Verilog hdl编程实现盲人报时钟
时间: 2023-08-06 19:05:25 浏览: 110
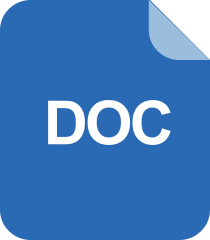
基于-VerilogHDL的闹钟设计.doc

好的,下面是一个基于Verilog HDL的盲人报时钟的代码实现。
首先,我们需要确定时钟模块的输入输出端口。时钟模块的输入端口需要包括一个时钟信号和一个复位信号,输出端口需要输出当前的时、分、秒。
```verilog
module clock(
input clk, // 时钟信号
input rst, // 复位信号
output reg [3:0] hour, // 时
output reg [5:0] minute, // 分
output reg [5:0] second // 秒
);
```
接下来,我们需要在时钟模块中定义一个计数器,用于记录当前的时、分、秒。每当计数器的值达到一定的阈值时,我们就可以更新时、分、秒的值。同时,我们需要实现一个报时功能,当时分秒满足特定条件时,时钟模块就会输出报时信号。
```verilog
module clock(
input clk, // 时钟信号
input rst, // 复位信号
output [3:0] hour, // 时
output [5:0] minute, // 分
output [5:0] second, // 秒
output reg beep // 报时信号
);
reg [3:0] cnt_hour;
reg [5:0] cnt_minute;
reg [5:0] cnt_second;
always @(posedge clk or posedge rst) begin
if (rst) begin
cnt_hour <= 0;
cnt_minute <= 0;
cnt_second <= 0;
beep <= 0;
end
else begin
cnt_second <= cnt_second + 1;
if (cnt_second == 60) begin
cnt_second <= 0;
cnt_minute <= cnt_minute + 1;
end
if (cnt_minute == 60) begin
cnt_minute <= 0;
cnt_hour <= cnt_hour + 1;
end
if (cnt_hour == 24) begin
cnt_hour <= 0;
end
end
end
always @(cnt_hour, cnt_minute, cnt_second) begin
beep <= 0;
if (cnt_second == 0 && cnt_minute == 0 && cnt_hour == 0) begin
beep <= 1; // 整点报时
end
else if (cnt_second == 0 && cnt_minute == 30) begin
beep <= 1; // 半点报时
end
end
assign hour = cnt_hour;
assign minute = cnt_minute;
assign second = cnt_second;
endmodule
```
在时钟模块中,我们使用了三个计数器来分别记录当前的时、分、秒。每当计数器达到一定阈值时,我们就会将其重置,并更新时、分、秒的值。同时,我们使用了两个if语句来实现报时功能。当时分秒满足特定条件时,我们就会将报时信号置为1。最后,我们将时、分、秒的值通过assign语句输出到对应的输出端口。
当时、分、秒满足报时条件时,我们可以通过一个蜂鸣器模块来实现报时的声音。蜂鸣器模块的输入端口需要包括一个时钟信号和一个报时信号,输出端口需要输出蜂鸣器的声音。蜂鸣器模块的代码如下所示。
```verilog
module buzzer(
input clk, // 时钟信号
input beep, // 报时信号
output reg out // 蜂鸣器输出
);
reg [23:0] cnt;
always @(posedge clk) begin
if (beep) begin
cnt <= cnt + 1;
if (cnt == 1000000) begin
out <= ~out;
cnt <= 0;
end
end
else begin
out <= 0;
end
end
endmodule
```
在蜂鸣器模块中,我们使用了一个计数器来控制蜂鸣器的输出。当报时信号为1时,计数器会不断累加,直到达到一个阈值时,我们就会将蜂鸣器的输出反转一次。这样就可以实现蜂鸣器的报时声音了。
最后,我们需要将时钟模块和蜂鸣器模块进行连接,以便实现完整的盲人报时钟。连接的代码如下所示。
```verilog
module clock_buzzer(
input clk, // 时钟信号
input rst, // 复位信号
output [3:0] hour, // 时
output [5:0] minute, // 分
output [5:0] second, // 秒
output reg out // 蜂鸣器输出
);
wire beep;
clock clock1(clk, rst, hour, minute, second, beep);
buzzer buzzer1(clk, beep, out);
endmodule
```
在连接模块中,我们首先定义了一个wire类型的beep信号,用于连接时钟模块和蜂鸣器模块。然后,我们将时钟模块和蜂鸣器模块进行连接,将beep信号作为输入端口进行连接。最终,我们就可以通过clk信号来控制整个盲人报时钟的运行了。
这就是一个基于Verilog HDL的盲人报时钟的代码实现。需要注意的是,具体的实现细节可能因为不同的硬件平台和编译工具而有所差异。如果您需要更详细的帮助,可以提供更具体的信息,我会尽力为您提供帮助。
阅读全文
相关推荐
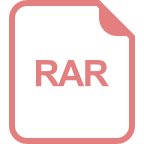
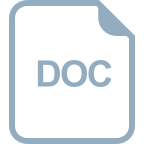
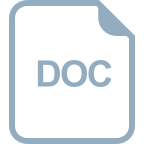
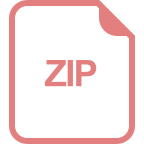
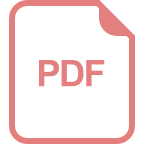
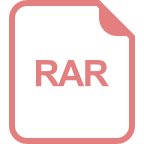
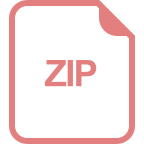
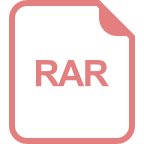
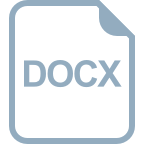
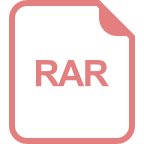
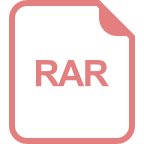
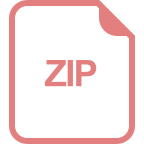