怎么在使用python读取word里面的内容,并使用flask,html在网页上显示,包括显示word里面的表格,公式,以及图片等,请给一个完整的代码
时间: 2024-02-09 12:09:29 浏览: 176
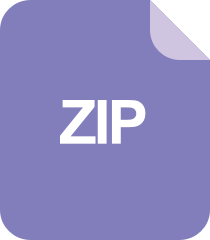
Python 使用Flask-SQLAlchemy创建数据表 Python源码
好的,以下是一个完整的代码示例,可以实现读取Word文档的内容,并使用Flask和HTML在网页上显示,包括表格、公式和图片等。请注意,需要将example.docx替换为实际的Word文档路径。
```python
import docx
from flask import Flask, render_template, url_for
app = Flask(__name__)
@app.route('/')
def index():
# 打开Word文档
doc = docx.Document('example.docx')
# 读取文本内容
paragraphs = [para.text for para in doc.paragraphs]
# 读取表格内容
table = []
for tbl in doc.tables:
for row in tbl.rows:
table.append([cell.text for cell in row.cells])
# 读取公式
formulas = []
for para in doc.paragraphs:
for run in para.runs:
if run._element.xml.startswith('<w:fldChar w:fldCharType="begin">'):
formulas.append(run.text)
# 读取图片
images = []
for i, image in enumerate(doc.inline_shapes):
image_name = f'image_{i}.png'
image_url = url_for('static', filename=image_name)
image.width, image.height = image.width // 6, image.height // 6 # 缩小图片
image._inline.graphic.graphicData.pic.nvPicPr.cNvPr.name = image_name # 修改图片名称
image._inline.graphic.graphicData.pic.blipFill.blip.embed = None # 删除原有的图片内容
image._inline.graphic.graphicData.pic.blipFill.blip = doc.part.add_image(image.blob, image.part.content_type) # 添加新的图片内容
image.blob = None # 删除图片内容
images.append({'filename': image_name, 'width': image.width, 'height': image.height, 'alt': image.alt})
return render_template('index.html', paragraphs=paragraphs, table=table, formulas=formulas, images=images)
if __name__ == '__main__':
app.run()
```
HTML模板代码如下:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Word内容展示</title>
</head>
<body>
<h1>Word文档内容</h1>
<div>
<p>文本内容:</p>
<ul>
{% for para in paragraphs %}
<li>{{ para }}</li>
{% endfor %}
</ul>
</div>
<div>
<p>表格内容:</p>
<table>
{% for row in table %}
<tr>
{% for cell in row %}
<td>{{ cell }}</td>
{% endfor %}
</tr>
{% endfor %}
</table>
</div>
<div>
<p>公式内容:</p>
<ul>
{% for formula in formulas %}
<li>{{ formula }}</li>
{% endfor %}
</ul>
</div>
<div>
<p>图片内容:</p>
{% for image in images %}
<img src="{{ url_for('static', filename=image.filename) }}" width="{{ image.width }}" height="{{ image.height }}" alt="{{ image.alt }}">
{% endfor %}
</div>
</body>
</html>
```
请注意,需要将index.html放置在templates文件夹下,将图片放置在static文件夹下。运行代码后,在浏览器中访问http://localhost:5000/,即可查看Word文档的内容。
阅读全文
相关推荐
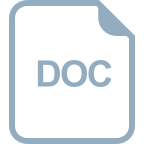
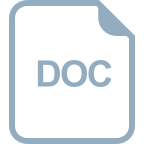




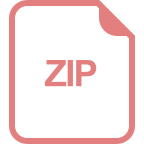
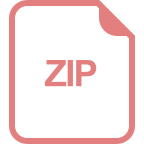

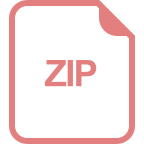
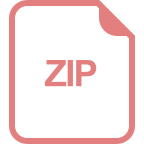
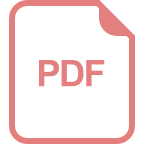
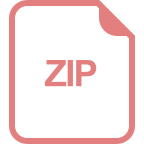
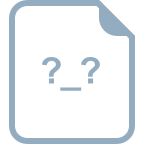
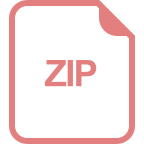
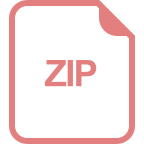
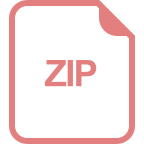