判断字符串是否为回文c++
时间: 2023-10-26 15:36:26 浏览: 113
#include <stdio.h>
#include <string.h>
int main()
{
char string[100];
int i, length, flag = 0;
printf("请输入一个字符串:");
scanf("%s", string);
length = strlen(string);
for(i=0;i < length ;i++){
if(string[i] != string[length-i-1]){
flag = 1;
break;
}
}
if (flag) {
printf("%s 不是回文字符串\n", string);
}
else {
printf("%s 是回文字符串\n", string);
}
return 0;
}
相关问题
判断字符串是否是回文C++
以下是C++判断字符串是否是回文的代码示例:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
int main() {
char str[101];
cin >> str;
int len = strlen(str);
bool isPalindrome = true;
for (int i = 0; i < len / 2; i++) {
if (str[i] != str[len - i - 1]) {
isPalindrome = false;
break;
}
}
if (isPalindrome) {
cout << "yes" << endl;
} else {
cout << "no" << endl;
}
return 0;
}
```
该代码首先读入一个字符串,然后使用一个布尔变量`isPalindrome`来记录该字符串是否是回文。接着使用一个循环遍历字符串的前一半字符,判断其是否与后一半字符对应位置的字符相同,如果不同则将`isPalindrome`设为`false`并跳出循环。最后根据`isPalindrome`的值输出结果。
判断一个字符串是否为回文C++
以下是C++实现判断字符串是否为回文的代码,其中使用了栈和过滤字符等技术:
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <algorithm>
using namespace std;
bool isPalindrome(string s) {
stack<char> st;
string filtered;
// 过滤掉非字母和数字的字符
remove_copy_if(s.begin(), s.end(), back_inserter(filtered), [](char c) {
return !isalnum(c);
});
// 将过滤后的字符压入栈中
for (char c : filtered) {
st.push(tolower(c));
}
// 依次弹出栈中的字符,与原字符串比较
for (char c : s) {
if (!isalnum(c)) {
continue;
}
if (tolower(c) != st.top()) {
return false;
}
st.pop();
}
return true;
}
int main() {
string s = "A man, a plan, a canal: Panama";
if (isPalindrome(s)) {
cout << "Yes" << endl;
} else {
cout << "No" << endl;
}
return 0;
}
```
阅读全文
相关推荐
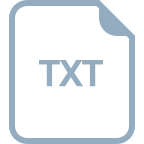













