jquery ajax json参数传递
时间: 2023-04-28 10:05:10 浏览: 180
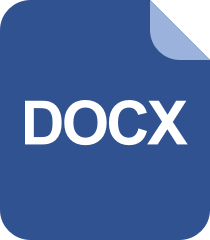
jsp中利用jquery+ajax在前后台之间传递json格式参数.docx
jQuery的Ajax方法支持使用JSON格式传递参数。在传递参数时,需要将参数转换为JSON字符串,并在Ajax设置中设置"contentType"为"application/json"。示例代码如下:
```
$.ajax({
type: "POST",
url: "some.php",
data: JSON.stringify({ name: "John", time: "2pm" }),
contentType: "application/json",
dataType: "json",
success: function(data){
alert(data);
}
});
```
这样可以在服务器端使用$_POST['name']和$_POST['time']来获取参数。
阅读全文
相关推荐
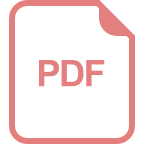
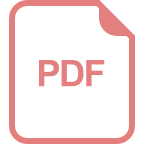
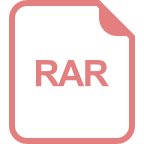
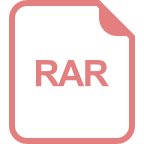
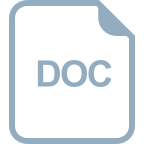

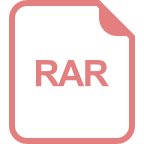
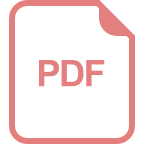
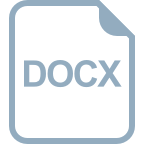
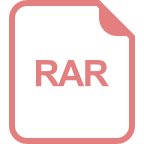
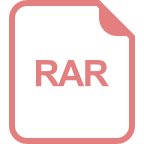
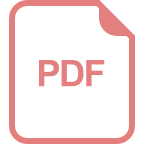
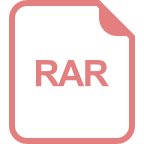
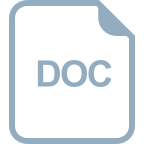
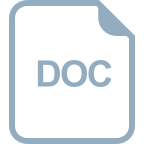
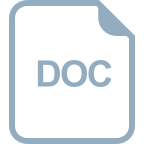
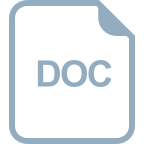