual) for individual in pop]
total_score = sum(fitness_scores)
probabilities = [score / total_score for score in fitness_scores]
selected = random.choices(pop, weights=probabilities, k=int(len(pop) * k))
return selected
# 交叉操作
def crossover(parent1, parent2):
point = random.randint(1, DNA_SIZE - 1)
child1 = parent1[:point] + parent2[point:]
child2 = parent2[:point] + parent1[point:]
return child1, child2
# 突变操作
def mutation(individual):
point = random.randint(0, DNA_SIZE - 1)
gene = random.choice('abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ')
mutated = individual[:point] + gene + individual[point+1:]
return mutated
# 遗传算法主程序
def genetic_algorithm(pop_size, dna_size, generations=100):
population = init_population(pop_size, dna_size)
for _ in range(generations):
parents = selection(population)
offspring = []
while len(offspring) < pop_size:
parent1, parent2 = random.sample(parents, 2)
child1, child2 = crossover(parent1, parent2)
child1 = mutation(child1)
child2 = mutation(child2)
offspring.append(child1)
offspring.append(child2)
population = offspring
best_individual = max(population, key=fitness)
return best_individual
# 运行遗传算法
best_solution = genetic_algorithm(POP_SIZE, DNA_SIZE)
print("Best Solution:", best_solution)
```
上面的代码实现了一个简单的遗传算法,用于解决字符串匹配问题。代码中的`init_population`函数用于初始化种群,`fitness`函数用于计算个体的适应度,`selection`函数用于选择父代个体,`crossover`函数用于进行交叉操作,`mutation`函数用于进行突变操作,`genetic_algorithm`函数是遗传算法的主程序。最后,使用`genetic_algorithm`函数运行遗传算法并打印出最佳解。
相关推荐
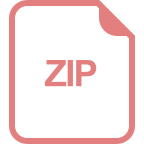









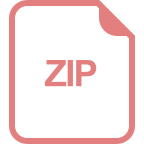
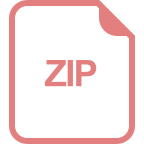
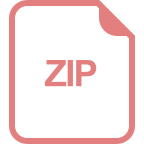