用C语言输入2012年(闰年)的一个月份,输出这个月的天数。(switch语句)
时间: 2024-05-06 18:19:38 浏览: 119
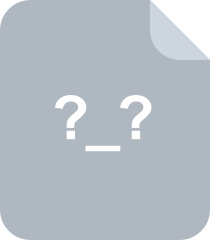
输入年和月的信息,输出该月对应的天数。注意合理性与闰年的判别。(switch语句练习)

```c
#include <stdio.h>
int main() {
int month;
printf("请输入一个月份:");
scanf("%d", &month);
switch(month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
printf("该月有31天。\n");
break;
case 4:
case 6:
case 9:
case 11:
printf("该月有30天。\n");
break;
case 2:
printf("该月有29天。\n");
break;
default:
printf("输入的月份不合法!\n");
break;
}
return 0;
}
```
阅读全文
相关推荐















