deeplabv3+添加SE教程
时间: 2023-09-22 16:07:01 浏览: 163
1. 安装SE模块
在deeplabv3代码中,SE模块已经被集成到了resnet.py文件中,因此我们只需要将此文件导入我们的项目即可使用。
2. 修改deeplabv3代码
在deeplabv3代码中,我们需要对resnet block的定义进行修改,以实现SE模块的添加。具体操作如下:
(1)打开resnet.py文件,找到resnet50和resnet101的定义部分。
(2)在resnet block的定义中加入SE模块,在每个block的最后添加一个SE block。SE block的定义如下:
```
class SEModule(nn.Module):
def __init__(self, channel, reduction=16):
super(SEModule, self).__init__()
self.avg_pool = nn.AdaptiveAvgPool2d(1)
self.fc = nn.Sequential(
nn.Linear(channel, channel // reduction, bias=False),
nn.ReLU(inplace=True),
nn.Linear(channel // reduction, channel, bias=False),
nn.Sigmoid()
)
def forward(self, x):
b, c, _, _ = x.size()
y = self.avg_pool(x).view(b, c)
y = self.fc(y).view(b, c, 1, 1)
return x * y
```
其中,channel为输入的通道数,reduction为SE模块中的缩放比例。
(3)将SE block添加到resnet block的最后。
```
class Bottleneck(nn.Module):
expansion = 4
def __init__(self, inplanes, planes, stride=1, downsample=None, reduction=16):
super(Bottleneck, self).__init__()
self.conv1 = nn.Conv2d(inplanes, planes, kernel_size=1, bias=False)
self.bn1 = nn.BatchNorm2d(planes)
self.conv2 = nn.Conv2d(planes, planes, kernel_size=3, stride=stride,
padding=1, bias=False)
self.bn2 = nn.BatchNorm2d(planes)
self.conv3 = nn.Conv2d(planes, planes * 4, kernel_size=1, bias=False)
self.bn3 = nn.BatchNorm2d(planes * 4)
self.relu = nn.ReLU(inplace=True)
self.downsample = downsample
self.stride = stride
self.se = SEModule(planes * 4, reduction=reduction) # 添加SE模块
def forward(self, x):
residual = x
out = self.conv1(x)
out = self.bn1(out)
out = self.relu(out)
out = self.conv2(out)
out = self.bn2(out)
out = self.relu(out)
out = self.conv3(out)
out = self.bn3(out)
if self.downsample is not None:
residual = self.downsample(x)
out += residual
out = self.relu(out)
out = self.se(out) # 添加SE模块
return out
```
(4)将修改后的resnet.py文件导入到我们的项目中。
3. 训练deeplabv3模型
在训练deeplabv3模型时,我们需要在训练代码中加入SE模块的计算。具体操作如下:
(1)在train.py文件中,找到训练循环的定义部分。
(2)在训练循环中加入SE模块的计算。
```
for i, (input, target) in enumerate(train_loader):
input = input.to(device)
target = target.to(device)
# 计算SE模块
output = model(input)
output = F.interpolate(output, size=target.size()[1:], mode='bilinear', align_corners=True)
loss = criterion(output, target)
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
其中,model为我们修改后的deeplabv3模型,criterion为我们选择的损失函数。
4. 测试deeplabv3模型
在测试deeplabv3模型时,我们同样需要在测试代码中加入SE模块的计算。具体操作如下:
(1)在test.py文件中,找到测试循环的定义部分。
(2)在测试循环中加入SE模块的计算。
```
for i, (input, target) in enumerate(test_loader):
input = input.to(device)
target = target.to(device)
# 计算SE模块
output = model(input)
output = F.interpolate(output, size=target.size()[1:], mode='bilinear', align_corners=True)
loss = criterion(output, target)
test_loss += loss.item()
pred = output.data.max(1)[1].cpu().numpy()
# 计算指标
test_acc += np.sum(pred == target.cpu().numpy())
test_total += target.size(0)
```
其中,model为我们修改后的deeplabv3模型,criterion为我们选择的损失函数。
5. 总结
通过以上步骤,我们就成功地将SE模块添加到了deeplabv3模型中。在训练和测试过程中,我们需要在计算输出时加入SE模块的计算,以提高模型的性能。
阅读全文
相关推荐
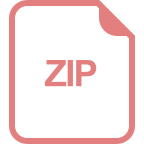
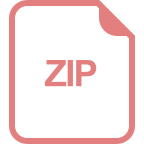
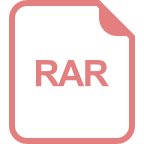

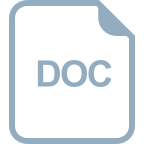













