html设计用户注册界面,收集用户手机号、账号、密码、邮箱等信息。要求:(1)设计注册界面;(2)为了确保用户数据有效,须在用户提交数据时验证数据有效性,设计程序验证用户数据有效性。
时间: 2023-06-15 10:07:54 浏览: 56
首先,我们可以设计一个基本的用户注册界面,其中包括以下字段:
1. 手机号
2. 账号
3. 密码
4. 确认密码
5. 邮箱
界面可以使用HTML和CSS进行设计,如下所示:
```html
<!DOCTYPE html>
<html>
<head>
<title>User Registration</title>
<style>
form {
width: 50%;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
}
label {
display: block;
margin-bottom: 10px;
}
input[type="text"], input[type="password"], input[type="email"] {
padding: 10px;
margin-bottom: 10px;
border-radius: 5px;
border: 1px solid #ccc;
width: 100%;
}
input[type="submit"] {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
}
</style>
</head>
<body>
<form action="register.php" method="post">
<label for="phone">Phone Number:</label>
<input type="text" id="phone" name="phone" required>
<label for="username">Username:</label>
<input type="text" id="username" name="username" required>
<label for="password">Password:</label>
<input type="password" id="password" name="password" required>
<label for="confirm_password">Confirm Password:</label>
<input type="password" id="confirm_password" name="confirm_password" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<input type="submit" value="Register">
</form>
</body>
</html>
```
接下来,我们需要设计一个程序来验证用户提交的数据是否有效。可以使用PHP来实现此功能,下面是一个简单的示例:
```php
<?php
// 获取用户提交的数据
$phone = $_POST['phone'];
$username = $_POST['username'];
$password = $_POST['password'];
$confirm_password = $_POST['confirm_password'];
$email = $_POST['email'];
// 验证手机号格式是否正确
if (!preg_match("/^[0-9]{11}$/", $phone)) {
die("Invalid phone number.");
}
// 验证用户名是否为空
if (empty($username)) {
die("Username cannot be empty.");
}
// 验证密码和确认密码是否相同
if ($password !== $confirm_password) {
die("Passwords do not match.");
}
// 验证邮箱格式是否正确
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
die("Invalid email address.");
}
// 执行用户注册操作
// TODO: 在此添加代码来执行用户注册操作
echo "Registration successful.";
?>
```
以上代码使用了正则表达式来验证手机号格式是否正确,使用if语句来验证其他字段是否有效。如果任何字段无效,则程序将停止执行并输出相应的错误消息。如果所有字段都有效,则程序将执行用户注册操作并输出“Registration successful.”消息。
注意:以上示例代码仅供参考,实际情况中需要根据实际需求进行修改和完善。
相关推荐
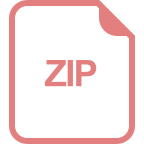
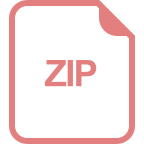
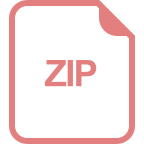
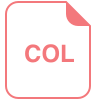
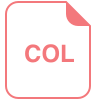
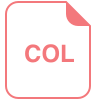











