用python3.6的版本 四叉树均匀图像的特征点
时间: 2024-05-04 12:17:41 浏览: 110
四叉树是一种用于将二维平面分割成四个象限数据结构。在图处理中,四叉树常用于对图像进行分割和特征提取。
以下是一个用Python 3.6实现对像进行四叉树分割特征点提取的例代码:
```python
import cv
import numpy as np
# 定义四叉节点类
class QuadtreeNode:
def __init__(self, x, y, w, h, threshold):
self.x = x
self.y = y
self.w = w
self.h = h
self.threshold = threshold
self.color = -1
self.children = []
def is_leaf(self):
return len(self.children) == 0
# 计算图像的灰度直方图
def compute_histogram(img):
hist = np.zeros(256)
for i in range(img.shape[0]):
for j in range(img.shape[1]):
hist[img[i][j]] += 1
return hist
# 计算图像的方差
def compute_variance(img):
mean = np.mean(img)
variance = 0
for i in range(img.shape[0]):
for j in range(img.shape[1]):
variance += (img[i][j] - mean) ** 2
variance /= (img.shape[0] * img.shape[1])
return variance
# 构建四叉树
def build_quadtree(img, x, y, w, h, threshold):
node = QuadtreeNode(x, y, w, h, threshold)
if w <= 1 or h <= 1:
node.color = img[y][x]
elif compute_variance(img[y:y+h, x:x+w]) <= threshold:
node.color = img[y][x]
else:
nw = build_quadtree(img, x, y, w//2, h//2, threshold)
ne = build_quadtree(img, x+w//2, y, w-w//2, h//2, threshold)
sw = build_quadtree(img, x, y+h//2, w//2, h-h//2, threshold)
se = build_quadtree(img, x+w//2, y+h//2, w-w//2, h-h//2, threshold)
node.children = [nw, ne, sw, se]
return node
# 提取特征点
def extract_feature_points(node, points):
if node.is_leaf():
points.append((node.x + node.w // 2, node.y + node.h // 2))
else:
for child in node.children:
extract_feature_points(child, points)
# 加载图像
img = cv2.imread('image.jpg', cv2.IMREAD_GRAYSCALE)
# 构建四叉树
root = build_quadtree(img, 0, 0, img.shape[1], img.shape[0], 50)
# 提取特征点
points = []
extract_feature_points(root, points)
# 在图像上绘制特征点
for x, y in points:
cv2.circle(img, (x, y), 2, (255, 0, 0), -1)
# 显示图像
cv2.imshow('image', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的代码中,我们首先定义了一个`QuadtreeNode`类来表示四叉树的节点。然后,我们实现了三个辅助函数:
- `compute_histogram`:计算图像的灰度直方图。
- `compute_variance`:计算图像的方差。
- `build_quadtree`:构建四叉树。
在`build_quadtree`函数中,我们使用递归的方式对图像进行分割。如果当前节点表示的区域的宽度或高度小于等于1,或者区域的方差小于等于给定的阈值,则将当前节点标记为叶节点,并用区域中心的灰度值填充它。否则,将当前节点分成四个子节点,并递归地构建它们。
最后,我们实现了一个`extract_feature_points`函数来提取特征点。如果当前节点是叶节点,则将它的中心坐标添加到特征点列表中。否则,递归地提取子节点的特征点。
在主程序中,我们首先加载一张图像,并使用`build_quadtree`函数构建四叉树。然后,我们使用`extract_feature_points`函数提取特征点,并在图像上绘制它们。最后,我们显示图像并等待用户按下任意键关闭窗口。
注意:上面的代码只是一个示例,实际使用时可能需要根据具体场景进行调整。
阅读全文
相关推荐

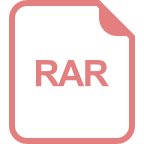
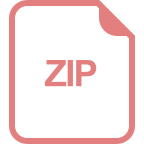
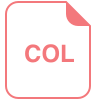
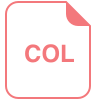
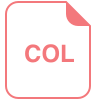
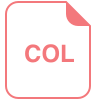
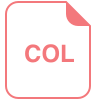
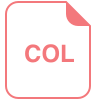
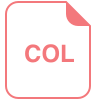
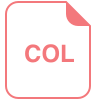
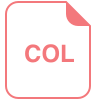
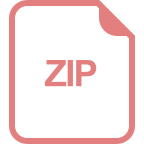
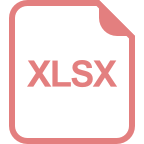