基于ffmpeg将摄像头采集的mat类型数据进行编码
时间: 2023-12-24 09:03:05 浏览: 57
要基于ffmpeg将摄像头采集的mat类型数据进行编码,可以分为以下步骤:
1. 初始化ffmpeg库,包括注册编解码器、格式器等组件;
2. 配置编码器,包括设置输出格式、编码参数、分辨率、帧率等参数;
3. 创建AVFrame结构体,将mat类型数据转换为AVFrame格式;
4. 使用编码器对AVFrame进行编码,并将编码后的数据写入输出文件或输出流;
5. 循环执行第3、4步,直到所有数据都被编码完成;
6. 释放资源,包括关闭编码器、销毁AVFrame等。
下面是一个简单的示例代码,可以将摄像头采集的mat类型数据编码为H.264格式的视频:
```cpp
// 初始化ffmpeg库
av_register_all();
avcodec_register_all();
// 配置编码器
AVCodec* codec = avcodec_find_encoder(AV_CODEC_ID_H264);
AVCodecContext* codec_context = avcodec_alloc_context3(codec);
codec_context->bit_rate = 400000;
codec_context->width = width;
codec_context->height = height;
codec_context->time_base = { 1, 25 };
codec_context->framerate = { 25, 1 };
codec_context->gop_size = 10;
codec_context->max_b_frames = 1;
codec_context->pix_fmt = AV_PIX_FMT_YUV420P;
// 打开编码器
avcodec_open2(codec_context, codec, NULL);
// 创建AVFrame结构体
AVFrame* frame = av_frame_alloc();
frame->width = width;
frame->height = height;
frame->format = AV_PIX_FMT_YUV420P;
av_frame_get_buffer(frame, 0);
// 循环编码
while (true) {
// 从摄像头采集一帧图像
cv::Mat image;
cap >> image;
// 将Mat类型数据转换为AVFrame格式
AVFrame* src_frame = av_frame_alloc();
av_image_fill_arrays(src_frame->data, src_frame->linesize, image.data, AV_PIX_FMT_BGR24, width, height, 1);
// 转换颜色空间
SwsContext* sws_context = sws_getContext(width, height, AV_PIX_FMT_BGR24, width, height, AV_PIX_FMT_YUV420P, 0, NULL, NULL, NULL);
sws_scale(sws_context, src_frame->data, src_frame->linesize, 0, height, frame->data, frame->linesize);
// 编码AVFrame并写入输出文件或输出流
AVPacket packet;
av_init_packet(&packet);
packet.data = NULL;
packet.size = 0;
avcodec_send_frame(codec_context, frame);
while (avcodec_receive_packet(codec_context, &packet) == 0) {
// 写入输出文件或输出流
fwrite(packet.data, 1, packet.size, output_file);
av_packet_unref(&packet);
}
// 释放资源
av_frame_free(&src_frame);
sws_freeContext(sws_context);
}
// 关闭编码器、销毁AVFrame
av_write_trailer(output_file);
avcodec_close(codec_context);
avcodec_free_context(&codec_context);
av_frame_free(&frame);
```
需要注意的是,以上代码只是一个简单的示例,实际使用时还需要根据具体情况进行调整和优化。同时,还需要注意处理异常情况和错误信息,以保证程序的健壮性。
相关推荐
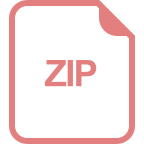
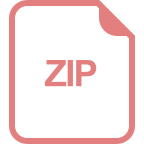
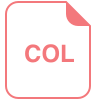













