C# MPU6050模块 代码算法
时间: 2023-11-18 07:05:47 浏览: 73
以下是一个使用 C# 和 MPU6050 模块的代码算法示例:
```csharp
using System;
using System.Threading;
namespace MPU6050
{
class Program
{
static void Main(string[] args)
{
// Initialize the MPU6050
MPU6050 mpu = new MPU6050();
mpu.Initialize();
// Continuously read and print the accelerometer and gyroscope values
while (true)
{
Vector3 acceleration = mpu.GetAcceleration();
Vector3 gyro = mpu.GetGyroscope();
Console.WriteLine("Acceleration: {0}, {1}, {2}", acceleration.x, acceleration.y, acceleration.z);
Console.WriteLine("Gyroscope: {0}, {1}, {2}", gyro.x, gyro.y, gyro.z);
Thread.Sleep(500);
}
}
}
public class MPU6050
{
private const int MPU_ADDRESS = 0x68;
private const int PWR_MGMT_1 = 0x6B;
private const int ACCEL_XOUT_H = 0x3B;
private const int GYRO_XOUT_H = 0x43;
private I2CDevice m_i2cDevice;
public void Initialize()
{
m_i2cDevice = I2CDevice.Create(new I2CConnectionSettings(1, MPU_ADDRESS));
// Wake up the MPU6050
WriteByte(PWR_MGMT_1, 0x00);
}
public Vector3 GetAcceleration()
{
byte[] buffer = ReadBytes(ACCEL_XOUT_H, 6);
short accelX = (short)((buffer[0] << 8) | buffer[1]);
short accelY = (short)((buffer[2] << 8) | buffer[3]);
short accelZ = (short)((buffer[4] << 8) | buffer[5]);
return new Vector3((float)accelX / 16384.0f, (float)accelY / 16384.0f, (float)accelZ / 16384.0f);
}
public Vector3 GetGyroscope()
{
byte[] buffer = ReadBytes(GYRO_XOUT_H, 6);
short gyroX = (short)((buffer[0] << 8) | buffer[1]);
short gyroY = (short)((buffer[2] << 8) | buffer[3]);
short gyroZ = (short)((buffer[4] << 8) | buffer[5]);
return new Vector3((float)gyroX / 131.0f, (float)gyroY / 131.0f, (float)gyroZ / 131.0f);
}
private void WriteByte(byte register, byte value)
{
m_i2cDevice.Write(new byte[] { register, value });
}
private byte[] ReadBytes(byte register, int count)
{
byte[] buffer = new byte[count];
m_i2cDevice.WriteRead(new byte[] { register }, buffer);
return buffer;
}
}
public struct Vector3
{
public float x;
public float y;
public float z;
public Vector3(float x, float y, float z)
{
this.x = x;
this.y = y;
this.z = z;
}
}
}
```
该示例使用了 C# 的 I2CDevice 类来与 MPU6050 进行通信,读取加速度和陀螺仪的值,并将其打印到控制台上。请注意,此示例中的 MPU6050 初始化使用了“0x68”作为地址,这可能需要根据您的硬件配置进行更改。
在此示例中,我们使用了一个名为“Vector3”的简单结构体,用于存储三维向量的值。这使得我们可以轻松地在代码中处理加速度和陀螺仪的值。
阅读全文
相关推荐



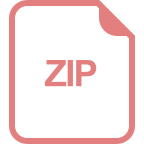
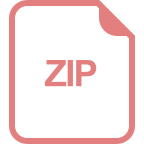
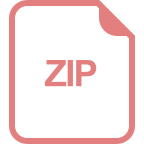
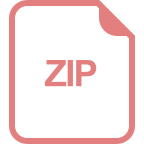
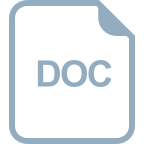
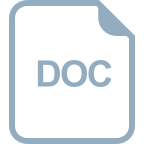
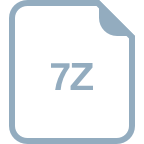