用C++实现base64编码
时间: 2023-07-11 15:32:23 浏览: 67
以下是使用C++实现base64编码的示例代码:
```c++
#include <iostream>
#include <string>
#include <vector>
std::string base64_encode(const std::string& input)
{
std::string base64_chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789+/";
std::string output;
int bits = 0;
int bit_count = 0;
unsigned char ch = 0;
for (const auto& c : input)
{
ch = c;
bits = (bits << 8) | ch;
bit_count += 8;
while (bit_count >= 6)
{
bit_count -= 6;
ch = (bits >> bit_count) & 0x3f;
output += base64_chars[ch];
}
}
if (bit_count > 0)
{
bits = bits << (6 - bit_count);
ch = bits & 0x3f;
output += base64_chars[ch];
while (bit_count < 6)
{
output += '=';
bit_count += 2;
}
}
return output;
}
int main()
{
std::string input = "Hello, world!";
std::string encoded = base64_encode(input);
std::cout << encoded << std::endl;
return 0;
}
```
在这个示例代码中,我们定义了一个 `base64_encode` 函数,它接受一个字符串作为输入,并返回一个编码过的字符串。在实现中,我们使用了一个 `base64_chars` 字符串来存储可用的字符。我们遍历输入字符串,并将每个字符转换为一个 8 位的整数,然后将这些整数合并为一个大的整数。我们将大的整数分成 6 位的块,并将每个块转换为一个可用的字符。最后,我们将所有字符连接起来,形成编码过的字符串。如果输入字符串的长度不是三的倍数,我们将使用 `=` 字符进行填充,使其长度变为三的倍数。
相关推荐








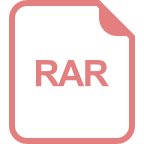
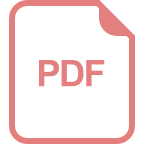
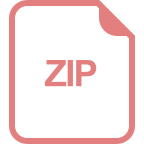