原型继承如何实现?
时间: 2023-11-27 13:14:04 浏览: 42
在 JavaScript 中,原型继承可以通过以下几种方式来实现:
1. 构造函数继承:通过在子类构造函数中调用父类构造函数来实现属性继承。在子类构造函数中调用父类构造函数可以使用 call 或 apply 方法实现。
```js
function Parent(name) {
this.name = name;
}
function Child(name, age) {
Parent.call(this, name);
this.age = age;
}
```
2. 原型链继承:通过将子类的原型对象指向父类的实例来实现原型继承。
```js
function Parent() {}
Parent.prototype.sayHello = function() {
console.log('Hello');
}
function Child() {}
Child.prototype = new Parent();
Child.prototype.constructor = Child;
```
3. 组合继承:通过将构造函数继承和原型链继承结合起来,既可以继承属性,也可以继承方法。
```js
function Parent(name) {
this.name = name;
}
Parent.prototype.sayHello = function() {
console.log('Hello');
}
function Child(name, age) {
Parent.call(this, name);
this.age = age;
}
Child.prototype = new Parent();
Child.prototype.constructor = Child;
```
4. 原型式继承:通过 Object.create 方法创建一个新对象,并将该对象的原型指向一个已有的对象,从而实现继承。
```js
var parent = {
name: 'parent',
sayHello: function() {
console.log('Hello');
}
};
var child = Object.create(parent);
child.age = 18;
```
5. 寄生式继承:通过在原型式继承的基础上,增强新对象,从而实现继承。
```js
function createChild(parent) {
var child = Object.create(parent);
child.sayName = function() {
console.log(this.name);
}
return child;
}
var parent = {
name: 'parent'
};
var child = createChild(parent);
child.sayName();
```
6. 寄生组合式继承:通过在组合继承的基础上,优化父类实例的创建,从而实现继承。
```js
function Parent(name) {
this.name = name;
}
Parent.prototype.sayHello = function() {
console.log('Hello');
}
function Child(name, age) {
Parent.call(this, name);
this.age = age;
}
Child.prototype = Object.create(Parent.prototype);
Child.prototype.constructor = Child;
```
相关推荐
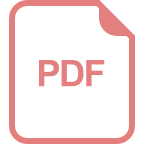
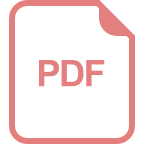














