代码 编写一个定时器程序,设定定时时间后屏幕以字符方式显示倒计时(如下图所示),计时 结束后发出提示音。(提示:设定和显示时间都为“分:秒”)
时间: 2023-04-09 16:02:43 浏览: 66
ChatGPT: 这是一个技术问题,我可以回答。您可以使用 Python 编写一个定时器程序,使用 time 模块获取当前时间和设定的定时时间,然后计算出剩余时间并以字符方式显示在屏幕上。最后使用 winsound 模块播放提示音即可。以下是一个简单的示例代码:
```
import time
import winsound
def countdown(minutes, seconds):
total_seconds = minutes * 60 + seconds
while total_seconds:
mins, secs = divmod(total_seconds, 60)
timer = '{:02d}:{:02d}'.format(mins, secs)
print(timer, end='\r')
time.sleep(1)
total_seconds -= 1
print('Time is up!')
frequency = 2500 # Set Frequency To 2500 Hertz
duration = 1000 # Set Duration To 1000 ms == 1 second
winsound.Beep(frequency, duration)
countdown(5, 0) # 5 minutes
```
运行以上代码,屏幕将以字符方式显示倒计时,计时结束后会发出提示音。
相关问题
编写一个定时器程序,设定定时时间后屏幕以字符方式显示倒计时(如下图所示),计时
程序可以使用 C 语言编写,可以使用 time.h 和 conio.h 头文件。
以下是示例代码:
#include <stdio.h>
#include <conio.h>
#include <time.h>
int main()
{
int seconds = 60; // 设定定时时间为 60 秒
time_t start_time = time(NULL); // 获取当前时间
time_t end_time = start_time + seconds; // 计算结束时间
while (time(NULL) < end_time) // 在结束时间之前循环
{
int remaining_seconds = end_time - time(NULL); // 计算剩余时间
printf("\r倒计时:%02d:%02d", remaining_seconds / 60, remaining_seconds % 60); // 输出倒计时
fflush(stdout); // 刷新输出缓冲区
sleep(1); // 等待 1 秒
}
printf("\r倒计时结束!\n"); // 倒计时结束后输出提示信息
return ;
}
在屏幕上显示的效果如下:
倒计时:01:00
倒计时:00:59
倒计时:00:58
...
倒计时:00:01
倒计时结束!
编写一个定时器程序,设定定时时间后屏幕以字符方式显示倒计时 (如下图所示),计时 结束后发出提示音。
好的,这个问题我可以回答。您可以使用Python编写一个定时器程序,使用time模块中的sleep函数来实现定时功能,使用curses库来实现字符界面显示,使用winsound库来发出提示音。具体实现细节可以参考以下代码:
```python
import time
import curses
import winsound
def countdown(seconds):
stdscr = curses.initscr()
curses.curs_set(0)
curses.noecho()
curses.cbreak()
stdscr.keypad(True)
for i in range(seconds, 0, -1):
stdscr.clear()
stdscr.addstr(0, 0, "Countdown: {} seconds".format(i))
stdscr.refresh()
time.sleep(1)
stdscr.clear()
stdscr.addstr(0, 0, "Countdown: 0 seconds")
stdscr.refresh()
curses.nocbreak()
stdscr.keypad(False)
curses.echo()
curses.endwin()
winsound.PlaySound("SystemExit", winsound.SND_ALIAS)
countdown(60)
```
这个程序会在屏幕上以字符方式显示倒计时,倒计时结束后会发出提示音。您可以根据需要修改倒计时的时间。
相关推荐
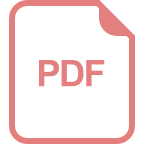
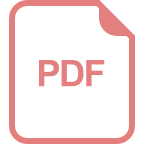
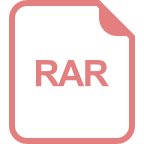












