springboot怎么把execl表格展示在html中
时间: 2024-02-05 13:12:48 浏览: 28
要在HTML中展示Excel表格,需要先将Excel文件转换为HTML格式。可以使用第三方的Java库,如Apache POI或JExcelAPI,来读取Excel文件,然后将其转换为HTML格式。
以下是一个简单的示例代码,使用Apache POI将Excel文件转换为HTML格式:
```java
import java.io.FileInputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.util.CellRangeAddress;
import org.apache.poi.ss.util.CellReference;
public class ExcelToHtmlConverter {
public static String convertExcelToHtml(String filePath) throws IOException {
StringBuilder html = new StringBuilder();
FileInputStream input = new FileInputStream(filePath);
Workbook workbook = WorkbookFactory.create(input);
// Get the number of sheets in the workbook
int numberOfSheets = workbook.getNumberOfSheets();
// Loop through each sheet and convert it to HTML
for (int i = 0; i < numberOfSheets; i++) {
Sheet sheet = workbook.getSheetAt(i);
html.append("<table>");
// Loop through each row in the sheet
for (Row row : sheet) {
html.append("<tr>");
// Loop through each cell in the row
for (Cell cell : row) {
String cellValue = "";
// Check the cell type and format accordingly
switch (cell.getCellType()) {
case STRING:
cellValue = cell.getStringCellValue();
break;
case NUMERIC:
cellValue = String.valueOf(cell.getNumericCellValue());
break;
case BOOLEAN:
cellValue = String.valueOf(cell.getBooleanCellValue());
break;
case FORMULA:
// Evaluate the formula and get the cell value
cellValue = String.valueOf(cell.getNumericCellValue());
break;
}
html.append("<td>").append(cellValue).append("</td>");
}
html.append("</tr>");
}
html.append("</table>");
}
return html.toString();
}
}
```
然后,在Spring Boot应用程序中,可以使用Thymeleaf模板引擎将HTML代码渲染到页面上。在控制器中,可以调用以上的`convertExcelToHtml`方法来获取HTML代码,然后将其传递到模板中进行渲染。
以下是一个简单的示例控制器代码:
```java
import java.io.IOException;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
@Controller
public class ExcelController {
@GetMapping("/excel/{filename}")
public String viewExcel(Model model, @PathVariable("filename") String filename)
throws IOException {
String filePath = "/path/to/excel/" + filename + ".xlsx";
String html = ExcelToHtmlConverter.convertExcelToHtml(filePath);
model.addAttribute("html", html);
return "excel-view";
}
}
```
在上面的示例中,控制器的`viewExcel`方法根据文件名加载Excel文件,并将其转换为HTML格式。然后,将HTML代码添加到模型中,并将其传递到名为"excel-view"的Thymeleaf模板中进行渲染。在模板中,可以使用`th:utext`指令来渲染HTML代码,例如:
```html
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Excel View</title>
</head>
<body>
<h1>Excel View</h1>
<div th:utext="${html}"></div>
</body>
</html>
```
这将在页面上展示Excel表格的HTML代码。
相关推荐
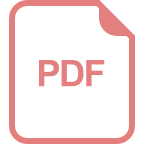
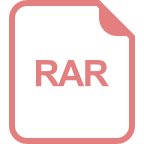
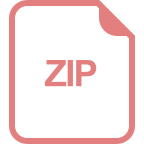














